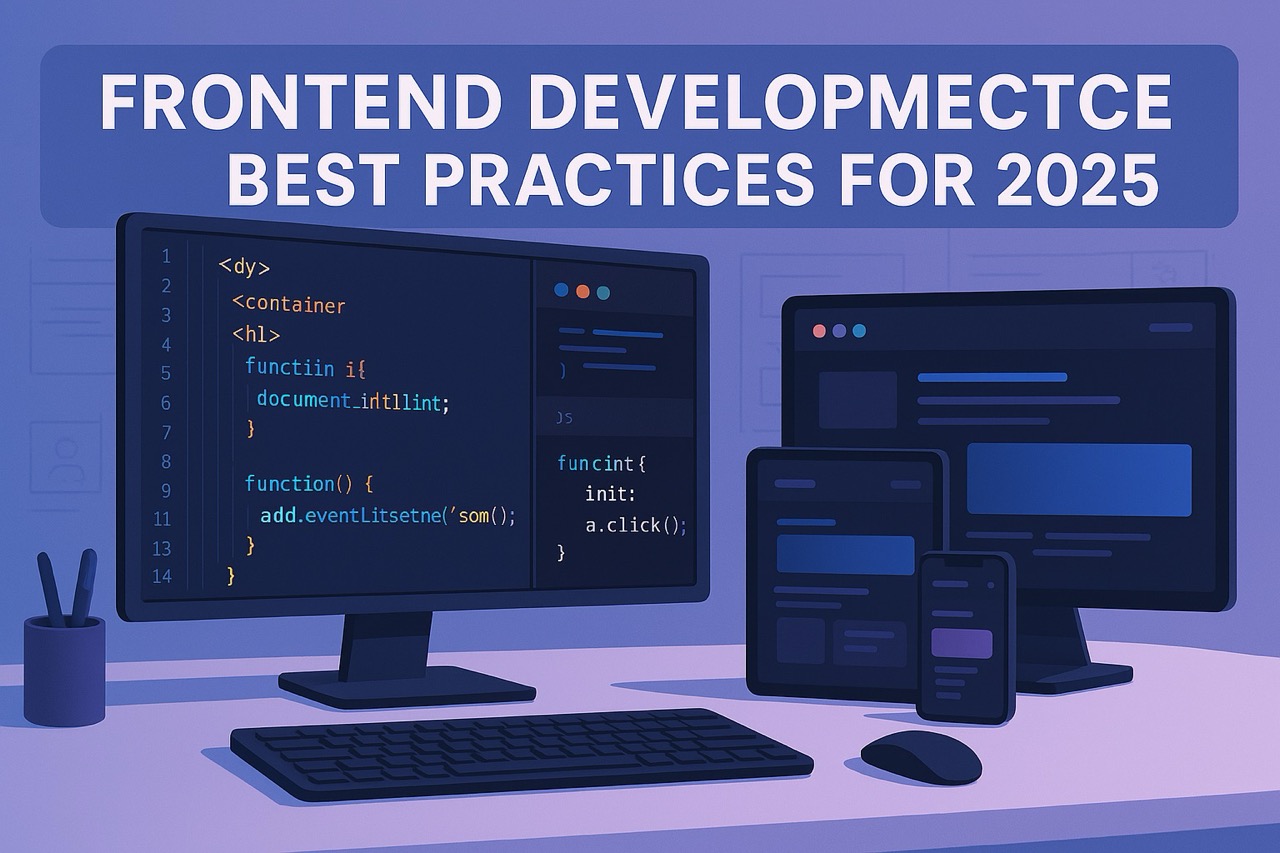
Introduction
The frontend development landscape continues to evolve at a remarkable pace. As we navigate through 2025, staying updated with modern frontend development best practices isn't just advantageous—it's essential for career growth and project success.
