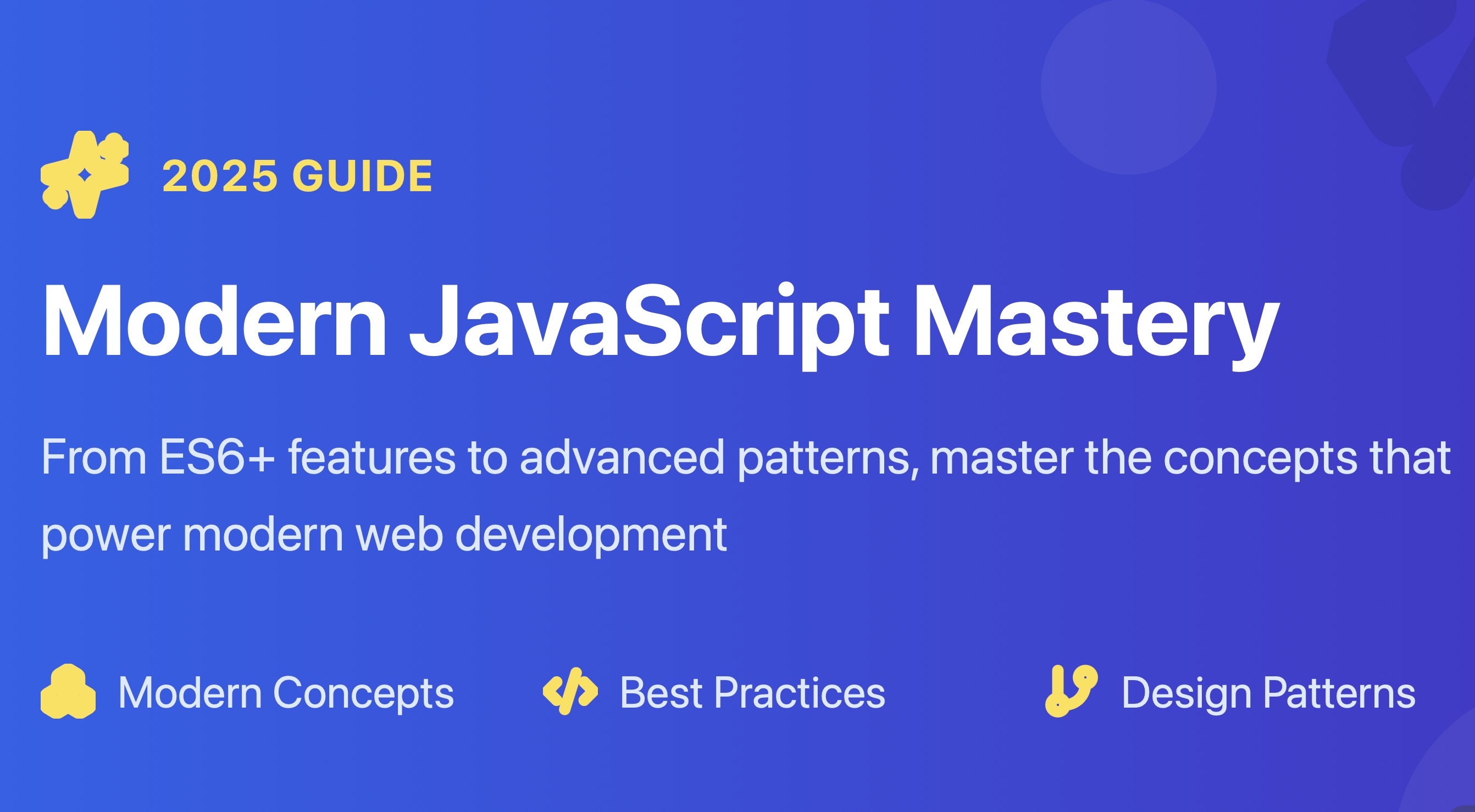
JavaScript 101: Essential Concepts Every Developer Should Master (2025 Guide)
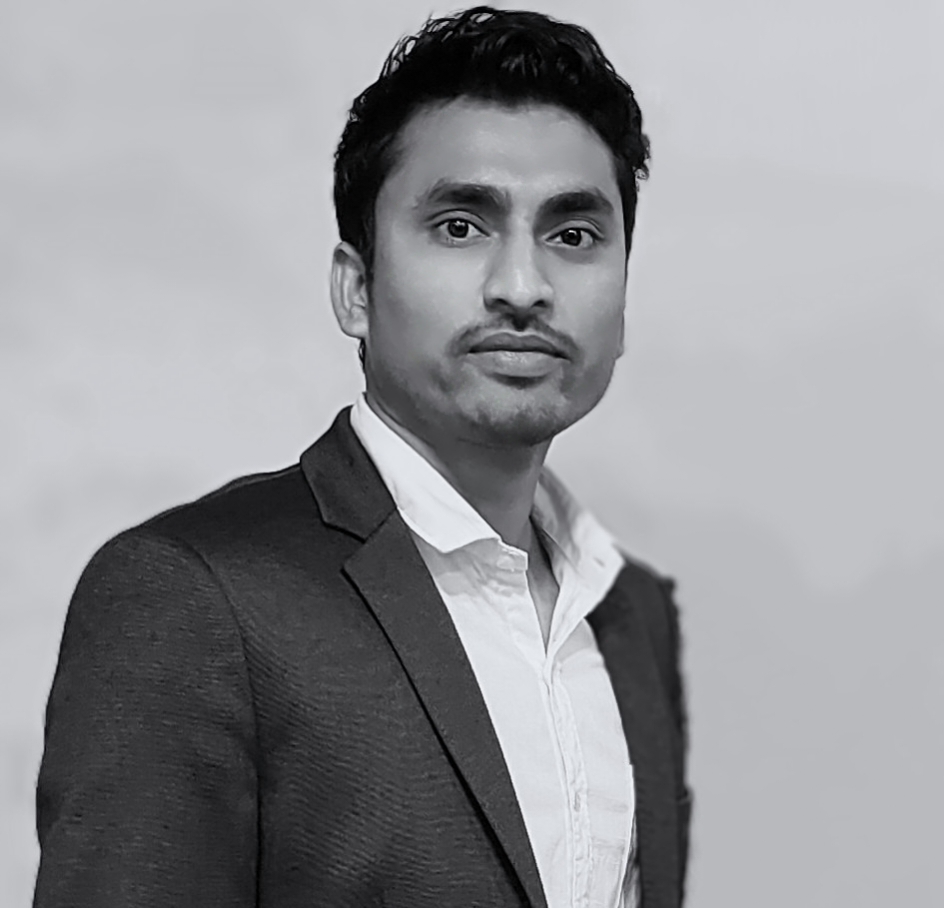
By Amaresh Adak
JavaScript has evolved from a simple browser scripting language into a powerful, versatile programming language that powers modern web applications, server-side systems, and even desktop software. As we move through 2025, understanding JavaScript's core concepts and advanced features is essential for any developer looking to build robust, efficient applications.
Here’s the updated Table of Contents with the point numbers added after every hash:
Table of Contents
- Modern JavaScript Fundamentals
- Advanced Data Types and Structures
- Functions, Closures, and Scope
- Object-Oriented Programming
- Modern Asynchronous Programming
- ES2025 Features
- Error Handling and Debugging
- Performance Optimization
- Testing and Quality Assurance
- Security Best Practices
- Design Patterns and Architecture
- Integration with Modern Frameworks
- Common Pitfalls and Solutions
- Best Practices and Tips
1. Modern JavaScript Fundamentals
Modern JavaScript introduces several fundamental concepts that form the foundation of effective JavaScript development.
