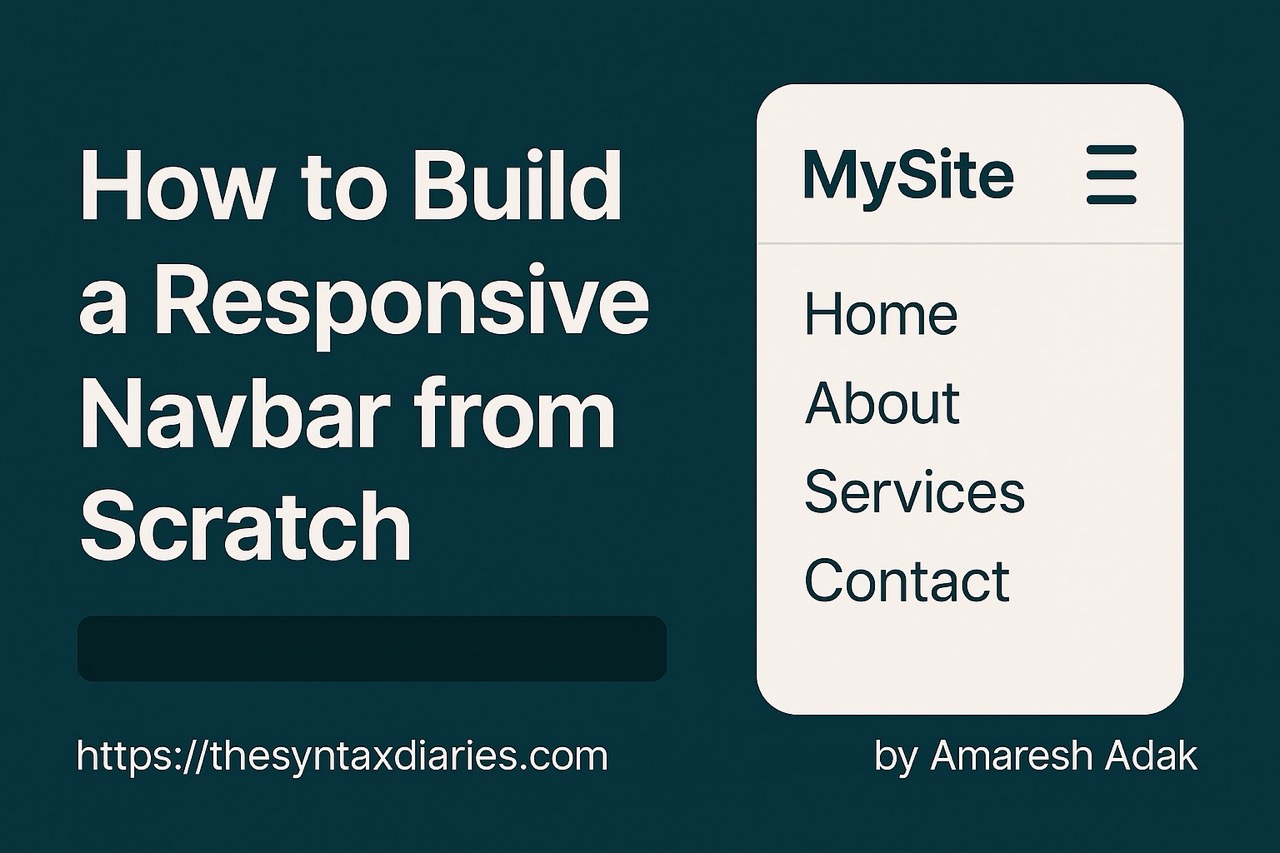
Every website needs a clean, mobile-friendly navbar — but building one from scratch can be tricky. In this beginner-friendly tutorial, you'll learn how to create a fully responsive navbar using just HTML, CSS, and JavaScript — no frameworks, no libraries, just the real stuff.
If you've ever struggled with hamburger menus or making your navigation look good on both desktop and mobile, this guide is for you. By the end, you'll have a professional-looking navbar that works perfectly across all devices.
