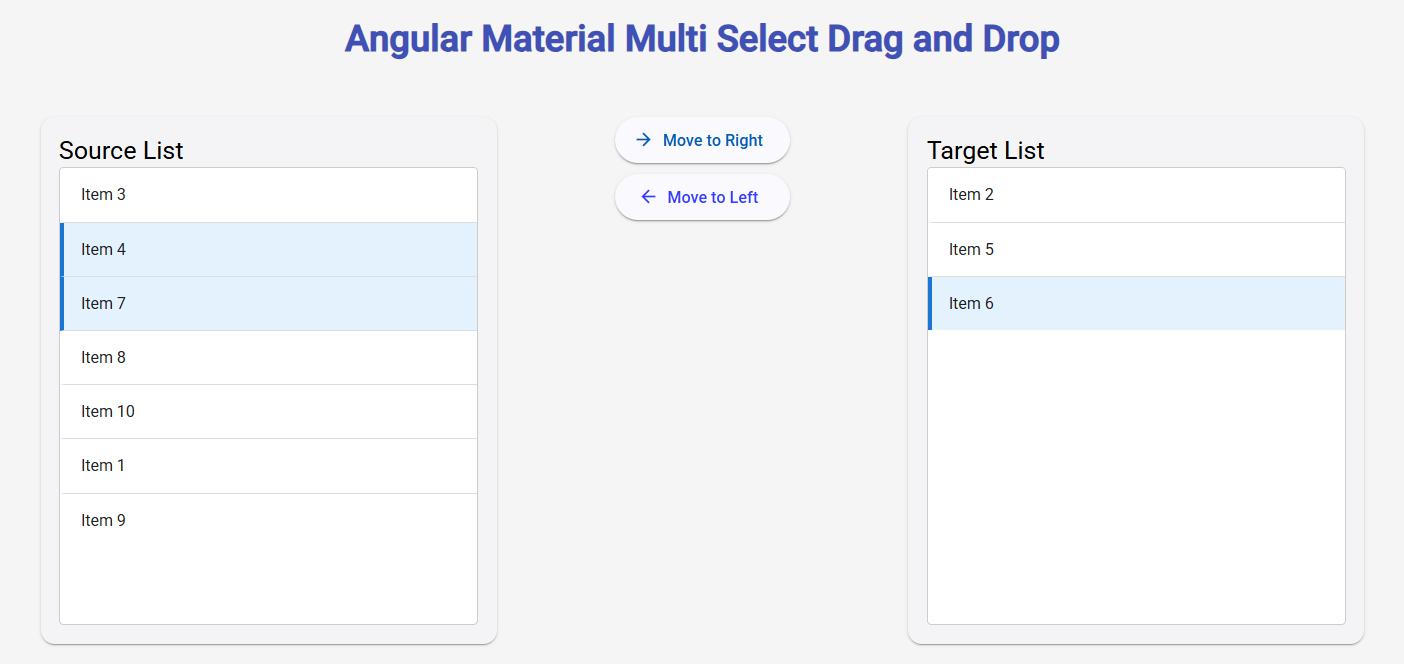
What is Multi-Select Drag and Drop in Angular?
Multi-select drag and drop is a UI pattern that allows users to select multiple items at once and move them between lists using either drag gestures or button controls. Unlike standard drag and drop that only moves one item at a time, multi-select provides a more efficient way to organize large datasets, making it perfect for task management, content organization, and data categorization interfaces.
Why standard drag and drop isn't enough: Angular Material's CDK provides basic drag and drop functionality, but doesn't natively support moving multiple selected items simultaneously. Our implementation solves this common limitation.