The frameworks and tools that dominated just a few years ago have undergone significant transformations. Build systems have become more sophisticated. User expectations for performance, accessibility, and experience have reached unprecedented levels. For mid-level developers, this constant evolution presents both challenges and opportunities to enhance your skills and create more impactful digital experiences.
This comprehensive guide to frontend development best practices for 2025 provides actionable insights into the current frontend ecosystem, optimization techniques, and emerging trends. Whether you're looking to refine your current workflow or completely revamp your technical toolkit, you'll find practical strategies to excel in today's competitive frontend development environment.
Current Frontend Development Ecosystem in 2025
Modern Frontend Frameworks and Libraries
The ecosystem has matured considerably, with different tools serving different needs:
- React remains a dominant force but has transformed significantly. React Server Components and the new React compiler have revolutionized how we build React applications, making them more efficient and performant. First-class TypeScript support is now standard in modern frontend development. For a deep dive into React performance optimization, check out our React Performance Optimization Guide for 2025.
// Modern React component using Server Components
// UserProfile.jsx
import { Suspense } from 'react'
import { fetchUserData } from '../api/user'
// This component runs on the server
async function UserData({ userId }) {
const userData = await fetchUserData(userId)
return <div className="user-data">{userData.name}</div>
}
// This is a Client Component
export default function UserProfile({ userId }) {
return (
<div className="profile-container">
<Suspense fallback={<p>Loading user data...</p>}>
<UserData userId={userId} />
</Suspense>
</div>
)
}
Vue.js has solidified its position with Vue 4.0, offering performance improvements and enhanced TypeScript integration.
Svelte and SvelteKit have gained significant market share, especially for projects where bundle size and runtime performance are critical concerns.
Astro has become the preferred solution for content-heavy websites, with its "islands architecture" now widely implemented as a frontend development best practice.
Qwik has established itself as a serious contender with its resumability approach to hydration.
Pro Tip: Framework selection should be guided by specific project requirements rather than popularity. For content-heavy sites with minimal interactivity, Astro might be optimal. For highly interactive applications, React, Vue, or Svelte continue to be strong choices for effective frontend development.
Frontend Development Build Tools
The build tool landscape has simplified considerably:
Vite has emerged as the standard build tool across most JavaScript frameworks, offering exceptional dev server performance and out-of-the-box optimizations.
Turbopack has matured and is now integrated into many Next.js projects.
Bun has evolved from a JavaScript runtime to include a comprehensive build toolchain that rivals Vite in performance for modern frontend development workflows.
# Setting up a modern frontend project with Bun in 2025
$ bun create react-app my-project
$ cd my-project
$ bun install
$ bun run dev
Gotcha: While newer build tools offer impressive performance, they sometimes lack plugin ecosystems as robust as more established tools. Always verify that the specific optimizations your project requires are supported before adopting a new frontend development build system.
CSS Methodologies in Frontend Development
CSS approaches have continued to evolve:
CSS-in-JS libraries have largely addressed performance concerns, with zero-runtime solutions like Vanilla Extract and Linaria gaining widespread adoption in modern frontend development.
Tailwind CSS remains popular and has evolved with more powerful customization options and improved tooling.
CSS Modules and Sass continue to be reliable choices for teams preferring a more traditional approach to styling in frontend development projects.
Container Queries and Cascade Layers are now widely used and supported, enhancing component-based design systems.
/* Modern CSS using cascade layers and container queries */
@layer base, components, utilities;
@layer components {
.card {
container-type: inline-size;
border-radius: 8px;
box-shadow: 0 2px 8px rgba(0, 0, 0, 0.1);
}
.card-content {
padding: 1rem;
}
@container (min-width: 400px) {
.card-content {
padding: 2rem;
display: grid;
grid-template-columns: 2fr 1fr;
}
/* Need help with CSS Grid layouts? Try our visual CSS Grid Generator: /tools/css-grid-generator */
}
}
Frontend Development Performance Optimization
Performance optimization has shifted from being a specialized concern to a core competency for all frontend developers. Here are the essential frontend development performance techniques for 2025:
Core Web Vitals Optimization for Frontend Development
Google's Core Web Vitals have expanded, and optimizing for these metrics is now a standard frontend development best practice:
- Largest Contentful Paint (LCP): Prioritize rendering the largest element in the viewport.