Variable Declarations
The language offers three ways to declare variables, each serving a specific purpose:
// const: for values that won't be reassigned
const API_KEY = 'xyz123';
// let: for values that will change
let userScore = 0;
// var: legacy declaration (avoid in modern code)
// var statusCode = 200; // Not recommended
Understanding when to use each type:
- Use
const
by default for better code clarity and preventing accidental reassignment - Choose
let
when you need to reassign values - Avoid
var
due to its function-scoping and hoisting behavior
Data Types and Modern Features
JavaScript includes both primitive and complex data types, with modern features enhancing their usability:
// Template literals for string interpolation
const username = "Sarah";
const message = `Welcome ${username}!`;
// Modern number methods
const price = Number.parseFloat("99.99");
const isValid = Number.isInteger(42);
// Symbol for unique identifiers
const id = Symbol('userId');
Key concepts to understand:
- Template literals provide cleaner string interpolation
- Modern number methods offer safer type conversion
- Symbols create truly unique identifiers
2. Advanced Data Types and Structures
Enhanced Array Operations
Modern JavaScript provides powerful array methods for data manipulation:
const items = [
{ id: 1, name: "Laptop", price: 999 },
{ id: 2, name: "Phone", price: 699 },
{ id: 3, name: "Tablet", price: 499 }
];
// Filter expensive items
const expensive = items.filter(item => item.price > 500);
// Transform data structure
const names = items.map(item => item.name);
// Calculate total
const total = items.reduce((sum, item) => sum + item.price, 0);
Important array methods to master:
- filter() for selecting elements
- map() for transforming elements
- reduce() for aggregating values
- find() for locating specific elements
Maps and Sets
These modern collections solve specific problems more elegantly than objects and arrays:
// Map for key-value pairs
const userRoles = new Map([
['admin@example.com', 'ADMIN'],
['user@example.com', 'USER']
]);
// Set for unique values
const uniqueTags = new Set(['javascript', 'web', 'javascript']);
console.log([...uniqueTags]); // ['javascript', 'web']
Benefits of using Maps and Sets:
- Maps allow any type as keys
- Sets automatically handle uniqueness
- Both maintain insertion order
- Built-in size property and utility methods
3. Functions, Closures, and Scope
Modern Function Patterns
JavaScript functions have evolved with new syntax and capabilities:
// Arrow functions
const multiply = (a, b) => a * b;
// Default parameters
const greet = (name = 'Guest') => `Hello, ${name}!`;
// Rest parameters
const sum = (...numbers) => numbers.reduce((a, b) => a + b, 0);
Key function features:
- Arrow functions provide concise syntax
- Default parameters simplify function signatures
- Rest parameters handle variable arguments
Closures in Practice
Closures enable powerful patterns for data privacy and state management:
function createCounter(initialValue = 0) {
let count = initialValue;
return {
increment: () => ++count,
getValue: () => count
};
}
const counter = createCounter(10);
Understanding closures:
- Functions remember their creation scope
- Enable private variables and state
- Useful for data encapsulation
4. Object-Oriented Programming
Modern Class Syntax
Classes in modern JavaScript provide a clean way to implement OOP:
class BankAccount {
#balance = 0; // Private field
deposit(amount) {
if (amount <= 0) throw new Error('Invalid amount');
this.#balance += amount;
}
get balance() {
return this.#balance;
}
}
Key class features:
- Private fields with # prefix
- Getter/setter methods
- Constructor for initialization
- Method definitions
5. Modern Asynchronous Programming
Promises and Async/Await
Asynchronous operations have become more manageable:
// Using async/await
async function fetchUserData(userId) {
try {
const response = await fetch(`/api/users/${userId}`);
return await response.json();
} catch (error) {
console.error('Error:', error);
throw error;
}
}
// Promise combinations
const [users, posts] = await Promise.all([
fetchUsers(),
fetchPosts()
]);
Asynchronous programming concepts:
- Promises represent future values
- async/await simplifies asynchronous code
- Error handling with try/catch
- Promise combinators for complex scenarios
6. ES2025 Features
Latest JavaScript Enhancements
The newest ECMAScript features improve developer productivity:
// Pattern matching (new in ES2025)
const response = await fetch('/api/data');
match (response.status) {
case 200 -> handleSuccess(response)
case 404 -> handleNotFound()
default -> handleError(response)
}
// Top-level await
const config = await loadConfiguration();
New features to explore:
- Pattern matching for cleaner conditionals
- Top-level await in modules
- Enhanced type checking capabilities
7. Error Handling and Debugging
Modern Error Management
Proper error handling is crucial for robust applications:
class ValidationError extends Error {
constructor(message, field) {
super(message);
this.name = 'ValidationError';
this.field = field;
}
}
try {
await validateUserData(userData);
} catch (error) {
if (error instanceof ValidationError) {
console.error(`${error.field}: ${error.message}`);
}
}
Error handling best practices:
- Create custom error classes
- Use try/catch blocks effectively
- Implement proper error logging
- Handle different error types appropriately
8. Performance Optimization
Efficient Code Patterns
Optimize your applications for better performance:
// Debouncing example
const debounce = (fn, delay) => {
let timeoutId;
return (...args) => {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => fn(...args), delay);
};
};
const searchAPI = debounce((query) => {
// API call here
}, 300);
Performance considerations:
- Implement debouncing and throttling
- Use appropriate data structures
- Optimize loops and iterations
- Manage memory effectively
9. Testing and Quality Assurance
Modern Testing Approaches
Testing ensures code reliability and maintainability:
// Jest testing example
describe('User Authentication', () => {
test('should validate correct password', async () => {
const isValid = await validatePassword('correct123');
expect(isValid).toBe(true);
});
test('should reject invalid password', async () => {
await expect(
validatePassword('')
).rejects.toThrow('Invalid password');
});
});
Testing fundamentals:
- Write unit tests for individual components
- Implement integration testing
- Practice test-driven development (TDD)
- Use modern testing frameworks
10. Security Best Practices
Secure Coding Patterns
Implement security measures to protect your applications:
// Input sanitization
function sanitizeInput(input) {
return input
.replace(/[<>]/g, '')
.trim()
.slice(0, 100); // Length limit
}
// Secure token handling
const secureToken = crypto.randomBytes(32).toString('hex');
Security considerations:
- Sanitize all user inputs
- Use HTTPS for data transmission
- Implement proper authentication
- Follow OWASP security guidelines
11. Design Patterns and Architecture
Common Design Patterns
Implement proven solutions to common problems:
// Singleton pattern
class Configuration {
static #instance;
static getInstance() {
if (!this.#instance) {
this.#instance = new Configuration();
}
return this.#instance;
}
}
// Observer pattern
class EventEmitter {
#listeners = new Map();
on(event, callback) {
if (!this.#listeners.has(event)) {
this.#listeners.set(event, new Set());
}
this.#listeners.get(event).add(callback);
}
}
Key design patterns:
- Singleton for shared resources
- Observer for event handling
- Factory for object creation
- Module for code organization
12. Integration with Modern Frameworks
Framework Integration
Modern JavaScript works seamlessly with popular frameworks:
// React component example
const UserProfile = ({ user }) => {
const [loading, setLoading] = useState(false);
useEffect(() => {
// Side effects here
}, [user]);
return <div>{user.name}</div>;
};
// Vue.js component
const app = createApp({
data() {
return {
message: 'Hello Vue!'
};
}
});
Framework considerations:
- Understanding component lifecycle
- State management patterns
- Virtual DOM concepts
- Framework-specific best practices
13. Common Pitfalls and Solutions
Avoiding Common Mistakes
Learn from common JavaScript pitfalls:
// Type coercion issues
if (value === null || value === undefined) {
// Safer than using == null
}
// Async handling mistakes
// Wrong:
promise.then(handleSuccess).catch(handleError);
// Correct:
promise
.then(handleSuccess)
.catch(handleError)
.finally(cleanup);
Common pitfalls to avoid:
- Type coercion issues
- Callback hell
- Memory leaks
- Scope-related bugs
14. Best Practices and Tips
Coding Standards and Practices
Follow established best practices:
// Clear variable naming
const isUserActive = user.lastLogin > Date.now() - TIMEOUT;
// Function documentation
/**
* Processes user data and returns normalized result.
* @param {Object} userData - Raw user data
* @returns {Promise<Object>} Normalized user data
*/
async function processUserData(userData) {
// Implementation
}
Best practices to follow:
- Use clear, descriptive names
- Write documentation
- Follow consistent formatting
- Keep functions focused and small
Conclusion
Mastering JavaScript requires understanding both its fundamental concepts and modern features. This guide has covered the essential aspects of JavaScript development, from basic syntax to advanced patterns and best practices. Key takeaways include:
- Embrace modern JavaScript features
- Write clean, maintainable code
- Implement proper error handling
- Focus on security and performance
- Stay updated with new developments
Remember that becoming proficient in JavaScript is an ongoing journey. Continue practicing these concepts, building real-world applications, and staying current with the latest features and best practices.