What You'll Build
Before we dive in, let's take a look at what we're creating:
Demo Link - Check out the live version
Here's what our navbar includes:
- Clean, professional layout with logo and navigation links
- Smooth transitions between desktop and mobile views
- Hamburger menu that toggles on mobile with a satisfying animation
- No dependencies — pure HTML, CSS, and JavaScript
Project Setup
First, let's create our project structure. It's super simple:
project/
├── index.html
├── style.css
└── script.js
Now let's build this navbar step by step with detailed explanations of each component.
Step 1: HTML Structure - Breaking Down The Foundation
Let's start by creating the basic HTML structure for our navbar:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Responsive Navbar</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<header class="navbar">
<div class="logo">
<a href="#">MySite</a>
</div>
<nav class="nav-menu">
<ul class="nav-links">
<li><a href="#" class="active">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Services</a></li>
<li><a href="#">Projects</a></li>
<li><a href="#">Contact</a></li>
</ul>
</nav>
<div class="hamburger">
<span class="bar"></span>
<span class="bar"></span>
<span class="bar"></span>
</div>
</header>
<main>
<!-- Your page content goes here -->
<div class="content">
<h1>Welcome to MySite</h1>
<p>Resize the browser window to see the responsive navbar in action!</p>
</div>
</main>
<script src="script.js"></script>
</body>
</html>
Detailed Explanation of the HTML Structure
Let's break down each part of our HTML structure to understand its purpose:
Document Structure
- We start with the standard
<!DOCTYPE html>
declaration - We include
<html lang="en">
to specify the language for accessibility - In the
<head>
section, we include:- Character encoding with
<meta charset="UTF-8">
- The responsive viewport meta tag:
<meta name="viewport" content="width=device-width, initial-scale=1.0">
- this is crucial for mobile responsiveness - A link to our external CSS file
- Character encoding with
- We start with the standard
Navbar Container
- We use a semantic
<header>
element with class "navbar" as the main container - Using a semantic tag like
<header>
is better for SEO and accessibility than a generic<div>
- This element will hold all our navigation components
- We use a semantic
Logo Section
- A simple
<div>
with class "logo" containing an anchor link - We're using text for the logo, but you could easily replace this with an image
- The
<a>
tag makes the logo clickable, typically linking back to the homepage
- A simple
Navigation Menu
- We use the semantic
<nav>
element with class "nav-menu" to contain our navigation links - Inside, we have an unordered list
<ul>
with class "nav-links" - Each navigation item is a list item
<li>
containing an anchor<a>
tag - The "active" class on the Home link will help us style the current page differently
- Using a list structure is semantically correct for navigation items and helps with accessibility
- We use the semantic
Hamburger Menu
- A
<div>
with class "hamburger" will serve as our mobile menu toggle - It contains three
<span>
elements with class "bar" that will create the visual hamburger icon - We're building this with HTML/CSS rather than using an icon font or emoji for better control and performance
- It will be hidden on desktop views and only appear on mobile
- A
Page Content
- We include a simple
<main>
element with example content - This helps demonstrate how the navbar will look with actual page content below it
- We include a simple
JavaScript Link
- At the end of the body, we link to our JavaScript file
- Placing the script tag at the end of the body ensures that the HTML is fully loaded before any JavaScript runs
This structure follows best practices by:
- Using semantic HTML elements
- Creating a clean, logical hierarchy
- Being accessible to screen readers and other assistive technologies
- Separating structure (HTML), presentation (CSS), and behavior (JavaScript)
Step 2: Styling the Desktop View - Detailed CSS Explanation
Now let's add the CSS to make our navbar look good on desktop. We'll go through each section of the CSS in detail:
/* Reset some default browser styles */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
}
body {
background-color: #f5f5f5;
}
/* Basic content styling for demo */
.content {
max-width: 1200px;
margin: 100px auto 0;
padding: 0 20px;
text-align: center;
}
.content h1 {
margin-bottom: 20px;
color: #333;
}
/* Navbar Styles */
.navbar {
position: fixed;
top: 0;
left: 0;
width: 100%;
background-color: #2c3e50;
display: flex;
justify-content: space-between;
align-items: center;
padding: 0 2rem;
height: 70px;
box-shadow: 0 2px 10px rgba(0, 0, 0, 0.1);
z-index: 1000;
}
/* Logo Styles */
.logo a {
color: white;
font-size: 1.5rem;
font-weight: 700;
text-decoration: none;
transition: all 0.3s ease;
}
.logo a:hover {
color: #3498db;
}
/* Navigation Menu Styles */
.nav-links {
display: flex;
list-style: none;
}
.nav-links li {
margin-left: 2rem;
}
.nav-links a {
color: #ecf0f1;
text-decoration: none;
font-size: 1rem;
font-weight: 500;
padding: 0.5rem 0;
transition: all 0.3s ease;
position: relative;
}
.nav-links a:hover {
color: #3498db;
}
/* Active link indicator */
.nav-links a.active {
color: #3498db;
}
.nav-links a.active::after {
content: '';
position: absolute;
bottom: -3px;
left: 0;
width: 100%;
height: 2px;
background-color: #3498db;
}
/* Hamburger Menu */
.hamburger {
display: none;
cursor: pointer;
}
.bar {
display: block;
width: 25px;
height: 3px;
margin: 5px auto;
background-color: white;
transition: all 0.3s ease;
}
CSS Reset and Basic Styles
/* Reset some default browser styles */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
}
body {
background-color: #f5f5f5;
}
- CSS Reset: We start with a simple reset to remove browser default margins and padding
- Box-sizing: We set
box-sizing: border-box
on all elements to make sizing more predictable. This ensures padding and borders are included in an element's total width and height - Font Family: We define a system font stack using common sans-serif fonts
- Background Color: A light gray background provides contrast against the navbar
Content Styles
/* Basic content styling for demo */
.content {
max-width: 1200px;
margin: 100px auto 0;
padding: 0 20px;
text-align: center;
}
.content h1 {
margin-bottom: 20px;
color: #333;
}
- Max Width: Limits the content to 1200px for better readability on large screens
- Margin: Creates space above the content (100px) to account for the fixed navbar
- Padding: Adds horizontal spacing (20px) for better mobile presentation
- Text Alignment: Centers the text for this demo
Navbar Container Styles
/* Navbar Styles */
.navbar {
position: fixed;
top: 0;
left: 0;
width: 100%;
background-color: #2c3e50;
display: flex;
justify-content: space-between;
align-items: center;
padding: 0 2rem;
height: 70px;
box-shadow: 0 2px 10px rgba(0, 0, 0, 0.1);
z-index: 1000;
}
- Position: Fixed: This makes the navbar stay at the top of the viewport even when scrolling
- Width: Full width (100%) ensures the navbar spans the entire screen
- Background: Dark blue color (#2c3e50) for good contrast against white text
- Flexbox: We use
display: flex
withjustify-content: space-between
andalign-items: center
to:- Space out the logo and navigation items to opposite ends
- Vertically center all items within the navbar
- Padding: Horizontal padding (2rem) keeps content from touching the edges
- Height: Fixed height (70px) provides enough space without taking too much screen real estate
- Box Shadow: A subtle shadow adds depth and separates the navbar from content
- Z-index: Ensures the navbar appears above other elements (important for the mobile dropdown menu)
Logo Styles
/* Logo Styles */
.logo a {
color: white;
font-size: 1.5rem;
font-weight: 700;
text-decoration: none;
transition: all 0.3s ease;
}
.logo a:hover {
color: #3498db;
}
- Text Color: White for good contrast against the dark background
- Font Size: Larger (1.5rem) to make the logo prominent
- Font Weight: Bold (700) for emphasis
- Text Decoration: Removed underline from the anchor tag
- Transition: Smooth 0.3s transition for hover effects
- Hover Effect: Changes to blue (#3498db) on hover for interactive feedback