// Using priority hints for critical resources
<img src="hero.jpg" importance="high" fetchpriority="high" alt="Hero image" />
- Interaction to Next Paint (INP): Optimize all interactions, not just the first one.
// Debouncing inputs for better INP in frontend development
function debounce(func, delay) {
let timeout
return function () {
const context = this
const args = arguments
clearTimeout(timeout)
timeout = setTimeout(() => func.apply(context, args), delay)
}
}
const handleSearch = debounce((event) => {
// Search logic here
}, 150)
- Cumulative Layout Shift (CLS): Ensure elements don't move unexpectedly as the page loads.
Advanced Frontend Rendering Strategies
The conversation has shifted from client-side vs. server-side rendering to more nuanced approaches in modern frontend development:
- Partial Hydration: Only hydrate the interactive parts of a page.
- Progressive Hydration: Hydrate components gradually as they become visible.
- Streaming SSR: Start sending HTML to the browser before the entire page is ready.
- Islands Architecture: Independent components that hydrate in isolation.
// Example of progressive hydration with React for frontend development
import { lazy, Suspense } from 'react'
// Only loaded and hydrated when in viewport
const LazyComponent = lazy(() => import('./HeavyComponent'))
function App() {
return (
<div>
<Header />
<Main />
<Suspense fallback={<div>Loading...</div>}>
{/* This component will only be hydrated when visible */}
<IntersectionObserverWrapper>
<LazyComponent />
</IntersectionObserverWrapper>
</Suspense>
<Footer />
</div>
)
}
Pro Tip: Use the browser's Performance API to measure real user performance data rather than relying solely on lighthouse scores from development environments.
// Collecting and reporting real user performance metrics
if ('performance' in window && 'getEntriesByType' in performance) {
// Wait for load + 5 seconds to capture LCP
window.addEventListener('load', () => {
setTimeout(() => {
const navigationEntries = performance.getEntriesByType('navigation')[0]
const paintEntries = performance.getEntriesByType('paint')
const lcpEntry = performance.getEntriesByType('largest-contentful-paint')[0]
// Send to analytics
sendToAnalytics({
ttfb: navigationEntries.responseStart,
fcp: paintEntries.find((entry) => entry.name === 'first-contentful-paint')?.startTime,
lcp: lcpEntry?.startTime,
})
}, 5000)
})
}
Frontend Development Asset Optimization
Asset optimization remains crucial for modern frontend development:
Image Optimization: Modern formats like AVIF and WebP are now the standard, with automatic format selection based on browser support.
Font Loading Optimization: Optimizing font loading with the
size-adjust
descriptor and ensuring proper fallbacks are essential frontend development best practices.
<!-- Modern font loading approach for frontend performance -->
<link rel="preload" href="/fonts/MyFont.woff2" as="font" type="font/woff2" crossorigin />
<style>
@font-face {
font-family: 'MyFont';
src: url('/fonts/MyFont.woff2') format('woff2');
font-display: swap;
size-adjust: 105%;
ascent-override: 90%;
descent-override: 20%;
}
</style>
- Code Splitting: Breaking JavaScript bundles into smaller chunks loaded on demand is now easier with modern build tools and framework support.
Gotcha: Be careful with excessive code splitting - too many small chunks can lead to network congestion and actually harm performance by increasing the number of HTTP requests in your frontend development projects.
Frontend Development Accessibility Best Practices
Accessibility has transitioned from an afterthought to a fundamental requirement in frontend development, with legal implications in many jurisdictions:
WCAG 2.2 Compliance in Frontend Development
The Web Content Accessibility Guidelines (WCAG) 2.2 standards are now widely enforced:
- Focus Management: Ensuring all interactive elements are keyboard accessible and have visible focus states.
