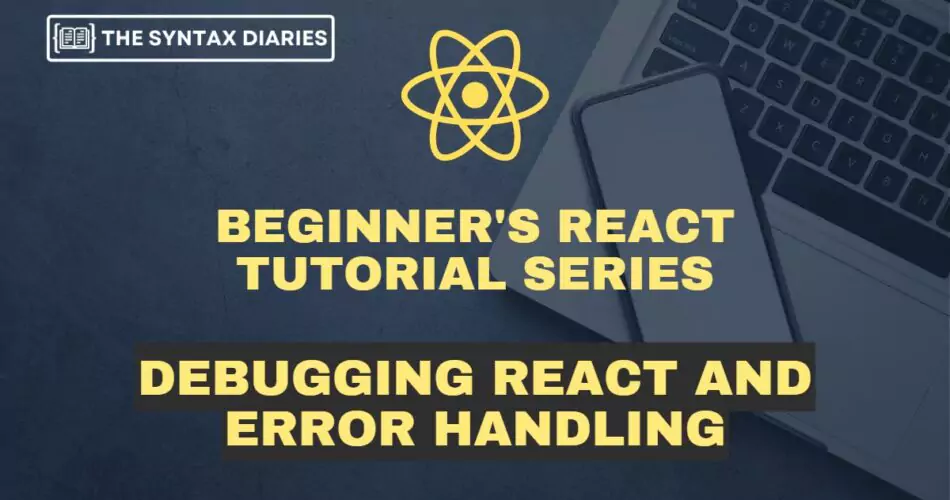
Debugging React and Error Handling - Tools and Techniques
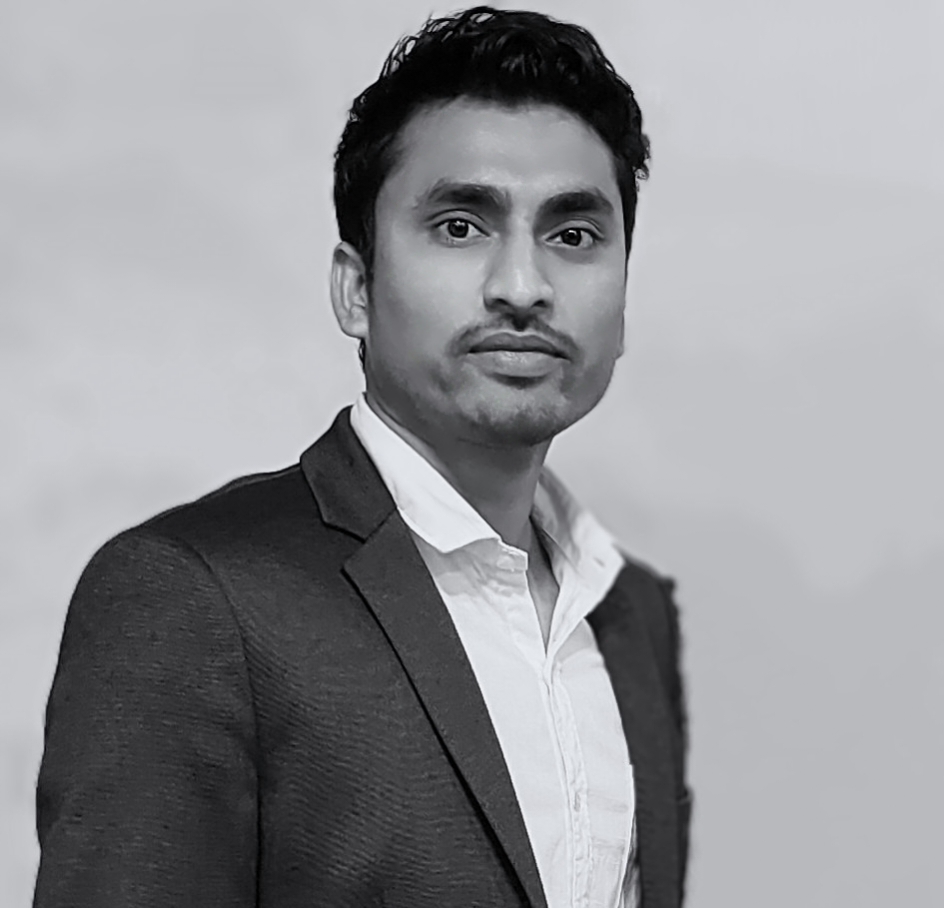
By Amaresh Adak
Debugging React applications and effectively handling errors are the key topics we will explore in this article. React has gained immense popularity as a JavaScript library for building user interfaces due to its component-based architecture and efficient rendering. However, like any other software development framework, React applications are prone to errors. In this article, we will explore the various techniques and best practices for handling errors and debugging React applications effectively. 🚀
If you are interested in learning about react components, I recommend checking out my blog post dedicated to this topic. You can read the post by clicking here.
If you want to learn more about React State and Props, I have a blog post that explains everything in simple terms. Just click here to read the post and dive into the topic.
Common Types of Errors in React 🐛
Before diving into error handling techniques, it’s essential to understand the common types of errors that can occur in React applications. Errors can be categorized into distinct groups as follows:
Syntax Errors 💥
Syntax errors occur when there are mistakes in the code that violate the rules of the JavaScript language. These errors are usually detected during the compilation phase and prevent the code from running.
Runtime Errors 🚩
Runtime errors, also known as exceptions, are unexpected events that can occur during the execution of a program. They can be caused by various factors, such as incorrect data manipulation, invalid function calls, or unexpected behavior of external dependencies.
