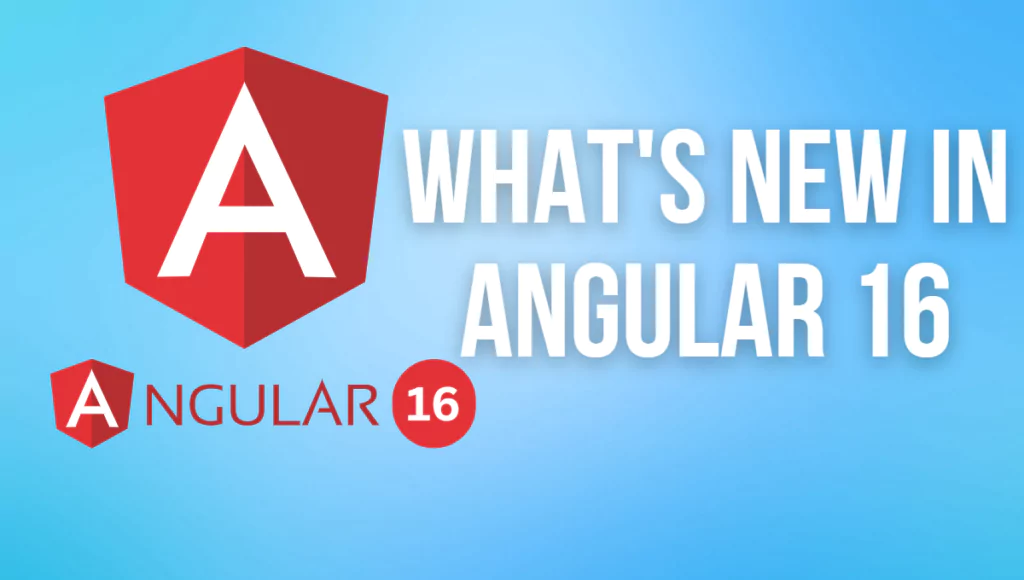
Angular 16 is the latest version of the Angular framework, released on May 3, 2023. This release brings a number of new features and improvements, making Angular a more powerful and versatile platform for building web applications. If you’re looking to learn Angular 16 and take advantage of its new capabilities, this Angular 16 tutorial will guide you through the process.
Are you new to Angular? Enhance your skills with my step-by-step Angular tutorials:
👉 Part 1: [Click here] to read the First Part and begin your Angular journey.
👉 Part 2: [Click here] to read the Second Part and dive deeper into Angular concepts and techniques.
For more information and external resources, check out the following helpful links:
Official documentation: The official documentation for Angular.
Community forum: Engage with the Angular community and get support.
Angular tutorials on YouTube: Explore Angular tutorials on YouTube.
In this blog post, I’ll take a look at some of the new features in Angular 16. I’ll also share some tips on how to upgrade your Angular applications to Angular 16.
So, if you’re an Angular developer, or if you’re thinking about using Angular, then this blog post is for you!
Let’s get started!
Rethinking Reactivity
Angular 16 introduces a new reactivity model, which is based on signals. Signals are a lightweight way to notify Angular when data changes, which can improve performance and reduce complexity.
In previous versions of Angular, reactivity was based on zones. Zones are a way to intercept and handle asynchronous events, such as network requests and DOM changes. However, zones can add overhead, and they can make it difficult to reason about the flow of control in an Angular application.
Signals are a more lightweight way to handle asynchronous events. Signals are created by the Angular compiler, and they are attached to data properties. When the value of a data property changes, the signal is notified. Angular can then react to the change by updating the view.
The use of signals can improve performance by reducing the number of times that Angular needs to check for changes in data properties. Signals can also reduce complexity by making it easier to reason about the flow of control in an Angular application.
To use the new reactivity model in Angular 16, you need to import the rxjs/reactivity
package. Once you have imported the package, you can use the BehaviorSubject
class to create a reactive value. The BehaviorSubject
class is a special type of observable that always has a current value. You can use the subscribe
method to subscribe to a BehaviorSubject
and receive notifications whenever the value changes.
