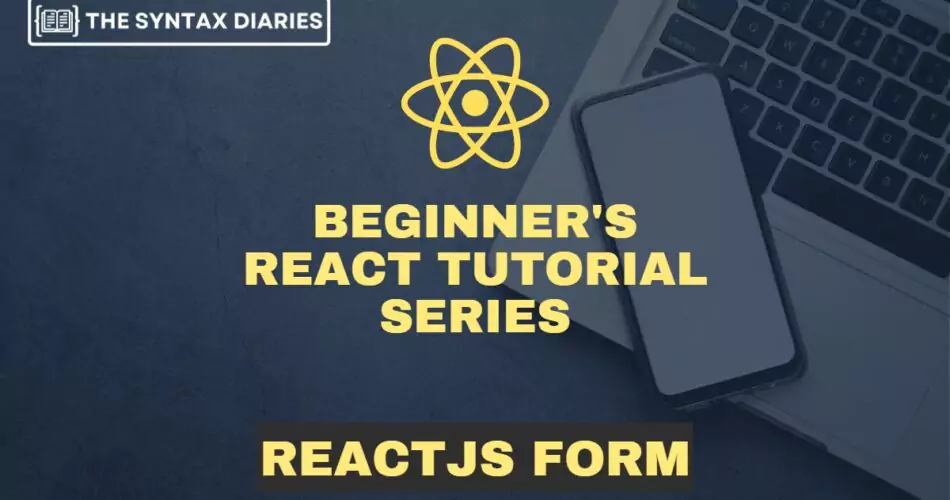
React Form Example: Simplifying User Input Management ???
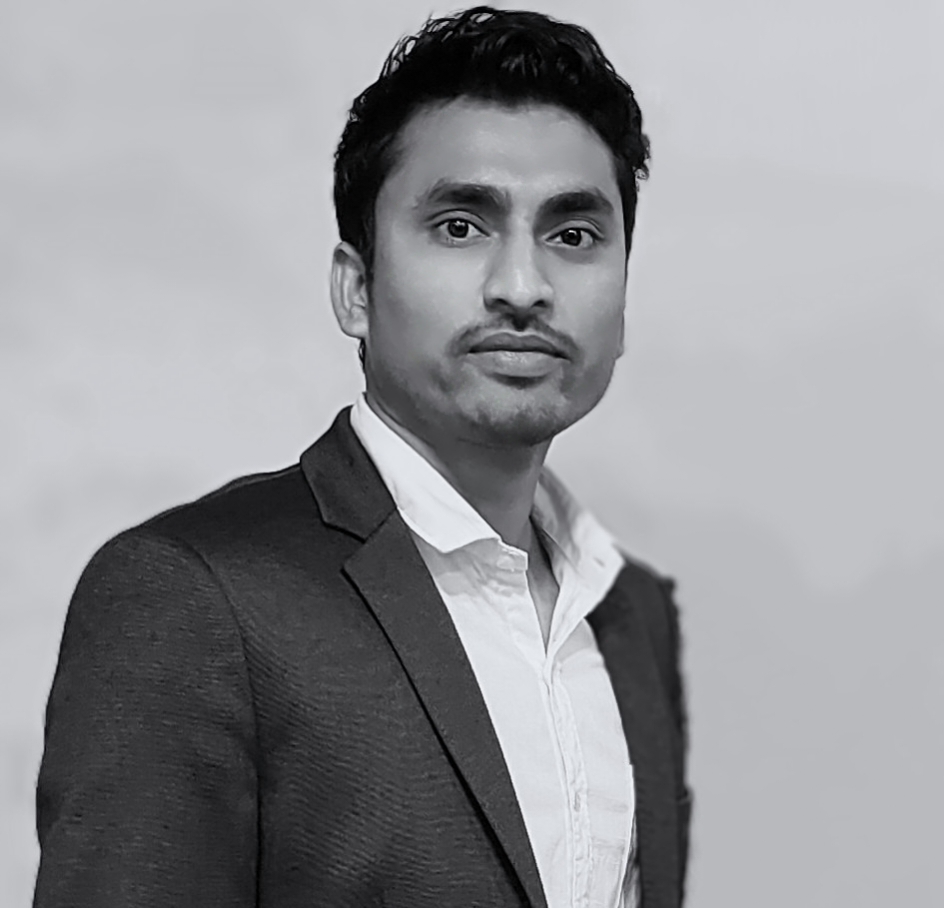
By Amaresh Adak
In this article, we will discuss forms with a practical React form example, enabling you to simplify user input and create interactive web forms for enhanced user experiences.
