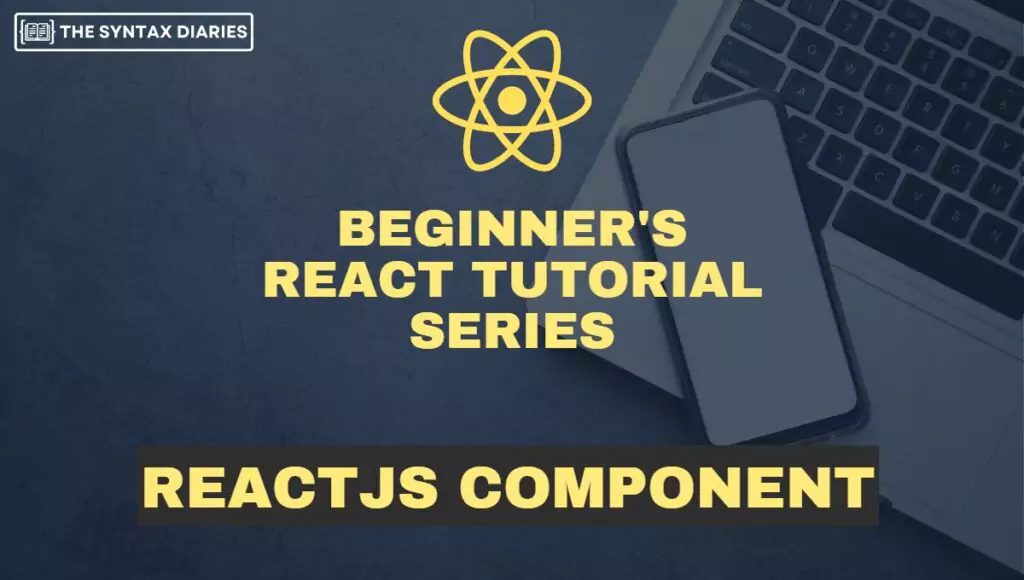
Welcome to our exciting guide on ReactJS Components and Props! š In this blog post, we will explore the essential concepts of ReactJS components and how they empower developers to build interactive web applications with ease. Whether youāre a beginner or an experienced ReactJS enthusiast, this comprehensive guide will provide you with valuable insights to level up your ReactJS development skills. Letās dive in! šŖš
1. What are ReactJS Components? š¤
ReactJS components are the building blocks of a React application. They encapsulate reusable pieces of UI, making it easier to develop and maintain complex user interfaces. ReactJS components allow developers to break down the UI into smaller, self-contained sections, each responsible for specific functionality. This modular approach promotes code reusability, improves maintainability, and enhances the overall development experience. Letās explore the different types of ReactJS components. š
2. Types of ReactJS Components
2.1. Functional Components
Functional components are the simplest type of ReactJS components. They are JavaScript functions that accept props as input and return React elements to describe the UI. Functional components are lightweight, easy to understand, and encourage a functional programming style. They are the preferred choice when you only need to render UI based on props and donāt require internal state or lifecycle methods.
Hereās an example of a functional component:
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
// Usage
<Greeting name="John" />
<Greeting name="Jane" />
In this example, the Greeting
component accepts a name
prop and renders a personalized greeting. You can use the Greeting
component multiple times with different names to display customized greetings.
2.2. Class Components
Class components are the traditional way of creating ReactJS components. They are JavaScript classes that extend the React.Component
class. Class components have access to powerful features such as internal state, lifecycle methods, and class inheritance. While functional components are recommended for most scenarios, class components are still used in certain cases, especially when dealing with complex state management or interacting with external APIs.
Hereās an example of a class component:
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
increment() {
this.setState({ count: this.state.count + 1 });
}
render() {
return (
<p>Count: {this.state.count}</p>
<button onClick={() => this.increment()}>Increment</button>
);
}
}
// Usage
<Counter />
In this example, the Counter
component maintains a count value in its state. Clicking the āIncrementā button updates the count and triggers a
re-render of the component.
2.3. React Hooks ā
React Hooks are a recent addition to ReactJS, introduced in React 16.8. They provide a way to use state and other React features in functional components. Hooks enable ReactJS developers to write reusable logic and manage stateful behavior without using class components. They offer a more concise and intuitive way to handle state, side effects, and lifecycle events in functional components.
Hereās an example of a functional component using React Hooks:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
);
}
// Usage
<Counter />
In this example, the Counter
component uses the useState
hook to manage the count state. Clicking the āIncrementā button updates the count and triggers a re-render.