For example, the following code creates a reactive value called counter
and subscribes to it:
import { BehaviorSubject } from "rxjs/reactivity"
const counter = new BehaviorSubject(0)
counter.subscribe((value) => {
console.log(value)
})
The console.log
statement will print the current value of counter
whenever it changes.
Angular 16 Signals
Angular Signals is a new library that provides a way to define reactive values and expose their dependencies. This can be used to improve the performance and maintainability of Angular applications.
Reactive values are values that can be observed for changes. When the value of a reactive value changes, all of the observers are notified. This can be used to implement features such as real-time updates and data binding.
Dependency injection is a technique for injecting dependencies into Angular Components. This can help to improve the maintainability of Angular applications by making it easier to unit test components.
Angular Signals provides a way to combine reactive values and Angular Dependency Injection. This can be used to create components that are more performant and easier to maintain.
To use Angular Signals in Angular 16, you need to import the angular/common/signal
package. Once you have imported the package, you can use the Signal
class to create a signal. The Signal
class is a special type of observable that can be used to notify Angular when a value changes.
For example, the following code creates a signal called counter
and subscribes to it:
import { Signal } from "angular/common/signal"
const counter = new Signal(0)
counter.subscribe((value) => {
console.log(value)
})
The console.log
statement will print the current value of counter
whenever it changes.
Improved Interoperability with Angular 16 RxJS
Angular 16 improves interoperability with RxJS, a popular library for reactive programming. This makes it easier to use RxJS features in Angular applications.
RxJS is a library that provides a number of features for reactive programmings, such as Observables, Subjects, and Operators. Observables are a way to represent asynchronous events, such as network requests and DOM changes. Subjects are a way to broadcast data to multiple subscribers. Operators are a way to manipulate Observables.
Angular 16 includes a number of features that make it easier to use RxJS in Angular applications. For example, Angular 16 includes a number of built-in operators that can be used to manipulate Observables. Angular 16 also includes a number of built-in Subjects that can be used to broadcast data to multiple subscribers.
The improved interoperability with RxJS makes it easier to use RxJS features in Angular applications. This can help to improve the performance and maintainability of Angular applications.
Angular 16 improves interoperability with RxJS by providing a number of new features. For example, Angular 16 includes a new pipe called async
that can be used to convert an Observable into an array of values. Angular 16 also includes a new pipe called takeUntil
that can be used to stop an Observable from emitting values after a certain condition is met.
For example, the following code uses the async
pipe to convert an Observable into an array of values:
const items = [{ name: "A" }, { name: "B" }, { name: "C" }]
const observable = Observable.from(items)
const array = observable | async
console.log(array) // ['A', 'B', 'C']
The console.log
statement will print the array of values that was emitted by the Observable.
Improved Support for Server-Side Rendering (SSR) in Angular 16
Angular 16 includes improved support for server-side rendering (SSR). SSR is a technique for rendering an Angular application on the server before it is sent to the client. This can improve the performance of Angular applications by making them load faster.
In previous versions of Angular, SSR was a complex process. However, Angular 16 makes SSR easier to use by providing a number of improvements. For example, Angular 16 includes a new compiler that can be used to generate server-side code. Angular 16 also includes a new router that can be used to route between pages on the server.
The improved support for SSR makes it easier to build Angular applications that load faster. This can improve the user experience and make Angular applications more appealing to users.
To use the improved support for SSR in Angular 16, you need to enable the serverRendering
option in the angular.json
file. Once you have enabled the serverRendering
option, you can use the ng serve
command to build and serve your application in both the browser and on the server.
For example, the following code enables the serverRendering
option in the angular.json
file:
"app": {
"architect": {
"build": {
"options": {
"serverRendering": true
}
}
}
}
The following code uses the ng serve
command to build and serve the application in both the browser and on the server.
The application will be built and served on the server. You can then access the application in the browser by opening the URL http://localhost:4200
.
Improved Hydration Process
Angular 16 also includes an improved hydration process. Hydration is the process of converting a server-rendered application into a client-side application. This can improve the performance and user experience of Angular applications.
