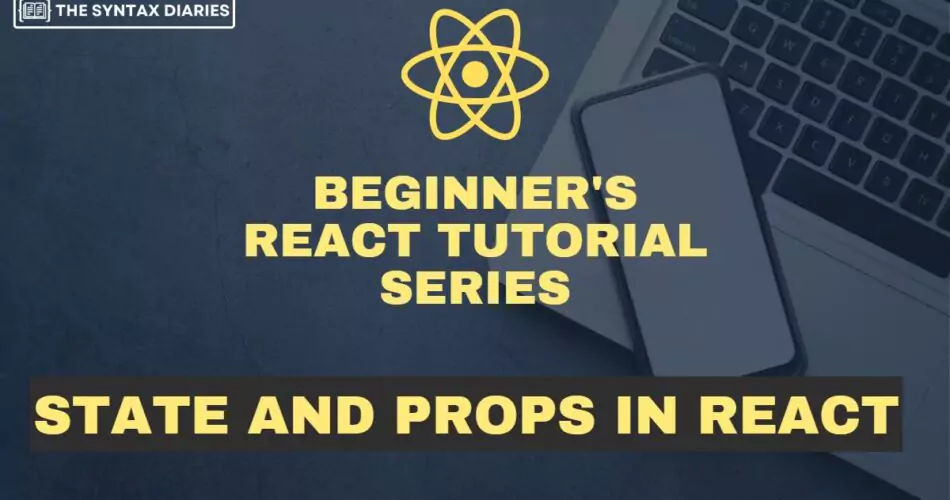
React State Management using React Props: Strategies and Techniques
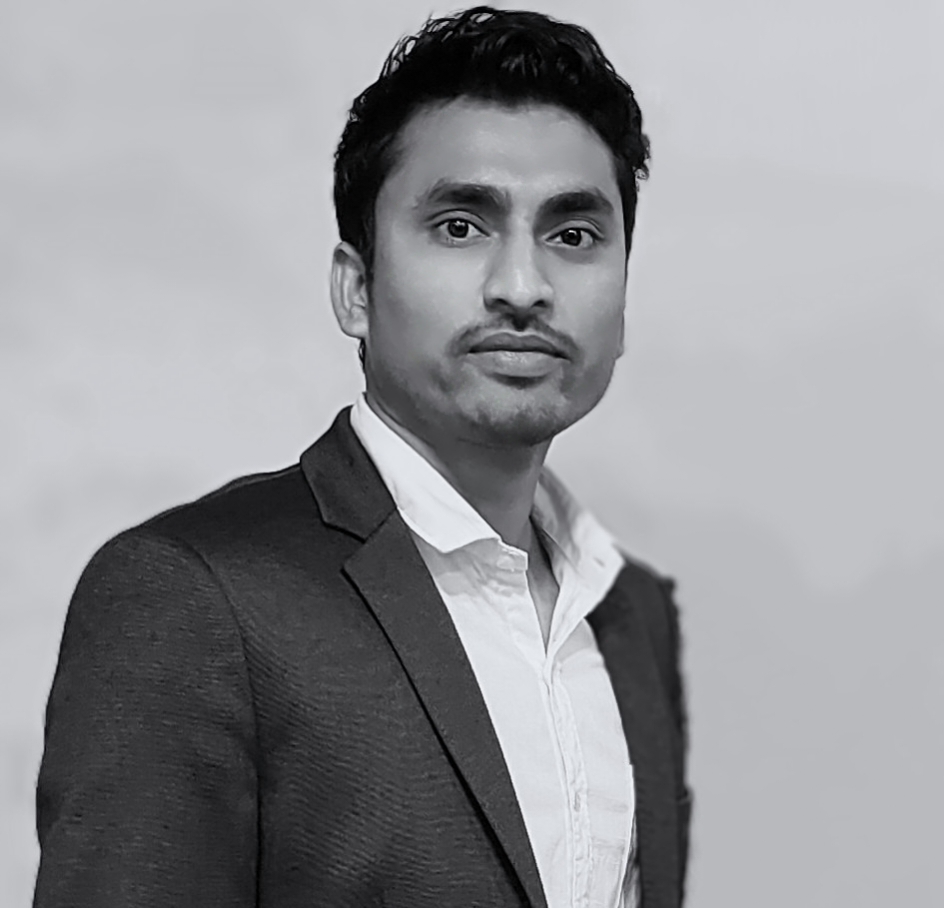
By Amaresh Adak
When it comes to building dynamic and interactive web applications, React has emerged as one of the most popular JavaScript libraries. React is a component-based architecture that allows developers to create reusable UI components, resulting in efficient and maintainable code. Understanding how to manage data within React components is essential. This is where the concepts of react state management and react props come into play. 🎯
In this article, we will explore the basics of state and react props in React. We’ll cover their definitions, differences, and use cases, providing you with a solid foundation to build upon. Whether you’re new to React or looking to refresh your knowledge, this guide will help you gain a deeper understanding of state and props and their significance in React development. 📚
If you are interested in learning about components, I recommend checking out my blog post dedicated to this topic. You can read the post by clicking here.
