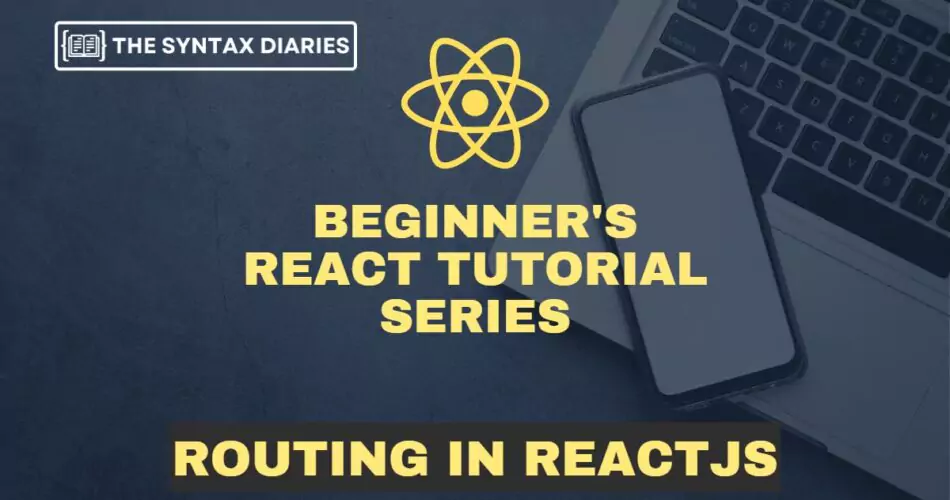
Routing in React is essential for modern web applications 🛤️✨. As web apps become more complex, users expect to be able to navigate between pages without having to reload the entire application. React routing, a JavaScript library makes this possible by enabling the creation of React single page apps (SPAs) that are both seamless and interactive.🚀🌐
1. Introduction to Routing in React and React Single Page App
🛣️✨Routing is the process of determining which component to render based on the current URL or user actions. In React, routing enables the creation of SPAs by allowing developers to define routes and map them to specific components. This ensures that the appropriate component is rendered when a user navigates to a specific URL or triggers a certain action.🚀🔀
What is React Single Page App?
A React Single Page App (SPA) refers to a web application built using React that operates as a single page. Instead of loading separate HTML pages for each interaction, an SPA dynamically updates the content on a single page in response to user actions. This approach offers a smoother and more interactive user experience as it eliminates the need for full page reloads. React SPAs leverage routing techniques to handle the navigation and rendering of different components without refreshing the entire page.
2. Setting Up a React Project
Before we dive into routing in React, let’s first set up a new React project. You can use Create React App, a popular tool for bootstrapping React applications, to quickly create a new project. Launch your terminal and execute the provided command:
npx create-react-app my-app
This command will create a new directory named my-app
with a basic React project structure.
3. Installing React Router
React Router is a widely-used library that provides routing capabilities for React applications. To install React Router, go to your project directory and execute the following command:
npm install react-router-dom
This command will install the necessary packages for React Router.
4. Basic Routing in React with React Router
Now that we have our React project set up and React Router installed, let’s start implementing basic routing. Open the src/App.js
file and replace its content with the following code:
import React from "react"
import { BrowserRouter as Router, Route, Switch } from "react-router-dom"
import Home from "./components/Home"
import About from "./components/About"
import Contact from "./components/Contact"
function App() {
return (
<Router>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</Switch>
</Router>
)
}
export default App
In the above code, we import the necessary components from React Router and define our routes using the Route
component. The exact
attribute ensures that the corresponding component is rendered only when the URL exactly matches the defined path.
5. Route Parameters and Dynamic Routing
Sometimes, we need to pass parameters in the URL to create dynamic routes. React Router allows us to define route parameters using the :paramName
syntax. Let’s modify our code to include a dynamic route:
import User from "./components/User"
function App() {
return (
<Router>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
<Route path="/user/:id" component={User} />
</Switch>
</Router>
)
}
In the above code, we added a new route /user/:id
that will match any URL starting with /user/
followed by a dynamic id
parameter. This enables us to access the id
parameter inside the User
component and render content based on it.
