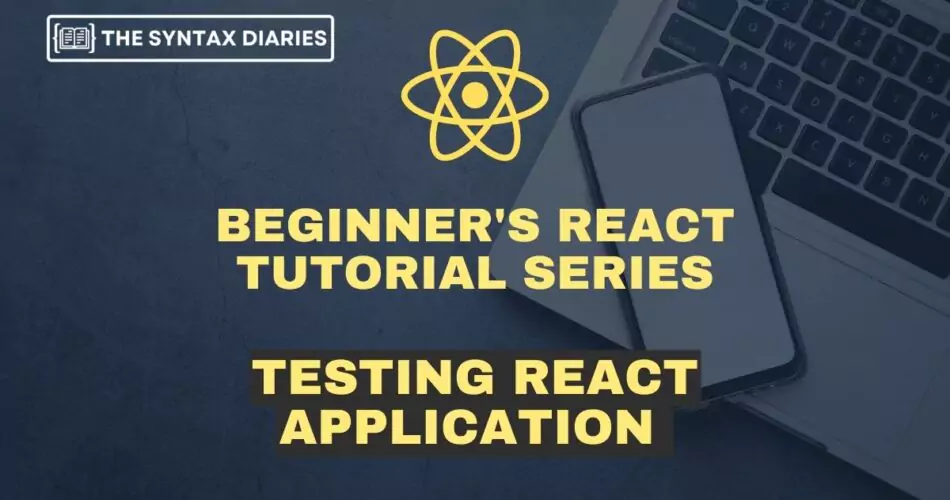
Unit Testing React Components: A Comprehensive Guide
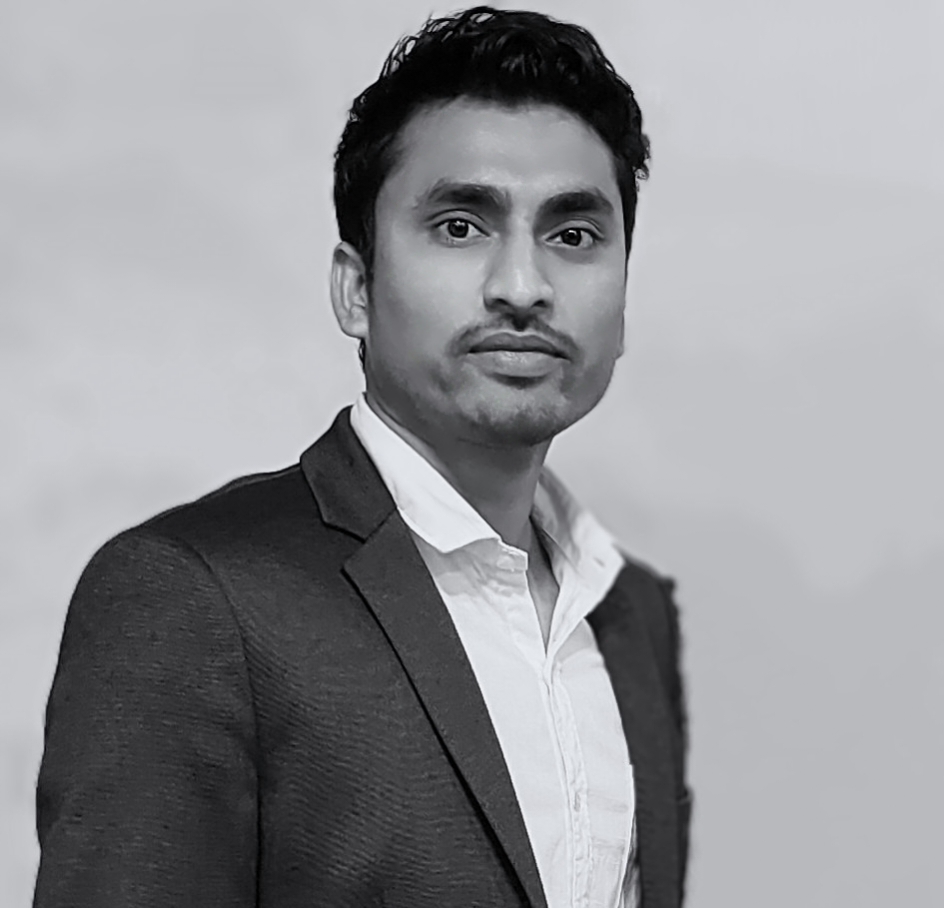
By Amaresh Adak
Introduction 🧪📝
Welcome to a comprehensive guide on Unit Testing React components! Testing your React components is essential for ensuring their functionality, reliability, and a great user experience. In this guide, we will walk you through the process of testing React components step by step, providing you with practical examples and tips along the way. Let’s dive in and explore the world of testing in React! 🚀
Why Test React Components?