/* Accessible focus states for frontend development */
:focus-visible {
outline: 2px solid transparent;
outline-offset: 2px;
box-shadow: 0 0 0 2px currentColor;
}
- Semantic HTML: Using the correct HTML elements for their intended purpose remains the foundation of accessible websites and a core frontend development best practice. Learn more about semantic HTML in our guide to HTML5 essential tips and tricks.
<!-- Good semantic structure for frontend accessibility -->
<header>
<nav aria-label="Main navigation">
<ul>
<li><a href="/">Home</a></li>
<!-- More navigation items -->
</ul>
</nav>
</header>
<main>
<h1>Page title</h1>
<section aria-labelledby="section-heading">
<h2 id="section-heading">Section title</h2>
<!-- Content -->
</section>
</main>
<footer>
<!-- Footer content -->
</footer>
- ARIA Attributes: Using ARIA attributes appropriately when HTML semantics aren't sufficient is an important frontend development technique for enhanced accessibility.
Frontend Development Accessibility Testing
The tooling for accessibility testing has significantly improved:
- Automated Testing: Tools like Axe-core and Lighthouse have become more sophisticated in catching accessibility issues in frontend development.
// Integrating axe-core into your frontend testing workflow
import { axe } from 'jest-axe'
test('renders accessible content', async () => {
const { container } = render(<MyComponent />)
const results = await axe(container)
expect(results).toHaveNoViolations()
})
- Manual Testing: Screen reader testing has become a standard part of QA processes in modern frontend development, with better documentation and training available.
Pro Tip: Don't rely solely on automated accessibility tests. They typically only catch about 30-40% of issues. Regular manual testing with keyboard navigation and screen readers is essential for thorough frontend development accessibility compliance.
Responsive Design Strategies in Frontend Development
Responsive design has evolved beyond media queries to address the growing diversity of devices and user contexts:
Container Queries for Frontend Development
Container queries allow components to respond to their container's size rather than the viewport, revolutionizing modern frontend development:
.card-container {
container-type: inline-size;
container-name: card;
}
@container card (min-width: 400px) {
.card-title {
font-size: 1.5rem;
}
.card-content {
display: grid;
grid-template-columns: 2fr 1fr;
gap: 1rem;
}
}
Fluid Typography and Spacing in Frontend Development
Instead of discrete breakpoints, fluid values that scale smoothly across viewport sizes have become the norm in responsive frontend development:
:root {
--fluid-min-width: 320;
--fluid-max-width: 1200;
--fluid-screen: 100vw;
--fluid-bp: calc(
(var(--fluid-screen) - var(--fluid-min-width) / 16 * 1rem) /
(var(--fluid-max-width) - var(--fluid-min-width))
);
/* Fluid typography example */
--fs-300-min: 0.875;
--fs-300-max: 1;
--fs-300: calc(
(var(--fs-300-min) * 1rem) + (var(--fs-300-max) - var(--fs-300-min)) * var(--fluid-bp)
);
/* Fluid spacing example */
--space-md-min: 1;
--space-md-max: 2;
--space-md: calc(
(var(--space-md-min) * 1rem) + (var(--space-md-max) - var(--space-md-min)) * var(--fluid-bp)
);
}
body {
font-size: var(--fs-300);
padding: var(--space-md);
}
Advanced Media Features in Frontend Development
Media features beyond screen size have gained traction in modern frontend development:
/* Adapting UI based on user preference for reduced motion */
@media (prefers-reduced-motion: reduce) {
* {
animation-duration: 0.01ms !important;
animation-iteration-count: 1 !important;
transition-duration: 0.01ms !important;
scroll-behavior: auto !important;
}
}
/* Adapting UI based on user's color scheme preference */
@media (prefers-color-scheme: dark) {
:root {
--background: #121212;
--text: #ffffff;
}
}
Gotcha: Remember that not all devices support all media features, and degradation should be graceful. Default styles should be sensible even when media queries aren't supported in your frontend development projects.