Navigation Links Styles
/* Navigation Menu Styles */
.nav-links {
display: flex;
list-style: none;
}
.nav-links li {
margin-left: 2rem;
}
.nav-links a {
color: #ecf0f1;
text-decoration: none;
font-size: 1rem;
font-weight: 500;
padding: 0.5rem 0;
transition: all 0.3s ease;
position: relative;
}
.nav-links a:hover {
color: #3498db;
}
- Flexbox for List:
display: flex
makes the navigation links display horizontally instead of vertically - List Style: We remove default bullet points with
list-style: none
- Spacing: Each list item gets left margin (2rem) to space them apart
- Link Styling:
- Light color (#ecf0f1) for good contrast
- No underline (
text-decoration: none
) - Moderate font size (1rem) and medium weight (500) for readability
- Vertical padding (0.5rem) increases clickable area
- Transition for smooth hover effects
- Relative positioning (important for the active indicator)
- Hover Effect: Changes to blue on hover for interactive feedback
Active Link Indicator
/* Active link indicator */
.nav-links a.active {
color: #3498db;
}
.nav-links a.active::after {
content: '';
position: absolute;
bottom: -3px;
left: 0;
width: 100%;
height: 2px;
background-color: #3498db;
}
- Active Text Color: Blue indicates the current page
- Underline Effect:
- Uses a pseudo-element (::after) to create an underline
- Position absolute with bottom: -3px places it below the text
- Full width (100%) spans the entire link
- 2px height creates a visible but not overwhelming line
- Same blue color as the text for consistency
Hamburger Menu (Hidden on Desktop)
/* Hamburger Menu */
.hamburger {
display: none;
cursor: pointer;
}
.bar {
display: block;
width: 25px;
height: 3px;
margin: 5px auto;
background-color: white;
transition: all 0.3s ease;
}
- Display None: Hides the hamburger menu on desktop views
- Cursor: Changes to pointer on hover to indicate it's clickable
- Hamburger Bars:
- Each bar is a span displayed as a block element
- 25px width and 3px height creates the line appearance
- 5px margin between bars spaces them out
- White color for visibility against the dark background
- Transition for animation effects (to be used in mobile view)
Step 3: Adding Mobile Responsiveness - Detailed Breakdown
Now we'll add the CSS that transforms our navbar for smaller screens:
/* Mobile Responsive Styles */
@media only screen and (max-width: 768px) {
/* Show hamburger menu on mobile */
.hamburger {
display: block;
}
/* Animate hamburger to X when menu is active */
.hamburger.active .bar:nth-child(1) {
transform: translateY(8px) rotate(45deg);
}
.hamburger.active .bar:nth-child(2) {
opacity: 0;
}
.hamburger.active .bar:nth-child(3) {
transform: translateY(-8px) rotate(-45deg);
}
/* Position the nav menu for mobile */
.nav-menu {
position: fixed;
top: 70px; /* Height of the navbar */
left: -100%;
width: 100%;
background-color: #2c3e50;
text-align: center;
transition: 0.3s;
box-shadow: 0 10px 10px rgba(0, 0, 0, 0.1);
}
/* Show nav menu when active class is added */
.nav-menu.active {
left: 0;
}
/* Stack nav links vertically */
.nav-links {
flex-direction: column;
padding: 20px 0;
}
.nav-links li {
margin: 10px 0;
}
}
Media Query Explanation
@media only screen and (max-width: 768px) {
/* Mobile styles here */
}
- Media Query: This targets screens with a maximum width of 768px (tablets and phones)
- Breakpoint Choice: 768px is a common breakpoint for tablets and smaller devices
- We're using "only screen" to target just screen displays, not print or other media types
Hamburger Menu Display
/* Show hamburger menu on mobile */
.hamburger {
display: block;
}
- Display Block: Changes from
display: none
on desktop todisplay: block
on mobile - This makes the hamburger icon visible only on smaller screens
Hamburger Animation
/* Animate hamburger to X when menu is active */
.hamburger.active .bar:nth-child(1) {
transform: translateY(8px) rotate(45deg);
}
.hamburger.active .bar:nth-child(2) {
opacity: 0;
}
.hamburger.active .bar:nth-child(3) {
transform: translateY(-8px) rotate(-45deg);
}
- Animation Logic: When the
.active
class is added to the hamburger (via JavaScript), the bars transform into an "X" shape - First Bar: Moves down 8px and rotates 45 degrees