Testing React components is crucial for several reasons. By testing your components, you can:
Ensure that they work as expected and provide a seamless user experience.✅
Identify and fix issues early in the development process, saving time and effort.✅
Enhance code maintainability, allowing for easier refactoring and modification.✅
Act as documentation, providing insights into component usage and behavior.✅
Now, let’s explore the different types of testing available for React components. 🧪
Types of Testing in React
When it comes to testing React components, there are three primary types of tests you should know about:
- Unit Testing React: Unit tests focus on testing individual units of code, in this case, React components, in isolation. They ensure that each component behaves as intended independently of other components.
- Integration Testing: Integration tests verify how multiple components work together as a whole. They ensure that components interact correctly and produce the desired outcome.
- Snapshot Testing: Snapshot tests capture the output of a component and compare it to a previously saved “snapshot.” They help detect unintended changes in the component’s appearance or structure.
Now that you have an overview of the testing types, let’s set up the testing environment and start writing tests! 🛠️
Setting Up the Testing Environment
Before we begin testing React components, we need to set up the testing environment. Here are the steps:
- Choose a Testing Framework: Jest is a popular choice for testing React components. It’s easy to set up and provides powerful features tailored for React testing.
- Install Dependencies: Open your project’s terminal and run the following command to install Jest and other necessary dependencies:
npm install --save-dev jest react-test-renderer
This command installs Jest as a development dependency and the [react-test-renderer](https://legacy.reactjs.org/docs/test-renderer.html)
package, which allows us to render React components in our tests.
With the testing environment set up, let’s move on to writing our tests! ✍️
Writing Unit Tests
Unit testing is an essential part of testing React components. It focuses on testing individual components in isolation to ensure their functionality. Let’s walk through the process of writing effective unit tests step by step.
Step 1: Testing Component Rendering
The first step in unit testing a React component is to verify that it renders correctly. Let’s consider a simple Button
component:
import React from "react"
function Button({ label }) {
return <button>{label}</button>
}
To test the rendering of this component, we can use the react-test-renderer
package:
import React from "react"
import renderer from "react-test-renderer"
import Button from "./Button"
test("Button renders correctly", () => {
const component = renderer.create(<Button label="Click me" />)
const tree = component.toJSON()
expect(tree).toMatchSnapshot()
})
In this test, we create an instance of the Button
component with the desired props using renderer.create
. Then, we convert the component to a JSON representation using component.toJSON()
. Finally, we use the toMatchSnapshot
matcher from Jest to compare the JSON output with a previously saved snapshot.
Step 2: Testing Component Behavior
In addition to rendering, we also want to test the behavior of our components. Let’s consider a Counter
component that increments a counter value when a button is clicked:
import React, { useState } from "react"
function Counter() {
const [count, setCount] = useState(0)
const increment = () => {
setCount(count + 1)
}
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
)
}
To test the behavior of this component, we can use React Testing Library:
import React from "react"
import { render, fireEvent } from "@testing-library/react"
import Counter from "./Counter"
test("Counter increments count on button click", () => {
const { getByText } = render(<Counter />)
const countElement = getByText("Count: 0")
const button = getByText("Increment")
fireEvent.click(button)
expect(countElement.textContent).toBe("Count: 1")
})
Initially, we render the Counter component using render
from React Testing Library. Then, we obtain the count element and the button element using getByText
. Next, we simulate a click event on the button using fireEvent.click
. Finally, we assert that the count element displays the expected count after the button click.
Step 3: Mocking Dependencies
React components often have dependencies, such as API calls or external libraries. To isolate our component and focus on its behavior, we can mock these dependencies. Let’s consider a UserList
component that fetches a list of users from an API:
import React, { useState, useEffect } from "react"
import axios from "axios"
function UserList() {
const [users, setUsers] = useState([])
useEffect(() => {
axios.get("/api/users").then((response) => {
setUsers(response.data)
})
}, [])
return (
<ul>
{users.map((user) => (
<li key={user.id}>{user.name}</li>
))}
</ul>
)
}
To test this component without making actual API calls, we can use Jest’s mocking capabilities:
import React from "react"
import { render, waitFor } from "@testing-library/react"
import axios from "axios"
import UserList from "./UserList"
test("UserList renders correctly", async () => {
const mockUsers = [
{ id: 1, name: "John Doe" },
{ id: 2, name: "Jane Smith" },
]
jest.spyOn(axios, "get").mockResolvedValue({ data: mockUsers })
const { getByText } = render(<UserList />)
await waitFor(() => {
expect(getByText("John Doe")).toBeInTheDocument()
expect(getByText("Jane Smith")).toBeInTheDocument()
})
})
First, we use jest.spyOn
to mock the axios.get
function. This allows us to control the data that is returned from the API call. We then resolve the mock with some mock user data.