State Management Solutions in Frontend Development
State management has become more streamlined and integrated into frameworks:
Framework-Native State Management in Frontend Development
- React's Built-in State Management: React's Context API combined with
useReducer
has become sufficient for many applications, reducing the need for external libraries in modern frontend development. Discover more about this approach in our article on using React Context API.
// Modern React state management approach for frontend development
import { createContext, useReducer, useContext } from 'react'
// Define initial state and reducer
const initialState = { count: 0 }
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 }
case 'decrement':
return { count: state.count - 1 }
default:
throw new Error()
}
}
// Create context
const CounterContext = createContext(null)
// Provider component
export function CounterProvider({ children }) {
const [state, dispatch] = useReducer(reducer, initialState)
return <CounterContext.Provider value={{ state, dispatch }}>{children}</CounterContext.Provider>
}
// Custom hook for using the context
export function useCounter() {
const context = useContext(CounterContext)
if (context === null) {
throw new Error('useCounter must be used within a CounterProvider')
}
return context
}
Vue's Pinia: Pinia has fully replaced Vuex as Vue's recommended state management solution for frontend development projects.
Svelte Stores: Svelte's built-in store mechanism aligns perfectly with its reactive programming model, providing efficient state management for modern frontend development.
External State Management Libraries for Frontend Development
For larger applications or specific needs, external libraries still have their place:
Redux Toolkit: Redux has been streamlined with Redux Toolkit, which is now the standard way to use Redux in frontend development.
Zustand: Offers a simpler API with hooks and less boilerplate than Redux, making it popular for medium-sized frontend development applications.
Jotai and Recoil: Atomic state management solutions have gained popularity for their granularity and flexibility in complex frontend development projects.
Pro Tip: Start with the simplest solution that meets your needs. Framework-native state management is often sufficient, and you can add more specialized tools as your application's requirements grow. This approach exemplifies frontend development best practices.
Frontend Development Testing Methodologies
Testing has shifted from being optional to being a core part of the frontend development process:
Component Testing in Frontend Development
Component testing has been simplified with modern tools:
// Modern component test using Vitest and Testing Library for frontend development
import { render, screen, fireEvent } from '@testing-library/react'
import { expect, test } from 'vitest'
import Counter from './Counter'
test('increments counter when button is clicked', () => {
render(<Counter initialCount={0} />)
const button = screen.getByRole('button', { name: /increment/i })
fireEvent.click(button)
expect(screen.getByText(/count: 1/i)).toBeInTheDocument()
})
For React-specific testing approaches, check out our comprehensive guide to unit testing React components.
End-to-End Testing for Frontend Development
E2E testing has become more reliable and easier to maintain:
- Playwright has gained significant adoption in frontend development due to its multi-browser support and powerful API.
// E2E test with Playwright for frontend applications
import { test, expect } from '@playwright/test'
test('user can log in', async ({ page }) => {
await page.goto('/login')
// Fill the login form
await page.fill('input[name="username"]', 'testuser')
await page.fill('input[name="password"]', 'password123')
// Click the login button
await page.click('button[type="submit"]')
// Verify successful login
await expect(page).toHaveURL('/dashboard')
await expect(page.locator('h1')).toContainText('Welcome, testuser')
})
- Cypress continues to be popular for frontend development testing with its developer-friendly API and visual debugging tools.
Frontend Development Testing Strategies
The testing pyramid has evolved with component testing taking a more central role:
- Unit Testing: Testing individual functions and utilities.
- Component Testing: Testing components in isolation, a key practice in modern frontend development.
- Integration Testing: Testing component interactions.
- E2E Testing: Testing complete user flows.
Gotcha: While test coverage is important in frontend development, it's easy to get caught in the trap of testing implementation details. Focus on testing behavior and user interactions rather than specific implementations, which can change as your code evolves.