React State Management and Props: A Brief Overview
Before we dive deeper, let’s start with a brief overview of what state and props are in the context of React. 🌟
What is State in React? 📦
State is a built-in feature in React that allows components to store and manage data internally. It represents the mutable properties of a component that can be updated and affect the component’s rendering. State enables components to be dynamic and responsive to user interactions, API responses, or any other events that trigger a change in data. 🔄
What are the React Props? 🎁
On the other hand, props (short for properties) are read-only attributes passed from a parent component to its child component. They serve as a mechanism for components to communicate and share information. react Props are used to pass data and configuration to child components, allowing them to render dynamically based on the received values. 📤
The Role of React State Management in React Components
To better understand the role of state, let’s explore its significance within React components. 🌈
Creating a Functional Component
In React, we have two types of components: class components and functional components. In this article, we will explore the use of functional components in ReactJS. Functional components are the preferred approach for the latest versions of ReactJS, as they are more concise, easier to test, and more performant than class components. Let’s create a simple functional component to get started: 🏗️
import React from "react"
const MyComponent = () => {
// Component logic goes here
return <div>Hello, React!</div>
}
export default MyComponent
Functional components are declared using arrow functions and return JSX elements that define the component’s UI. State management in functional components is achieved using React hooks, with the useState
hook being the primary one for managing state. ⚛️
Using React State Management in a Functional Component
To introduce state in our functional component, we import the useState
hook from the react
package and use it within the component body. Let’s update our example to include state: 🔥
import React, { useState } from "react"
const MyComponent = () => {
const [count, setCount] = useState(0)
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
)
}
export default MyComponent
In the above code, we introduce a state variable called count
and a corresponding function called setCount
using the useState
hook. The initial value count
is set to 0. By calling setCount
with a new value, we can update the state and trigger a re-render of the component. 🔄
Updating State and Re-rendering Components
When the user clicks the “Increment” button, the onClick
event triggers the anonymous arrow function that updates the count
state by incrementing it by 1. As a result, the component re-renders, displaying the updated value of count
. 🔄✨
This simple example demonstrates the basic usage of state in a functional component. A state can store various types of data, such as numbers, strings, booleans, arrays, or even objects. It enables components to react to user input, manage internal data, and update the UI accordingly. 💪
Passing Data with Props in React Components
Now that we have a good understanding of state, let’s shift our focus to props and how they facilitate communication between components. 🗣️
Creating a Parent Component
In React, components are organized in a tree structure. A parent component can pass data and configuration to its child component through react props. Let’s create a parent component and a child component to demonstrate this concept: 👪
import React from "react"
import ChildComponent from "./ChildComponent"
const ParentComponent = () => {
const greeting = "Hello, from the parent component!"
return (
<div>
<ChildComponent greeting={greeting} />
</div>
)
}
export default ParentComponent
In this example, we define a parent component called ParentComponent
that renders a child component called ChildComponent
. We pass a prop called greeting
to the child component, which contains the value 'Hello, from the parent component!'
. 🎁
Accessing React Props in a Child Component
To access and utilize the props passed from the parent component, we need to define the corresponding prop in the child component’s function signature. Let’s update our code to incorporate the child component and access the prop: 📤
import React from "react"
const ChildComponent = (props) => {
return <div>{props.greeting}</div>
}
export default ChildComponent
In the ChildComponent
function, we define props
as the function parameter to receive the props object passed from the parent component. We can then access the greeting
prop using props.greeting
the component’s UI. 👶
Dynamic Rendering with React Props
By leveraging props, we can dynamically render components based on the data and configuration provided by their parent components. The parent component can pass different values for props, allowing child components to adapt and render accordingly. 🎨✨
Props are read-only, meaning child components cannot modify their props directly. If a child component needs to update its behavior based on a prop, the parent component should pass a new prop value to trigger a re-render of the child component. ⚡
For more details about state management, you can click here to check out the official documentation on React state management. Here is the link to the official document: https://react.dev/learn/managing-state.
FAQs about React State Management and React Props
1. Q: What is the difference between state and props in React?
A: The key difference lies in their mutability. State represents mutable data within a component that can be updated, while props are read-only attributes passed from parent components. 🔄🎁
2. Q: Can functional components have state in React?
A: Yes, functional components can manage state using React hooks. The useState hook allows functional components to declare and update state variables. ⚛️🔥
3. Q: How do I pass props to a child component in React?
A. To pass props to a child component, simply include the desired prop and its value within the JSX markup when rendering the child component from the parent component. 📤🎨
4. Q: Can a parent component access the state of its child component in React?
A. No, React follows a unidirectional data flow. Child components receive data from parent components through props, but parent components cannot directly access the state of their child components. 🚫🔄
5. Q: Can I pass functions as props in React?
A. Yes, functions can be passed as props in React. This enables parent components to provide callback functions to child components, allowing child components to communicate back to their parents. 🗣️🎁
6. Q: When should I use state and when should I use props in React?
A. Use state when you need to manage mutable data within a component and props when you want to pass data from a parent component to its child component. 📦🌈
Conclusion
In this article, we explored the basics of state and props in React. The state allows components to manage internal data and render dynamically, while props facilitate communication between parent and child components. Understanding how to effectively utilize state and props is essential for building robust and interactive React applications. 💻🚀
We covered the fundamental concepts of state and props, including their definitions, usage in functional components, and how they enable dynamic rendering and component communication. By mastering these concepts, you’ll be well-equipped to leverage state and props effectively in your React projects. 🎓✨
Now that you have a solid understanding of the basics, it’s time to dive deeper and explore the countless possibilities and advanced techniques that state and props offer in React development. Happy coding! 💡💪