What We're Building
By the end of this tutorial, you'll create an Angular component that allows users to:
- Select multiple items across two lists
- Drag any selected item to move the entire selection
- Use buttons as an alternative to drag gestures
- See clear visual feedback for selected items
GitHub Repository: Complete Source Code Available Here
Common Angular Drag and Drop Errors (And How To Fix Them)
Before we dive into the implementation, let's address some frequent issues developers encounter:
Error | Common Cause | Solution |
---|---|---|
"Cannot read properties of undefined (reading 'data')" | Incorrect list references | Ensure your template correctly references list variables with #leftList and #rightList |
Items won't drag between lists | Missing connection | Verify [cdkDropListConnectedTo] arrays include all target lists |
Multi-selected items not moving together | Selection state not tracked | Implement separate arrays to track selected items |
Selection not visually indicated | Missing CSS | Add .selected class styling with prominent visual indicators |
Performance issues with large lists | DOM overload | Implement virtual scrolling for lists with >100 items |
Starting with Project Setup
First things first, let's set up our environment:
# Create a new Angular project
ng new angular-material-drag-drop-poc --routing=true --style=scss
# Navigate to project directory
cd angular-material-drag-drop-poc
# Add Angular Material
ng add @angular/material
# Install Angular CDK if not already included
npm install @angular/cdk
The Angular CDK (Component Dev Kit) provides the core drag-and-drop functionality we'll be using, while Angular Material gives us nice-looking UI components.
Understanding Our Data Structure
We need a simple interface to represent the items in our lists:
// Item model
export interface Item {
id: number
name: string
// Add more properties as needed for your use case
}
Each item has a unique ID and a name. In a real application, you might add more properties depending on what you're displaying.
Component Structure: Managing Two Lists
Our component needs to track two things:
- The items in each list
- Which items are currently selected in each list
Here's how we set up the component class:
import { Component, OnInit } from '@angular/core'
import { CdkDragDrop, moveItemInArray, transferArrayItem } from '@angular/cdk/drag-drop'
import { Item } from './item.model'
@Component({
selector: 'app-drag-drop-lists',
templateUrl: './drag-drop-lists.component.html',
styleUrls: ['./drag-drop-lists.component.scss'],
})
export class DragDropListsComponent implements OnInit {
// Data for our two lists
leftItems: Item[] = []
rightItems: Item[] = []
// Track which items are selected in each list
selectedLeftItems: Item[] = []
selectedRightItems: Item[] = []
ngOnInit(): void {
// Initialize with sample data
this.leftItems = [
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' },
{ id: 3, name: 'Item 3' },
{ id: 4, name: 'Item 4' },
{ id: 5, name: 'Item 5' },
{ id: 6, name: 'Item 6' },
{ id: 7, name: 'Item 7' },
{ id: 8, name: 'Item 8' },
]
this.rightItems = [
{ id: 9, name: 'Item 9' },
{ id: 10, name: 'Item 10' },
]
}
}
Notice how we're maintaining separate arrays for the items and the selected items. This separation is crucial for our multi-select functionality.
Building the HTML Template
Now, let's create the UI with two lists and action buttons between them:
<div class="drag-drop-container">
<!-- Left List -->
<div class="list-container">
<mat-card>
<mat-card-header>
<mat-card-title>Source List</mat-card-title>
</mat-card-header>
<mat-card-content>
<div
cdkDropList
#leftList="cdkDropList"
[cdkDropListData]="leftItems"
[cdkDropListConnectedTo]="[rightList]"
class="item-list"
(cdkDropListDropped)="drop($event)"
>
<div
*ngFor="let item of leftItems; trackBy: trackById"
class="item-box"
cdkDrag
[cdkDragData]="item"
[class.selected]="isSelected(item, 'left')"
(click)="toggleSelect(item, 'left')"
>
{{ item.name }}
</div>
</div>
</mat-card-content>
</mat-card>
</div>
<!-- Action Buttons -->
<div class="action-buttons">
<button
mat-raised-button
color="primary"
(click)="moveSelectedToRight()"
[disabled]="!hasSelectedItems('left')"
>
<mat-icon>arrow_forward</mat-icon> Move to Right
</button>
<button
mat-raised-button
color="accent"
(click)="moveSelectedToLeft()"
[disabled]="!hasSelectedItems('right')"
>
<mat-icon>arrow_back</mat-icon> Move to Left
</button>
</div>
<!-- Right List -->
<div class="list-container">
<mat-card>
<mat-card-header>