6. Nesting Routes
React Router allows us to nest routes within each other, providing a hierarchical structure to our application. This is useful when we want to render different components based on nested URLs. Here’s an illustrative example to further understand the concept:
import Dashboard from "./components/Dashboard"
import Profile from "./components/Profile"
import Settings from "./components/Settings"
function App() {
return (
<Router>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
<Route path="/dashboard">
<Dashboard>
<Route path="/dashboard/profile" component={Profile} />
<Route path="/dashboard/settings" component={Settings} />
</Dashboard>
</Route>
</Switch>
</Router>
)
}
In the above code, we created a nested route structure for a dashboard. The Dashboard
component acts as a layout for the nested routes, while Profile
and Settings
are rendered based on their respective paths under the /dashboard
route.
7. Redirects and NotFound Pages
Sometimes, we may need to redirect users to a different URL or show a custom “Not Found” page when a route doesn’t exist. React Router provides components for handling these scenarios.
To redirect users, we can use the Redirect
component. Here’s an example:
import { Redirect } from "react-router-dom"
function App() {
return (
<Router>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
<Route path="/dashboard" component={Dashboard} />
<Redirect to="/" />
</Switch>
</Router>
)
}
In the above code, if none of the defined routes match, the user will be redirected to the home page.
To handle “Not Found” scenarios, we can define a special route that matches all paths using the Route
component without a specified path
. This route should be placed at the end of the Switch
component, as shown below:
import NotFound from "./components/NotFound"
function App() {
return (
<Router>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
<Route path="/dashboard" component={Dashboard} />
<Route component={NotFound} />
</Switch>
</Router>
)
}
The NotFound
component will be rendered if the URL does not match any of the defined routes.
8. Using Link and NavLink Components
In React Router, we can use the Link
component to navigate between routes without reloading the entire page. The NavLink
component is similar to Link
, but it adds styling to the active link based on the current URL.
Here’s an example of using Link
and NavLink
components:
import { Link, NavLink } from "react-router-dom"
function Navigation() {
return (
<nav>
<ul>
<li>
<NavLink exact to="/" activeClassName="active">
Home
</NavLink>
</li>
<li>
<NavLink to="/about" activeClassName="active">
About
</NavLink>
</li>
<li>
<NavLink to="/contact" activeClassName="active">
Contact
</NavLink>
</li>
</ul>
</nav>
)
}
In the above code, the NavLink
components are used to create navigation links. The activeClassName
attribute is used to specify the class name for the active link, allowing us to style it differently.
9. Programmatic Navigation
In addition to using Link
and NavLink
components for navigation, React Router provides a history
object that allows programmatic navigation. We can push, replace, or go back to previous URLs using the history
object.
Here’s an example of programmatic navigation:
import { useHistory } from "react-router-dom"
function MyComponent() {
const history = useHistory()
const handleClick = () => {
history.push("/about")
}
return <button onClick={handleClick}>Go to About Page</button>
}
In the above code, the useHistory
hook is used to access the history
object. When the button is clicked, it triggers the handleClick
function, which programmatically navigates to the /about
page using history.push()
.
10. Route Guards and Authentication
In some cases, we may need to restrict access to certain routes based on user authentication or authorization. React Router allows us to implement route guards by creating higher-order components (HOCs) that wrap the protected routes.
Here’s an example of implementing route guards:
import { Route, Redirect } from "react-router-dom"
function PrivateRoute({ component: Component, isAuthenticated, ...rest }) {
return (
<Route
{...rest}
render={(props) => (isAuthenticated ? <Component {...props} /> : <Redirect to="/login" />)}
/>
)
}
In the above code, the PrivateRoute
component acts as a guard for protected routes. If the user is authenticated, the component is rendered; otherwise, they are redirected to the login page.
11. Code Splitting for Performance
As SPAs grow in complexity, it’s important to optimize performance by reducing the initial bundle size. Code splitting allows us to split our application code into smaller chunks that are loaded on-demand.
React provides a built-in mechanism for code splitting using dynamic imports and the React.lazy()
function. Here’s an example:
import React, { lazy, Suspense } from "react"
const AsyncComponent = lazy(() => import("./components/AsyncComponent"))
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<AsyncComponent />
</Suspense>
)
}
In the above code, the AsyncComponent
is loaded lazily when needed. The Suspense
component is used to display a fallback UI, such as a loading spinner, while the component is being loaded.