Integration Testing with React Testing Library
Integration testing is a type of testing that focuses on how multiple components work together. React Testing Library (RTL) provides utilities to render components and simulate user interactions, making it ideal for integration testing.
Step 1: Rendering Components
To test component interactions, we need to render the components in a test environment. Let’s consider a simple `LoginForm
` component that handles user login:
import React from "react"
import { render, waitFor } from "@testing-library/react"
import axios from "axios"
import UserList from "./UserList"
test("UserList renders correctly", async () => {
const mockUsers = [
{ id: 1, name: "John Doe" },
{ id: 2, name: "Jane Smith" },
]
jest.spyOn(axios, "get").mockResolvedValue({ data: mockUsers })
const { getByText } = render(<UserList />)
await waitFor(() => {
expect(getByText("John Doe")).toBeInTheDocument()
expect(getByText("Jane Smith")).toBeInTheDocument()
})
})
To test the interaction between the LoginForm
and a hypothetical AuthService
, we can write an integration test:
import React from "react"
import { render, fireEvent } from "@testing-library/react"
import LoginForm from "./LoginForm"
test("LoginForm submits login data", () => {
const mockAuthService = {
login: jest.fn(),
}
const { getByPlaceholderText, getByText } = render(<LoginForm onLogin={mockAuthService.login} />)
const usernameInput = getByPlaceholderText("Username")
const passwordInput = getByPlaceholderText("Password")
const loginButton = getByText("Login")
fireEvent.change(usernameInput, { target: { value: "testuser" } })
fireEvent.change(passwordInput, { target: { value: "testpassword" } })
fireEvent.click(loginButton)
expect(mockAuthService.login).toHaveBeenCalledWith("testuser", "testpassword")
})
To test the login functionality of the LoginForm component, we first create a mock AuthService with a login function. Then, we render the LoginForm component and obtain the input fields and the login button using the getByPlaceholderText and getByText methods, respectively. Next, we simulate user input by using the fireEvent.change method to change the values of the input fields and the fireEvent. Click the method to click on the login button. Finally, we assert that the login function from the AuthService is called with the expected username and password values.
Conclusion
Congratulations! You’ve learned the basics of testing React components. By following these step-by-step examples, you can start testing your React components effectively. Remember to choose the appropriate testing type, set up the testing environment correctly, and write focused and maintainable tests. Regularly testing your React components will ensure their reliability and enhance the overall quality of your applications. Happy testing! 🧪🚀✅
FAQs
1. What is the purpose of testing React components? ❓
Testing React components ensures that they function as expected, identify and fix issues early, enhance code maintainability, and act as documentation.
2. What are the types of testing in React? ❓
The three primary types of testing in React are unit testing, integration testing, and snapshot testing.
3. How do I set up the testing environment for React components? ❓
Choose a testing framework like Jest, install the necessary dependencies, and configure the environment accordingly.
4. What are some best practices for testing React components? ❓
Isolate tests, write testable code, and keep tests maintainable. Follow guidelines for test organization, naming, and documentation.
5. How can I automate testing using CI/CD pipelines? ❓
Integrate testing into your CI/CD pipelines to automatically run tests whenever changes are pushed to the repository. Utilize parallel test execution to optimize testing time.
6. What are some common issues in testing React components? ❓
Asynchronous code, mocking dependencies, and environment differences can pose challenges in testing. Utilize appropriate techniques and tools to handle such issues.
7. Why is performance testing important for React components? ❓
Performance testing is a critical step in ensuring that a React application is performant. By identifying performance bottlenecks, developers can optimize components to improve the user experience. Profiling tools like React DevTools and React Profiler can assist in this process by providing insights into component rendering times.
Remember, practice and experimentation are key to becoming proficient in testing React components. Embrace the testing mindset, and enjoy the process of ensuring the reliability and quality of your React applications!
Happy testing! 😊