In previous versions of Angular, the hydration process was a complex process. However, Angular 16 makes the hydration process easier to use by providing a number of improvements. For example, Angular 16 includes a new compiler that can be used to generate client-side code. Angular 16 also includes a new router that can be used to route between pages on the client.
The improved hydration process makes it easier to build Angular applications that load faster and have a better user experience.
To use the improved hydration process in Angular 16, you need to enable the enableProdMode
option in the angular.json
file. Once you have enabled the enableProdMode
option, you can use the ng build
command to build your application in production mode.
The ng build
command will generate a bundle.js
file that contains the compiled code for your application. You can then deploy the bundle.js
file to a web server.
When a user visits the web server, the browser will download the bundle.js
file and use it to render the application. The improved hydration process will ensure that the application is rendered quickly and efficiently.
Improved Tooling Experience for Standalone Components, Pipes, and Directives
Angular 16 also improves the tooling experience for standalone components, pipes, and directives. This makes it easier to develop and test these features.
In previous versions of Angular, it was difficult to develop and test standalone components, pipes, and directives. However, Angular 16 makes it easier to do this by providing a number of improvements. For example, Angular 16 includes a new compiler that can be used to generate code for standalone components, pipes, and directives. Angular 16 also includes a new test runner that can be used to test standalone components, pipes, and directives.
The improved tooling experience for standalone components, pipes, and directives makes it easier to develop and test these features. This can help to improve the quality of Angular applications.
Advancing Developer Tooling in Angular 16
Angular 16 includes a number of improvements to the Angular CLI, the official development tool for Angular. These improvements make it easier to develop and build Angular applications.
For example, Angular 16 includes a new command that can be used to generate a new Angular application. Angular 16 also includes a new command that can be used to upgrade an existing Angular application to the latest version.
The improved developer tooling in Angular 16 makes it easier to develop and build Angular applications. This can help to improve the productivity of Angular developers.
The Angular CLI in Angular 16 includes a number of new commands that can be used to improve the developer experience. For example, the ng generate component
command can now be used to generate a standalone component. The ng test
command can now be used to run unit tests for standalone components.
For example, the following code generates a standalone component called CounterComponent
:
ng generate component CounterComponent
The ng test
command can then be used to run unit tests for the CounterComponent
:
ng test CounterComponent
The ng test
command can then be used to run unit tests for the CounterComponent
:
Better Unit Testing using Jest and Web Test Runner
Angular 16 includes support for Jest, a popular unit testing framework. This makes it easier to write and run unit tests for Angular applications.
Jest is a fast and flexible unit testing framework. It is easy to learn and use, and it can be used to test Angular applications in a variety of ways.
The support for Jest in Angular 16 makes it easier to write and run unit tests for Angular applications. This can help to improve the quality of Angular applications.
To use Jest to test an Angular application, you need to install the Jest package. Once you have installed the package, you can use the ng test
command to run unit tests for your application.
For example, the following code installs the Jest package:
ng test CounterComponent
The following code runs unit tests for an Angular application:
ng test
Autocomplete Imports within the HTML Templates
Angular 16 includes support for autocomplete imports within the HTML templates. This makes it easier to import components, pipes, and directives into the HTML templates.
The autocomplete imports feature in Angular 16 makes it easier to write HTML templates. This can help to improve the productivity of Angular developers.
For example, the following code imports the CounterComponent
component into the HTML template:
<counter-component></counter-component>
The Angular compiler will automatically import the CounterComponent
component into the application.
Conclusion
Angular 16 is a major release that includes a number of new features and improvements. These features are designed to make Angular development faster, easier, and more powerful.
In this blog post, we have taken a closer look at some of the key new features in Angular 16. We have discussed the new reactivity model, Angular Signals, improved interoperability with RxJS, improved support for server-side rendering, improved hydration process, improved tooling experience for standalone components, pipes, and directives, advancing developer tooling, better unit testing using Jest and Web Test Runner, and autocomplete imports within the HTML templates.
We hope that this blog post has been helpful. If you have any questions, please feel free to ask.
Happy coding! 😄🚀