- Second Bar: Fades out completely
- Third Bar: Moves up 8px and rotates -45 degrees
- This creates a satisfying transformation from hamburger to X, providing visual feedback when the menu is open
Mobile Navigation Menu Positioning
/* Position the nav menu for mobile */
.nav-menu {
position: fixed;
top: 70px; /* Height of the navbar */
left: -100%;
width: 100%;
background-color: #2c3e50;
text-align: center;
transition: 0.3s;
box-shadow: 0 10px 10px rgba(0, 0, 0, 0.1);
}
/* Show nav menu when active class is added */
.nav-menu.active {
left: 0;
}
- Fixed Positioning: Keeps the menu in place when scrolling
- Off-Screen Placement: Initially positioned off-screen to the left (
left: -100%
) - Top Position: Placed directly below the navbar (70px from top)
- Full Width: Takes up the entire width of the screen
- Background Color: Matches the navbar for visual consistency
- Text Alignment: Centers the menu items
- Transition: Smooth 0.3s animation for the sliding effect
- Box Shadow: Adds depth when the menu is open
- Active State: When
.active
class is added, the menu slides in from the left (left: 0
)
Vertical Navigation Links
/* Stack nav links vertically */
.nav-links {
flex-direction: column;
padding: 20px 0;
}
.nav-links li {
margin: 10px 0;
}
- Flexbox Direction: Changes from horizontal (
row
) to vertical (column
) layout - Padding: Adds vertical space (20px) at top and bottom
- List Item Margins: Changes from horizontal margins to vertical margins (10px)
- This creates a clean, stacked appearance for the mobile menu links
Step 4: JavaScript Functionality - In-Depth Explanation
Finally, we'll add the JavaScript that makes our navbar interactive:
// Select elements we'll need to manipulate
const hamburger = document.querySelector('.hamburger')
const navMenu = document.querySelector('.nav-menu')
const navLinks = document.querySelectorAll('.nav-links a')
// Toggle mobile menu when hamburger is clicked
hamburger.addEventListener('click', () => {
// Toggle active class on hamburger and nav menu
hamburger.classList.toggle('active')
navMenu.classList.toggle('active')
// Add accessibility attributes
const isExpanded = hamburger.classList.contains('active')
hamburger.setAttribute('aria-expanded', isExpanded)
})
// Close mobile menu when a nav link is clicked
navLinks.forEach((link) => {
link.addEventListener('click', () => {
// Only take action if hamburger menu is visible (mobile view)
if (window.innerWidth <= 768) {
hamburger.classList.remove('active')
navMenu.classList.remove('active')
hamburger.setAttribute('aria-expanded', false)
}
})
})
// Add accessibility support
function addAccessibility() {
// Add ARIA attributes to hamburger menu
hamburger.setAttribute('aria-label', 'Toggle navigation menu')
hamburger.setAttribute('aria-expanded', false)
hamburger.setAttribute('role', 'button')
hamburger.setAttribute('tabindex', '0')
// Allow keyboard activation of hamburger menu
hamburger.addEventListener('keydown', (e) => {
if (e.key === 'Enter' || e.key === ' ') {
e.preventDefault()
hamburger.click()
}
})
}
// Initialize accessibility features
addAccessibility()
DOM Element Selection
// Select elements we'll need to manipulate
const hamburger = document.querySelector('.hamburger')
const navMenu = document.querySelector('.nav-menu')
const navLinks = document.querySelectorAll('.nav-links a')
- document.querySelector(): Selects the first element matching the CSS selector
- document.querySelectorAll(): Selects all elements matching the selector, returning a NodeList
- We're grabbing references to:
- The hamburger menu toggle button
- The navigation menu container
- All navigation links (as a collection)
Hamburger Menu Toggle
// Toggle mobile menu when hamburger is clicked
hamburger.addEventListener('click', () => {
// Toggle active class on hamburger and nav menu
hamburger.classList.toggle('active')
navMenu.classList.toggle('active')
// Add accessibility attributes
const isExpanded = hamburger.classList.contains('active')
hamburger.setAttribute('aria-expanded', isExpanded)
})
- Event Listener: Adds a 'click' event handler to the hamburger icon