When it comes to building interactive web applications, managing user input is a fundamental aspect. React, a popular JavaScript library provides powerful tools and techniques for handling form inputs effectively. In this article, we will explore the world of React forms and learn how they simplify user input management, making the development process smoother and more efficient. 💡🌐✨
What are React forms?
React forms are a set of components and techniques that enable developers to create dynamic and interactive forms within their React applications. They allow users to input data, submit forms, and provide mechanisms for validating and processing that data. React forms provide a declarative approach to managing form state and capturing user input, making it easier to build complex forms with ease. 📝🔧📝
Benefits of using React forms
Using React forms offers several benefits for developers and end-users alike:
- Modularity: React forms are highly modular, allowing you to create reusable form components that can be easily composed together to build complex forms. This modularity promotes code reusability and maintainability, saving development time and effort. ♻️🔗💡
- State management: React’s state management capabilities make it straightforward to handle form inputs and keep track of their values. By using state, you can easily retrieve and update the form data. This enables you to create dynamic forms that respond to user interactions in real-time. ⚙️🔄📊
- Efficient rendering: React’s virtual DOM efficiently updates and re-renders only the necessary parts of the form, resulting in better performance and a smoother user experience. This optimization ensures that the form remains responsive, even in applications with complex and data-intensive forms. ⚡🖥️🎮
- Validation and error handling: React provides flexible options for form validation, making it simple to validate user input and provide meaningful error messages when necessary. This helps ensure that the data submitted through the form meets the required criteria and improves data quality and integrity. 🔍✅⛔
- Enhanced user experience: React forms enable real-time feedback, allowing users to see instant validation results, error messages, or success notifications as they interact with the form. This feedback helps users understand the correctness of their input and provides a more engaging and intuitive user experience. 👁️📢🤩
Setting up a basic form in React Form Example
To create a basic form in React, you’ll need to set up the necessary HTML elements and handle their events. Let’s consider React Form Example of a simple registration form in React. 📝📑📝
import React, { useState } from "react"
function RegistrationForm() {
const [name, setName] = useState("")
const [email, setEmail] = useState("")
const [password, setPassword] = useState("")
const handleNameChange = (e) => {
setName(e.target.value)
}
const handleEmailChange = (e) => {
setEmail(e.target.value)
}
const handlePasswordChange = (e) => {
setPassword(e.target.value)
}
const handleSubmit = (e) => {
e.preventDefault()
// Form submission logic
}
return (
<form onSubmit={handleSubmit}>
<label htmlFor="name">Name:</label>
<input type="text" id="name" value={name} onChange={handleNameChange} />
<label htmlFor="email">Email:</label>
<input type="email" id="email" value={email} onChange={handleEmailChange} />
<label htmlFor="password">Password:</label>
<input type="password" id="password" value={password} onChange={handlePasswordChange} />
<button type="submit">Register</button>
</form>
)
}
export default RegistrationForm
In this React Form example, we use the useState
hook to create state variables for the name
, email
, and password
fields. The value
attributes of the input fields are set to these state variables, ensuring that the form reflects the current values. The onChange
event handlers update the respective state variables whenever the user types into the input fields. The handleSubmit
function handles the form submission logic.
Handling form inputs with state
React provides the useState
hook, which allows you to manage the state of form inputs. By defining state variables and event handlers, you can capture user input and update the form data accordingly. 📊🖋️🔄
In the previous example, we used useState
to create state variables for name
, email
, and password
. The value
attributes of the input fields are set to these state variables, ensuring that the form reflects the current values. The onChange
event handlers update the state variables whenever the user types into the input fields.
const [name, setName] = useState("")
const [email, setEmail] = useState("")
const [password, setPassword] = useState("")
const handleNameChange = (e) => {
setName(e.target.value)
}
const handleEmailChange = (e) => {
setEmail(e.target.value)
}
const handlePasswordChange = (e) => {
setPassword(e.target.value)
}
By leveraging React’s state management, you can easily access the form data and perform any necessary operations, such as validation or submission.