Logic Errors 🧩
Logic errors are the most elusive type of errors as they don’t cause the code to break or throw exceptions. Instead, they result in incorrect or unexpected behavior of the application. Identifying and fixing logic errors requires careful analysis and debugging.
Built-in Error Boundary in React 🛡️
React provides a built-in mechanism called “Error Boundary” to handle errors that occur within the component tree. An Error Boundary is a React component that wraps around other components and catches any errors thrown by its children.
To define an Error Boundary in React, you can use a functional component and the useErrorBoundary
hook provided by popular error boundary libraries such as react-error-boundary
. 🪄
Here’s an example of an Error Boundary component using the react-error-boundary
library:
import { useErrorBoundary } from "react-error-boundary"
const ErrorBoundary = () => {
const { ErrorBoundary, didCatch, error } = useErrorBoundary()
if (didCatch) {
// Render fallback UI when an error occurs
return <div>Oops! Something went wrong.</div>
}
return (
<ErrorBoundary>
<YourComponent />
</ErrorBoundary>
)
}
export default ErrorBoundary
Using the useErrorBoundary
hook, you can easily define an Error Boundary and handle errors within your functional components. 🎯
To learn more about the react-error-boundary
npm package, you can find detailed information by clicking here.
Handling Errors in React Components ⚙️
Apart from using Error Boundaries, there are other techniques to handle errors within individual React components. One common approach is to use try-catch statements to catch and handle errors within specific code blocks.
Here’s an example of handling errors with a try-catch statement in a functional component:
import React from "react"
const MyComponent = () => {
try {
// Code that may throw an error
} catch (error) {
// Handle the error gracefully
}
return <div>My Component</div>
}
export default MyComponent
In this example, any errors thrown within the try block will be caught by the catch block, allowing you to handle them appropriately.
Another technique involves conditional rendering, where you check for error conditions and render alternative UI components or error messages accordingly.
import React from "react"
const MyComponent = () => {
const [error, setError] = React.useState(null)
if (error) {
return <div>Error: {error.message}</div>
}
// Code that may throw an error
return <div>My Component</div>
}
export default MyComponent
In this example, the component checks for the presence of an error state and renders an error message if it exists. 🌈
Logging and Debugging in React 🐞
When it comes to debugging React applications, logging plays a crucial role in identifying and fixing errors. React provides various logging options, including using console logs to output information to the browser’s console.
import React from 'react';
const MyComponent = () => {
const MyComponent = () => {
const handleClick = () => {
console.log('Button clicked! 👆');
};
return <button onClick={handleClick}>Click Me</button>;
};
Additionally, React DevTools is a powerful browser extension that allows developers to inspect and debug React component hierarchies, props, and state. It provides a comprehensive view of the application’s components, making it easier to identify and fix issues. 🛠️
For more advanced debugging, developers can leverage Chrome Developer Tools, which provides a wide range of debugging features specific to JavaScript and React applications. 🔍
Best Practices for Error Handling and Debugging in React 🌟
To ensure efficient error handling and debugging in React applications, it is essential to follow best practices. Here are some recommendations:
- Consistent Error Messaging 📝: Provide clear and informative error messages to help users and developers understand the issue.
- Properly Handling Asynchronous Errors ⏳: When dealing with asynchronous operations, handle errors appropriately and communicate them to the user effectively.
- Testing Error Scenarios 🧪: Create test cases that cover various error scenarios to identify and fix issues before deploying the application.
Conclusion 🎉
Error handling and debugging are critical aspects of React development. By understanding different types of errors, utilizing Error Boundaries, adopting proper error handling techniques, leveraging debugging tools like breakpoints, and following best practices, developers can build robust and reliable React applications. 🛠️
Incorporate the best practices mentioned in this article to streamline the error handling and debugging process, ensuring a smooth user experience. Remember to remove breakpoints once you have finished debugging to ensure your code runs without interruption in production.
With the right approach to error handling and debugging, you can create React applications that are more resilient and provide an improved user experience. 🚀
Debugging React and Error Handling FAQs ❔
Q1: How do I find the source of a React error? ❓
To find the source of a React error, you can check the error message and the associated stack trace. The stack trace provides information about the sequence of function calls that led to the error. By examining the stack trace, you can identify the specific component or function responsible for the error.
Q2: Is it possible to utilize try-catch blocks within React components?✅
Yes, you can use try-catch blocks in React components to catch and handle errors within specific code blocks. However, it’s important to note that try-catch blocks should be used sparingly and for exceptional cases, as they may affect the performance of your application.
Q3: What is the purpose of an Error Boundary in React? 🛡️
An “Error Boundary” in React serves as a specialized component that effectively captures and handles JavaScript errors within its child component tree. When an error occurs, it not only logs the error but also gracefully presents a fallback user interface instead of displaying the crashed component tree. It helps prevent the entire application from crashing due to errors in specific components.
Q4: How can I debugging React components using React DevTools? 🛠️
To debug React components using React DevTools, install the React DevTools browser extension. After successful installation, you can effortlessly access the React DevTools panel within your browser’s developer tools. This intuitive panel presents a comprehensive tree view of the React component hierarchy, empowering you to meticulously examine and troubleshoot individual components, their props, and state.
Q5: Are there any tools for automated testing of error scenarios in React? 🧪
Yes, there are several tools available for automated testing of error scenarios in React. Some popular options include Jest, React Testing Library, and Cypress. These testing frameworks provide utilities and methods to simulate error conditions and assert the expected behavior of the application in response to those errors.