- classList.toggle(): Adds the 'active' class if it's not present, or removes it if it is
- This toggles both the hamburger animation and the mobile menu visibility
- Accessibility: Updates the 'aria-expanded' attribute to match the current state
- This helps screen readers announce whether the menu is expanded or collapsed
Close Menu on Link Click
// Close mobile menu when a nav link is clicked
navLinks.forEach((link) => {
link.addEventListener('click', () => {
// Only take action if hamburger menu is visible (mobile view)
if (window.innerWidth <= 768) {
hamburger.classList.remove('active')
navMenu.classList.remove('active')
hamburger.setAttribute('aria-expanded', false)
}
})
})
- forEach Loop: Iterates through all navigation links to add the same event listener to each
- Click Handler: When any navigation link is clicked:
- Checks if we're in mobile view
(window width <= 768px)
- If so, removes the 'active' class from both the hamburger and nav menu
- Updates the 'aria-expanded' attribute to false
- Checks if we're in mobile view
- UX Improvement: This automatically closes the mobile menu after a link is clicked
- Prevents users from having to manually close the menu after navigation
- Creates a smoother user experience by returning to a clean view of the new page content
Accessibility Enhancements
// Add accessibility support
function addAccessibility() {
// Add ARIA attributes to hamburger menu
hamburger.setAttribute('aria-label', 'Toggle navigation menu')
hamburger.setAttribute('aria-expanded', false)
hamburger.setAttribute('role', 'button')
hamburger.setAttribute('tabindex', '0')
// Allow keyboard activation of hamburger menu
hamburger.addEventListener('keydown', (e) => {
if (e.key === 'Enter' || e.key === ' ') {
e.preventDefault()
hamburger.click()
}
})
}
// Initialize accessibility features
addAccessibility()
- Encapsulation: Groups all accessibility-related code into a single function
- ARIA Attributes: Adds several important attributes for screen readers:
aria-label
: Provides a text description of the element's purposearia-expanded
: Indicates whether the controlled element is expanded or collapsedrole="button"
: Explicitly defines the element as a button for assistive technologiestabindex="0"
: Makes the element focusable with keyboard navigation
- Keyboard Activation: Adds keyboard support for the hamburger menu
- Listens for Enter or Space key presses (standard button activation keys)
- Prevents default behavior (like page scrolling when Space is pressed)
- Programmatically clicks the hamburger menu
- Function Call: Immediately invokes the function when the script loads
This JavaScript implementation focuses on three key areas:
- Functionality: Making the mobile toggle work correctly
- User Experience: Ensuring intuitive behavior when navigating
- Accessibility: Supporting all users, including those with disabilities
Full Implementation: How Everything Works Together
Now that we've built all the individual components, let's understand how they work together to create a seamless responsive navbar:
Desktop Experience (>768px)
On desktop screens:
- The navbar displays horizontally across the top of the page
- The logo appears on the left
- Navigation links display horizontally on the right
- The hamburger menu is hidden
- Hover effects provide visual feedback
- The current page is indicated with a blue color and underline
Mobile Experience (≤768px)
When the screen size reduces to 768px or smaller:
- The navbar remains at the top, but the navigation links disappear
- The hamburger icon appears on the right
- Clicking the hamburger icon:
- Animates the icon into an X shape
- Slides in the navigation menu from the left
- Updates accessibility attributes
- The navigation menu displays links in a stacked vertical layout
- Clicking any navigation link:
- Takes the user to the selected page
- Automatically closes the mobile menu
- Resets the hamburger icon back to its original state
Responsive Transition
The transition between desktop and mobile views happens automatically based on the screen width:
- When resizing below 768px:
- Navigation links disappear