React Form Example validation & error handling
Validating user input is crucial for maintaining data integrity and ensuring a smooth user experience. React provides various options for form validation, ranging from simple checks to more complex validation rules. 🔍✅🚦
One common approach is to perform validation within the event handlers. For example, you can add conditional logic to check if the input meets certain criteria and display error messages accordingly.
const [email, setEmail] = useState("")
const [emailError, setEmailError] = useState("")
const handleEmailChange = (e) => {
const value = e.target.value
setEmail(value)
if (!value.includes("@")) {
setEmailError("Please enter a valid email address.")
} else {
setEmailError("")
}
}
In this example, we check if the email input contains the ‘@’ symbol. If it doesn’t, we set the emailError
state variable with an appropriate error message. By conditionally rendering the error message, we can provide immediate feedback to the user.
Enhancing user experience with form feedback
Providing meaningful feedback to users is crucial for a positive user experience
. React forms allow you to enhance user experience by offering real-time feedback, validation messages, or success notifications as users interact with the form. 📢🌟👍
Let’s expand on the previous react form example and display the error message when the email input is invalid:
<label htmlFor="email">Email:</label>
<input
type="email"
id="email"
value={email}
onChange={handleEmailChange}
/>
{emailError && <span className="error">{emailError}</span>}
In this code snippet, we conditionally render the error message emailError
if it exists. This approach ensures that users receive immediate feedback when their input is invalid. By using CSS, you can style the error message to make it more noticeable and user-friendly.
Advanced form features in React
React offers several advanced features and libraries that can further enhance your form-building experience. These features include:
- Form libraries: Libraries like Formik, React Hook Form, and Final Form provide abstractions and utilities for managing complex forms, form validation, and state management.
- Conditional form fields: You can create dynamic forms that show or hide certain fields based on user input or specific conditions. This improves the form’s flexibility and usability.
- File uploads: React supports file upload functionality, allowing users to select and upload files through a form. You can handle file uploads using dedicated libraries or native browser APIs.
- Multi-step forms: With React, you can create multi-step forms that guide users through a series of steps, collecting data in an organized and intuitive manner.
These advanced features empower developers to build sophisticated forms that meet diverse requirements and provide a seamless user experience.
Best practices for building React forms
To build effective and user-friendly React forms, consider the following best practices:
- Keep forms simple: Aim for simplicity and clarity in form design. Avoid overwhelming users with unnecessary fields or complex layouts.
- Provide helpful guidance: Include clear instructions, placeholders, and validation messages to guide users and help them complete the form accurately.
- Use native form features: Leverage HTML5 form attributes like
required
andpattern
to perform basic client-side validation. This ensures that users can trust that your website or app will work as expected, regardless of the device or browser they are using. - Utilize form libraries: Consider using popular form libraries like Formik or React Hook Form, which offer additional features, form state management, and validation abstractions.
- Test thoroughly: Test your forms rigorously to ensure they work as expected across different browsers and devices. Validate user inputs on both the client and server sides to maintain data integrity.
Remember that building user-friendly forms is an iterative process. Continuously gather user feedback and make improvements to optimize the form’s usability and effectiveness.
Conclusion
React forms provide a powerful and flexible solution for managing user input in web applications. By understanding the fundamentals of React forms, handling form inputs with state, implementing validation and error handling, enhancing user experience with form feedback, and adopting best practices, you can create highly interactive and user-friendly forms.
Remember to stay up-to-date with the latest advancements and libraries in React forms to leverage their full potential and streamline the process of collecting and processing user data.
React Form Example: FAQs
1. Q: Can I use React forms with other JavaScript frameworks?
A: Yes, React forms can be integrated with other JavaScript frameworks or libraries. React’s flexibility allows you to use it alongside frameworks like Angular or Vue.js, enabling you to leverage the benefits of React forms within a larger application.
2. Q: Are there any limitations to React forms?
A: React forms offer extensive capabilities; however, they might not be suitable for all scenarios. In complex form scenarios with intricate data dependencies, you might consider using form libraries that provide additional features and abstractions.
3. Q: How can I handle form submission in React?
A: React forms offer extensive capabilities; however, they might not be suitable for all scenarios. In complex form scenarios with intricate data dependencies, you might consider using form libraries that provide additional features and abstractions.
4. Q: Can I style React forms?
A: Yes, you can style React forms using CSS or CSS-in-JS libraries like styled-components or Emotion. By adding custom styles, you can match the form’s appearance to your application’s overall design and branding.
5. Q: How can I handle complex form validations?
A: For complex form validations, you can utilize form validation libraries like Formik or Yup. These libraries provide advanced validation capabilities, including validation schemas, custom validation rules, and error message customization.