Future Trends in Frontend Development
Looking ahead, several emerging technologies and approaches are likely to shape the future of frontend development:
WebAssembly (WASM) in Frontend Development
WebAssembly has begun to play a larger role in frontend applications:
- Performance-Critical Features: Moving computation-heavy tasks to WASM modules is becoming a valuable frontend development technique.
- Language Diversity: Bringing other programming languages (Rust, Go, C++) to the browser expands the possibilities for modern frontend development.
AI-Assisted Frontend Development
AI tools have become integral to the development workflow:
- Code Generation: AI assistants helping write boilerplate and common patterns.
- Debugging Assistance: AI tools helping identify and fix bugs more quickly in frontend development projects.
- Personalization: Using AI to dynamically personalize user experiences based on behavior is an emerging frontend development best practice.
Web Components and Micro-Frontends in Modern Frontend Development
Component-based architecture continues to evolve:
- Web Components: Greater adoption of native web components for cross-framework compatibility in frontend development.
- Micro-Frontends: Breaking down large applications into independently deployable frontend modules is transforming how teams approach modern frontend development.
// Creating a web component for modern frontend development
class UserCard extends HTMLElement {
constructor() {
super()
this.attachShadow({ mode: 'open' })
}
connectedCallback() {
const name = this.getAttribute('name')
const role = this.getAttribute('role')
this.shadowRoot.innerHTML = `
<style>
:host {
display: block;
border: 1px solid #ddd;
border-radius: 8px;
padding: 16px;
max-width: 300px;
}
h2 {
margin-top: 0;
}
</style>
<div>
<h2>${name}</h2>
<p>${role}</p>
<slot name="actions"></slot>
</div>
`
}
}
customElements.define('user-card', UserCard)
Edge Computing in Frontend Development
Moving logic closer to users with edge computing is becoming a key consideration in modern frontend development:
- Edge Functions: Running code at the edge for improved performance.
- Edge Databases: Storing and querying data closer to users enhances frontend development application responsiveness.
For a deeper look at how PWAs are changing the mobile landscape, see our article on Progressive Web Apps for Mobile Engagement in 2025.
Conclusion: Frontend Development Best Practices for 2025
Frontend development in 2025 is characterized by a maturation of tools and practices rather than revolutionary change. The focus has shifted toward performance, accessibility, and user experience over constantly adopting new frameworks.
As a mid-level developer looking to stay current with frontend development best practices, prioritize these key areas:
- Performance optimization techniques, particularly around Core Web Vitals
- Accessibility as a fundamental requirement rather than an add-on
- Modern CSS capabilities like container queries and cascade layers
- Testing methodologies that focus on user behavior
- Component-based architecture regardless of your framework choice
The most successful frontend developers in 2025 are those who master the fundamentals while strategically adopting new technologies that provide tangible benefits. By focusing on the user experience rather than technical novelty, you'll be well-positioned to create exceptional frontend development solutions that stand the test of time.
For a solid foundation in JavaScript, which remains essential for frontend development, explore our JavaScript 101: Essential Concepts for 2025 and Mastering JavaScript Best Practices guides.
Remember: the best frontend development techniques are those that solve real problems for your users, not simply the most trending topics in the development community.
Essential Frontend Development Tools
Enhance your frontend development workflow with these practical tools:
- CSS Grid Generator - Create responsive grid layouts visually
- Flexbox Generator - Build flexible layouts with ease
- Color Converter - Convert between HEX, RGB, and HSL color formats
- Meta Tag Generator - Generate SEO-optimized meta tags
- SERP Preview - Preview how your pages appear in search results
- Gradient Generator - Create beautiful CSS gradients visually
- Box Shadow Generator - Design CSS box shadows with live preview
- Color Contrast Checker - Ensure WCAG accessibility compliance
- Favicon Generator - Create favicons for all devices