3. Creating and Using ReactJS Components and Props
3.1. JSX Syntax āØ
JSX (JavaScript XML) is a syntax extension for JavaScript that allows you to write HTML-like code within JavaScript. It is the recommended way to define React elements and components. JSX combines the power of JavaScript with the expressiveness of HTML, making it easier to describe the structure and appearance of your UI.
function MyComponent() {
return (
<h1>Hello, ReactJS!</h1>
<p>This is a JSX component.</p>
);
}
In this example, the MyComponent
function returns JSX code that represents a div element containing a heading and a paragraph. JSX helps you write concise and readable code.
3.2. ReactJS Component and Props š
Props (short for properties) are a fundamental concept in ReactJS. They allow you to pass data from a parent component to a child component. Props are read-only and should not be modified by the child component. They enable components to be reusable and customizable, as different instances of the same component can receive different data through props.
Hereās an example of a component that receives props:
function Greeting(props) {<br />
return </p>
<h1>Hello, {props.name}!</h1>
<p>;<br />
}<br />
// Usage<br />
<Greeting name="John" /><br />
<Greeting name="Jane" />
In this example, the Greeting
component accepts a name
prop and renders a personalized greeting based on the value passed in.
3.3. State š¦
State represents the internal data of a component. It allows components to keep track of information that can change over time. State is managed using the useState
hook (for functional components) or by extending the React.Component
class (for class components). By updating state, components can trigger re-rendering and reflect the changes in the UI.
Hereās an example of a component using state:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
);
}
// Usage
<Counter />
In this example, the Counter
component maintains a count value in its state. Clicking the āIncrementā button updates the count and triggers a re-render.
3.4. Lifecycle Methods ā
Lifecycle methods are special methods provided by ReactJS that allow you to hook into different stages of a componentās lifecycle. Class components have various lifecycle methods, such as componentDidMount
, componentDidUpdate
, and componentWillUnmount
, which provide opportunities to perform actions at specific points during the componentās existence. With the introduction of React Hooks, functional components can also use lifecycle functionality using the useEffect
hook.
Hereās an example of a class component using lifecycle methods:
class MyComponent extends React.Component {
componentDidMount() {
// Perform initialization logic here
console.log("Component mounted")
}
componentDidUpdate(prevProps, prevState) {
// Perform update logic here
console.log("Component updated")
}
componentWillUnmount() {
// Perform cleanup logic here
console.log("Component unmounted")
}
render() {
return <h1>Hello, ReactJS!</h1>
}
}
// Usage
;<MyComponent />
In this example, the MyComponent
class component logs messages to the console at different lifecycle stages.
For functional components, you can achieve similar functionality using the useEffect
hook:
import React, { useEffect } from "react"
function MyComponent() {
useEffect(() => {
// Perform initialization logic here
console.log("Component mounted")
return () => {
// Perform cleanup logic here
console.log("Component unmounted")
}
}, [])
return <h1>Hello, ReactJS!</h1>
}
// Usage
;<MyComponent />
In this example, the useEffect
hook is used to perform initialization logic when the component mounts and cleanup logic when it unmounts.
4. Reusability and Composition
ReactJS components are designed to be reusable and composable. Reusability means that components can be used in different parts of the application, reducing duplication and promoting code efficiency. Composition refers to the ability to combine multiple components to create more complex UI structures. ReactJS encourages the composition of smaller components to build larger and more sophisticated applications, enhancing code maintainability and scalability.
5. Best Practices for ReactJS Components and Props
To make the most out of ReactJS components, consider following these best practices:
- Keep components focused and single-purpose.
- Use functional components whenever possible.
- Avoid excessive nesting of components.
- Use meaningful prop names for better readability.
- Optimize component rendering using
React.memo
andshouldComponentUpdate
. - Follow naming conventions for component files and directories.
- Write clear and concise documentation for your components.
6. Conclusion
In this blog post, we explored the world of ReactJS components and Props and their significance in building interactive web applications. We discussed functional components, class components, and React Hooks, each serving different purposes in ReactJS development. We also covered JSX syntax, props, state, and lifecycle methods to give you a well-rounded understanding of ReactJS components.
Remember to apply best practices and leverage the power of component reusability and composition when developing your ReactJS applications. Stay curious, experiment, and keep learning to master the art of building dynamic and engaging user interfaces with ReactJS!
If you found this guide helpful, donāt hesitate to share it with your fellow React developers on social media and other platforms. Letās spread the ReactJS love! ā¤ļøš
If you have any questions or need further assistance, feel free to leave a comment below. Happy coding, React developer! š»š„