12. Handling 404 Errors
When a user navigates to a non-existent route, it’s important to provide a clear indication that the page doesn’t exist. We can create a custom 404 page to handle such cases.
Here’s an example of a 404 component:
codefunction NotFound() {
return (
<div>
<h1>404 - Page Not Found</h1>
<p>The page you are looking for does not exist.</p>
</div>
);
}
You can add the NotFound
component to your routing configuration as shown in a previous section.
13. Implementing Lazy Loading
Lazy loading is a clever approach to delay the loading of less important resources until they are genuinely required. This improves the initial load time of an application.
React Router provides a React.lazy()
function combined with dynamic imports to implement lazy loading. Here’s an example:
const Home = lazy(() => import("./components/Home"))
const About = lazy(() => import("./components/About"))
const Contact = lazy(() => import("./components/Contact"))
// ...
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
<Route component={NotFound} />
</Switch>
</Suspense>
)
}
In the above code, the Home
, About
, and Contact
components are loaded lazily when their respective routes are accessed.
14. SEO Considerations for React Single Page App
While SPAs provide a great user experience, they can present challenges for search engine optimization (SEO) due to the limited initial content available on the page. However, there are strategies to optimize React Single Page Apps (SPAs) for SEO:
- Pre-render critical content on the server: Server-side rendering (SSR) or static site generation (SSG) can be used to generate HTML with initial content on the server. This allows search engines to crawl and index the page.
- Use dynamic rendering: Dynamic rendering serves different versions of your page to search engines and users. You can provide a pre-rendered version for search engines and an interactive version for users.
- Implement metadata and Open Graph tags: Include relevant metadata, such as page title, description, and Open Graph tags, to provide search engines and social media platforms with essential information about your page.
- Submit a sitemap: Create a sitemap.xml file and submit it to search engines to ensure all your pages are discovered and indexed.
15. Frequently Asked Questions (FAQs)
Q1: What are the advantages of using Routing in React?
React offers several advantages for routing in single-page applications. Some of the key benefits include:
- Faster navigation and seamless page transitions without full page reload.
- Improved user experience with dynamic content updates.
- Efficient handling of complex application states using React’s component-based architecture.
- Compatibility with other React libraries and tools.
Q2: Can I use React Router with other UI frameworks or libraries?
Yes, React Router is designed to work seamlessly with other UI frameworks or libraries. It is independent of the rendering layer and can be integrated into any React application, regardless of the UI components being used.
Q3: Are there any alternatives to React Router for routing in React?
While React Router is the most popular routing library for React, there are a few alternatives you can explore. Some notable alternatives include Reach Router, Next.js, and React Navigation (for React Native applications). Each library has its own set of features and uses cases, so it’s important to evaluate your project requirements before making a choice.
Q4: How does routing in React impact SEO?
Routing in React primarily handles client-side navigation and rendering, which means search engine crawlers might face difficulties in indexing and understanding the content. However, with the advent of server-side rendering (SSR) techniques like Next.js, you can achieve better SEO by pre-rendering React components on the server and sending fully rendered HTML to the client.
Q5: Can I implement authentication and protected routes with React Router?
Yes, React Router provides features for implementing authentication and protecting routes. You can conditionally render components based on user authentication status, redirect users to login pages when necessary, and restrict access to certain routes for authenticated users only. React Router offers components like <Redirect>
and <PrivateRoute>
to handle these scenarios.
Q6: Is React Router suitable for large-scale applications?
Yes, React Router is suitable for large-scale applications as it offers flexibility, scalability, and robust routing capabilities. With proper code organization and optimization techniques, React Router can efficiently handle complex routing requirements in enterprise-level applications.
16. Conclusion
🌐🚀 Routing in React is a fundamental concept for creating SPAs. By using React Router, developers can implement efficient and dynamic routing in their applications, providing users with a seamless navigation experience. 🛤️💨 We covered various topics, including basic routing, route parameters, nested routes, redirects, navigation components, programmatic navigation, route guards, code splitting, error handling, lazy loading, and SEO considerations for SPAs. 🔀🔒🚦 With these techniques in your toolkit, you can build powerful and optimized single-page applications in React. Let’s dive in and unlock the full potential of routing in React! 💪🔥