- Hamburger menu appears
- Menu layout changes from horizontal to vertical (though hidden initially)
- When resizing above 768px:
- Hamburger menu disappears
- Navigation links reappear horizontally
- Any open mobile menu is effectively reset
Technical Implementation Details
Several key techniques make this responsive behavior possible:
- CSS Media Queries: Detect screen size and apply different styles
- Flexbox: Provides flexible layouts that adapt to different screen sizes
- CSS Transitions: Create smooth animations between states
- JavaScript DOM Manipulation: Dynamically changes classes to toggle visibility
- Event Listeners: Respond to user interactions (clicks and key presses)
- ARIA Attributes: Support accessibility for all users
Customization Options and Advanced Techniques
Now that you understand how to build a basic responsive navbar, let's explore some ways to enhance it further:
Adding Dropdown Menus
For sites with more complex navigation structures, dropdown menus can be a useful addition:
<!-- Add this to your HTML nav-links section -->
<li class="dropdown">
<a href="#" class="dropdown-toggle">Services <span class="dropdown-arrow">▼</span></a>
<ul class="dropdown-menu">
<li><a href="#">Web Design</a></li>
<li><a href="#">Development</a></li>
<li><a href="#">SEO</a></li>
</ul>
</li>
/* Dropdown styles */
.dropdown {
position: relative;
}
.dropdown-toggle {
display: flex;
align-items: center;
}
.dropdown-arrow {
font-size: 0.7rem;
margin-left: 5px;
transition: transform 0.3s ease;
}
.dropdown-menu {
position: absolute;
top: 100%;
left: 0;
background-color: #34495e;
width: 200px;
padding: 10px 0;
border-radius: 4px;
box-shadow: 0 5px 15px rgba(0, 0, 0, 0.1);
opacity: 0;
visibility: hidden;
transform: translateY(10px);
transition: all 0.3s ease;
z-index: 10;
list-style: none;
}
.dropdown:hover .dropdown-menu {
opacity: 1;
visibility: visible;
transform: translateY(0);
}
.dropdown:hover .dropdown-arrow {
transform: rotate(180deg);
}
.dropdown-menu li {
margin: 0;
}
.dropdown-menu a {
display: block;
padding: 8px 20px;
transition: background-color 0.3s ease;
}
.dropdown-menu a:hover {
background-color: #2c3e50;
}
/* Mobile adjustments for dropdowns */
@media only screen and (max-width: 768px) {
.dropdown-menu {
position: static;
width: 100%;
background-color: #34495e;
opacity: 1;
visibility: hidden;
max-height: 0;
overflow: hidden;
transform: none;
box-shadow: none;
transition: all 0.3s ease;
}
.dropdown.active .dropdown-menu {
visibility: visible;
max-height: 500px;
}
}
// Add this inside your existing JavaScript
const dropdowns = document.querySelectorAll('.dropdown')
dropdowns.forEach((dropdown) => {
const toggle = dropdown.querySelector('.dropdown-toggle')
toggle.addEventListener('click', (e) => {
// Only handle clicks for mobile view
if (window.innerWidth <= 768) {
e.preventDefault()
dropdown.classList.toggle('active')
}
})
})
This implementation:
- Uses absolute positioning for desktop dropdown menus
- Animates the dropdown arrow rotation
- Adapts the behavior for mobile (stacked, accordion-style dropdowns)
- Prevents default link behavior when toggling on mobile
Adding a Scroll Effect
A popular enhancement is to change the navbar's appearance when scrolling:
// Add this to your JavaScript file
window.addEventListener('scroll', () => {
const navbar = document.querySelector('.navbar')
// Add 'scrolled' class when page is scrolled down
if (window.scrollY > 50) {
navbar.classList.add('scrolled')
} else {
navbar.classList.remove('scrolled')
}
})
.navbar {
/* ... existing styles ... */
transition:
background-color 0.3s ease,
height 0.3s ease;
}
.navbar.scrolled {
background-color: rgba(44, 62, 80, 0.9); /* Semi-transparent background */
height: 60px; /* Smaller height when scrolled */
backdrop-filter: blur(5px); /* Modern blur effect */
}
/* Adjust logo size when scrolled */
.navbar.scrolled .logo a {
font-size: 1.3rem;
}
This creates:
- A subtle height reduction when scrolling
- A semi-transparent background with blur effect
- A smaller logo for the compact scrolled state
- Smooth transitions between normal and scrolled states
Enhanced Accessibility