<mat-card-title>Target List</mat-card-title>
</mat-card-header>
<mat-card-content>
<div
cdkDropList
#rightList="cdkDropList"
[cdkDropListData]="rightItems"
[cdkDropListConnectedTo]="[leftList]"
class="item-list"
(cdkDropListDropped)="drop($event)"
>
<div
*ngFor="let item of rightItems; trackBy: trackById"
class="item-box"
cdkDrag
[cdkDragData]="item"
[class.selected]="isSelected(item, 'right')"
(click)="toggleSelect(item, 'right')"
>
{{ item.name }}
</div>
</div>
</mat-card-content>
</mat-card>
</div>
</div>
Breaking down the key parts:
- The
cdkDropList
directive makes our container a drop target - We connect the two lists with
[cdkDropListConnectedTo]
- Each item gets the
cdkDrag
directive to make it draggable - We add a
(click)
handler to toggle selection - The
[class.selected]
binding applies styling to selected items - Buttons call methods to move selected items between lists
- We've added
trackBy
for performance optimization
Selection Functionality
The core of our multi-select feature is the ability to select items by clicking on them:
/**
* Toggle selection state of an item
*/
toggleSelect(item: Item, list: 'left' | 'right'): void {
if (list === 'left') {
const index = this.selectedLeftItems.findIndex(i => i.id === item.id);
if (index === -1) {
this.selectedLeftItems.push(item); // Select the item
} else {
this.selectedLeftItems.splice(index, 1); // Deselect the item
}
} else {
// Same logic for right list
const index = this.selectedRightItems.findIndex(i => i.id === item.id);
if (index === -1) {
this.selectedRightItems.push(item);
} else {
this.selectedRightItems.splice(index, 1);
}
}
}
/**
* Check if an item is selected
*/
isSelected(item: Item, list: 'left' | 'right'): boolean {
if (list === 'left') {
return this.selectedLeftItems.some(i => i.id === item.id);
} else {
return this.selectedRightItems.some(i => i.id === item.id);
}
}
/**
* Check if there are any selected items in a list
*/
hasSelectedItems(list: 'left' | 'right'): boolean {
return list === 'left'
? this.selectedLeftItems.length > 0
: this.selectedRightItems.length > 0;
}
/**
* Tracking function for ngFor performance
*/
trackById(index: number, item: Item): number {
return item.id;
}
What's happening here:
toggleSelect()
adds or removes an item from the selection arrayisSelected()
checks if an item is in the selection arrayhasSelectedItems()
helps us enable/disable the transfer buttonstrackById()
optimizes rendering performance for large lists
The Heart of Drag and Drop
The drop()
method handles what happens when a user drops items:
/**
* Handle drop events between lists
*/
drop(event: CdkDragDrop<Item[]>) {
if (event.previousContainer === event.container) {
// Reordering within the same list
moveItemInArray(
event.container.data,
event.previousIndex,
event.currentIndex
);
} else {
// Moving between lists
if (this.isSingleItemDrag(event)) {
// Single item transfer
transferArrayItem(
event.previousContainer.data,
event.container.data,
event.previousIndex,
event.currentIndex
);
} else {
// Multi-item transfer - our custom logic
this.handleMultiItemDrag(event);
}
}
// Clear selections after completing the operation
this.clearSelections();
}
/**
* Check if this is a single item drag operation
*/
private isSingleItemDrag(event: CdkDragDrop<Item[]>): boolean {
const sourceList = event.previousContainer.id === 'cdk-drop-list-0' ? 'left' : 'right';
const draggedItem = event.item.data;
// Check if the dragged item is part of a multi-selection
return sourceList === 'left'
? !this.selectedLeftItems.some(item => item.id === draggedItem.id) || this.selectedLeftItems.length === 0
: !this.selectedRightItems.some(item => item.id === draggedItem.id) || this.selectedRightItems.length === 0;
}
This is where things get interesting:
- We first check if we're reordering within a list or moving between lists
- If moving between lists, we decide whether this is a single item drag or a multi-item drag
- For single items, we use the CDK's built-in
transferArrayItem
function - For multiple items, we need custom logic
The Multi-Item Drag Magic
This is where the real magic happens - handling multiple selected items during a drag:
/**
* Custom logic to handle dragging multiple selected items
*/
private handleMultiItemDrag(event: CdkDragDrop<Item[]>) {
const sourceList = event.previousContainer.id === 'cdk-drop-list-0' ? 'left' : 'right';
const draggedItem = event.item.data;
const selectedItems = sourceList === 'left' ? this.selectedLeftItems : this.selectedRightItems;