To make your navbar even more accessible:
<!-- Add this before your main content -->
<a href="#main-content" class="skip-link">Skip to main content</a>
<!-- Add an ID to your main content -->
<main id="main-content">
<!-- Content here -->
</main>
/* Skip link for keyboard users */
.skip-link {
position: absolute;
top: -40px;
left: 0;
background-color: #3498db;
color: white;
padding: 8px;
z-index: 2000;
transition: top 0.3s ease;
}
.skip-link:focus {
top: 0;
}
This adds:
- A "skip to content" link that appears only when focused (for keyboard users)
- Allows users to bypass the navigation and jump directly to the main content
- An important accessibility feature recommended by WCAG guidelines
Mobile-First Approach
While our example started with desktop styles, a better practice is to use a mobile-first approach:
/* Mobile styles (base styles) */
.navbar {
/* Mobile styles here */
}
/* Desktop styles (applied only at larger screens) */
@media only screen and (min-width: 769px) {
.navbar {
/* Desktop styles here */
}
}
Benefits of mobile-first:
- Prioritizes the mobile experience (where most users are)
- Can improve performance on mobile devices
- Encourages simpler designs that scale up rather than complex designs that need to be simplified
Common Challenges and Solutions
Challenge 1: Navbar Covering Content
When using position: fixed
, the navbar takes up space in the viewport but not in the document flow.
Solution:
body {
padding-top: 70px; /* Same as navbar height */
}
Or preferably, use a more flexible approach:
:root {
--navbar-height: 70px;
}
.navbar {
height: var(--navbar-height);
}
body {
padding-top: var(--navbar-height);
}
Challenge 2: Handling Very Long Navigation Menus
For sites with many navigation items:
Solution for Desktop:
@media only screen and (min-width: 769px) {
.nav-links {
flex-wrap: wrap;
justify-content: flex-end;
}
}
Solution for Mobile:
@media only screen and (max-width: 768px) {
.nav-menu {
max-height: 80vh;
overflow-y: auto;
}
}
Challenge 3: Supporting Older Browsers
Some browsers might not support flexbox or CSS variables.
Solution:
/* Flexbox fallback using floats */
.navbar {
overflow: hidden; /* Clear floats */
}
.logo {
float: left;
}
.nav-menu {
float: right;
}
/* Then use your flexbox styles with @supports */
@supports (display: flex) {
.navbar {
display: flex;
overflow: visible;
}
.logo,
.nav-menu {
float: none;
}
}
Best Practices to Remember
- Semantic HTML: Use proper semantic elements (
<header>
,<nav>
,<ul>
, etc.) - Mobile-First: Start with mobile styles, then enhance for larger screens
- Accessibility: Include ARIA attributes, keyboard navigation, and focus styles
- Progressive Enhancement: Ensure basic functionality works without JavaScript
- Performance: Minimize unnecessary animations and transitions
- Testing: Test on multiple devices and browsers
- CSS Variables: Use them for consistent values (colors, sizes, etc.)
- Comments: Document your code for easier maintenance
- Minimum Touch Target: Ensure mobile tap targets are at least 44x44 pixels
- Contrast: Maintain sufficient contrast between text and background colors
Conclusion
Building a responsive navbar from scratch gives you complete control over its appearance, behavior, and performance. While frameworks like Bootstrap offer ready-made navbars, understanding how to build one yourself provides valuable insights into responsive design principles.
The approach we've covered uses modern HTML, CSS, and JavaScript techniques to create a navbar that:
- Works seamlessly across all device sizes
- Provides smooth transitions between states
- Supports accessibility requirements
- Offers a solid foundation for customization
By mastering this fundamental component, you'll be better equipped to create responsive websites that provide excellent user experiences regardless of the device being used.
Let's Connect!
💬 Got stuck or want the full source code? Drop a comment below or check out the GitHub repo here.
🔔 Follow me for more beginner-friendly frontend tutorials every week. Next time we'll tackle how to build a responsive image gallery with lightbox functionality!
Happy coding! 🚀