// Fall back to single item drag if the dragged item isn't in selection
if (!selectedItems.some(item => item.id === draggedItem.id)) {
transferArrayItem(
event.previousContainer.data,
event.container.data,
event.previousIndex,
event.currentIndex
);
return;
}
// Find indices of all selected items
const selectedIndices = selectedItems.map(item =>
event.previousContainer.data.findIndex(listItem => listItem.id === item.id)
).filter(index => index !== -1).sort((a, b) => a - b);
// Move all selected items while adjusting for removed items
for (let i = 0; i < selectedIndices.length; i++) {
// Adjust index based on already removed items
const adjustedIndex = selectedIndices[i] - i;
const item = event.previousContainer.data[adjustedIndex];
// Remove from source
event.previousContainer.data.splice(adjustedIndex, 1);
// Add to target at appropriate position
const targetIndex = event.currentIndex > event.previousIndex
? event.currentIndex - i
: event.currentIndex;
event.container.data.splice(targetIndex, 0, item);
}
}
Let's break down what's happening here:
- We determine which list is the source and which items are selected
- If the dragged item isn't in the selection, we fall back to single-item behavior
- We find the indices of all selected items in the source list
- We move each selected item one by one, carefully adjusting indices as we go
- The trickiest part is adjusting indices since removing items changes the positions of subsequent items
Button-Based Transfer
Not all users prefer drag-and-drop, so we also provide button controls for better accessibility:
/**
* Move selected items from left to right
*/
moveSelectedToRight(): void {
if (this.selectedLeftItems.length === 0) return;
// Add selected items to right list
this.rightItems = [...this.rightItems, ...this.selectedLeftItems];
// Remove them from left list
this.leftItems = this.leftItems.filter(
item => !this.selectedLeftItems.some(selected => selected.id === item.id)
);
this.clearSelections();
}
/**
* Move selected items from right to left
*/
moveSelectedToLeft(): void {
if (this.selectedRightItems.length === 0) return;
// Add selected items to left list
this.leftItems = [...this.leftItems, ...this.selectedRightItems];
// Remove them from right list
this.rightItems = this.rightItems.filter(
item => !this.selectedRightItems.some(selected => selected.id === item.id)
);
this.clearSelections();
}
/**
* Clear all selections
*/
clearSelections(): void {
this.selectedLeftItems = [];
this.selectedRightItems = [];
}
These methods:
- Add all selected items to the target list
- Remove those items from the source list
- Clear selections afterward
Styling the Interface
The visual presentation is crucial for usability and user feedback:
.drag-drop-container {
display: flex;
justify-content: space-between;
align-items: flex-start;
gap: 20px;
padding: 20px 0;
}
.list-container {
flex: 1;
max-width: 400px;
min-width: 250px;
}
.item-list {
min-height: 400px;
border: solid 1px #ccc;
border-radius: 4px;
background-color: white;
overflow: auto;
}
.item-box {
padding: 15px;
border-bottom: solid 1px #ddd;
border-left: 4px solid transparent;
color: rgba(0, 0, 0, 0.87);
cursor: pointer;
background: white;
transition: all 200ms cubic-bezier(0, 0, 0.2, 1);
display: flex;
align-items: center;
}
.item-box:hover {
background-color: #f5f5f5;
}
// Highlighted state for selected items
.selected {
background-color: #e3f2fd !important;
border-left: 4px solid #1976d2 !important;
}
// Drag animation classes
.cdk-drag-preview {
box-sizing: border-box;
border-radius: 4px;
box-shadow:
0 5px 5px -3px rgba(0, 0, 0, 0.2),
0 8px 10px 1px rgba(0, 0, 0, 0.14),
0 3px 14px 2px rgba(0, 0, 0, 0.12);
}
.cdk-drag-placeholder {
opacity: 0.3;
}
.cdk-drag-animating {
transition: transform 250ms cubic-bezier(0, 0, 0.2, 1);
}
.action-buttons {
display: flex;
flex-direction: column;
justify-content: center;
gap: 10px;
}
/* Responsive design for mobile */
@media (max-width: 768px) {
.drag-drop-container {
flex-direction: column;
}
.list-container {
max-width: 100%;
}
.action-buttons {
flex-direction: row;
padding: 10px 0;
}
}
The key styling elements:
- A flex container to position the lists and buttons
- Clear visual feedback for selected items
- Smooth animations and shadows for drag operations
- Responsive design that adapts to mobile devices
Real-World Applications of Multi-Select Drag and Drop
This pattern is extremely useful in many common interfaces:
1. Project Management Tools
Move multiple tasks between sprint backlogs, or assign several stories to a developer at once.
interface Task {
id: number
title: string
priority: 'high' | 'medium' | 'low'
assignee?: string
}
2. Email Clients
Select multiple emails and move them to different folders or apply bulk actions.
3. Content Management Systems
Organize articles, posts, or media assets into different categories with multi-select capabilities.
4. E-commerce Admin Panels
Categorize products by selecting multiple items and moving them to different collections.
5. Playlist Management
Select multiple songs and add them to different playlists in music applications.
Performance Optimization for Large Lists
If your lists contain hundreds of items, consider these optimizations:
// Implement virtual scrolling with Angular CDK
import { ScrollingModule } from '@angular/cdk/scrolling';
// In your template
<cdk-virtual-scroll-viewport itemSize="50" class="item-list">
<div *cdkVirtualFor="let item of leftItems; trackBy: trackById"
class="item-box"
cdkDrag
[cdkDragData]="item"
[class.selected]="isSelected(item, 'left')"
(click)="toggleSelect(item, 'left')">
{{ item.name }}
</div>
</cdk-virtual-scroll-viewport>
Keyboard Accessibility Enhancement
To make our component fully accessible, let's add keyboard shortcuts:
@HostListener('window:keydown', ['$event'])
handleKeyboardEvent(event: KeyboardEvent): void {
// Ctrl+Right Arrow to move selected items right
if (event.ctrlKey && event.key === 'ArrowRight') {
this.moveSelectedToRight();
event.preventDefault();
}
// Ctrl+Left Arrow to move selected items left
if (event.ctrlKey && event.key === 'ArrowLeft') {
this.moveSelectedToLeft();
event.preventDefault();
}
// Shift+Click for range selection would be implemented in the toggleSelect method
}
Integration with State Management
For larger applications, you might want to integrate this with NgRx:
// Action definitions
export const moveItems = createAction(
'[Drag Drop Component] Move Items',
props<{ items: Item[], source: string, target: string }>()
);
// In your component
moveSelectedToRight(): void {
if (this.selectedLeftItems.length === 0) return;
this.store.dispatch(moveItems({
items: this.selectedLeftItems,
source: 'left',
target: 'right'
}));
this.clearSelections();
}
Conclusion and Next Steps
Building a multi-select drag-and-drop interface in Angular requires careful implementation, but the result is a powerful, intuitive UI that gives users flexibility in how they organize data. By allowing both gesture-based and button-based interactions, you can create accessible interfaces that work across devices and accommodate different user preferences.
Ready to take this further? Try these enhancements:
- Add shift-click for range selection
- Implement custom drag previews showing the number of selected items
- Add filtering and search capabilities to the lists
- Create animations when items move between lists
- Add support for keyboard navigation within lists
Additional Resources
Have you implemented multi-select drag and drop in your Angular application? Share your experience in the comments below!
About the Author
I'm a Senior Angular Developer with over 7 years of experience building enterprise applications. I specialize in creating intuitive, accessible user interfaces and sharing knowledge with the developer community. Follow me for more advanced Angular tutorials.
This article was last updated on March 31, 2025