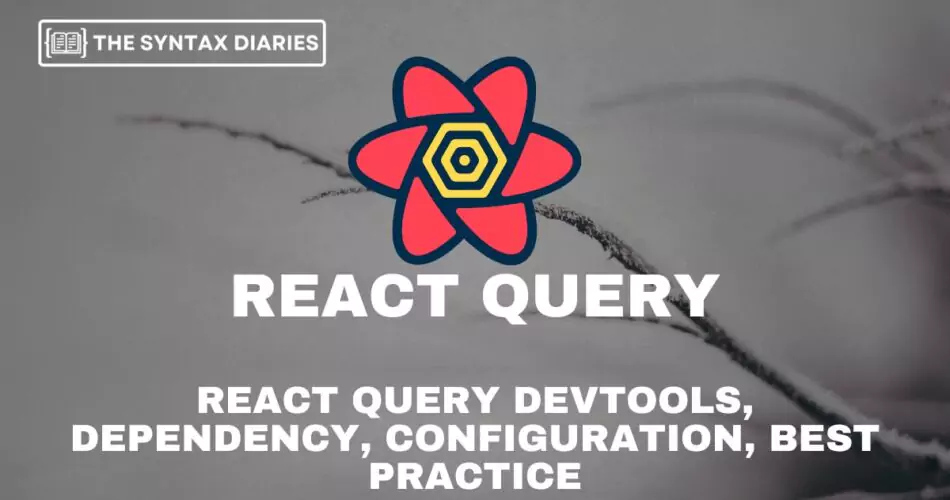
React Query Devtools, Dependency, Configuration, Best Practice
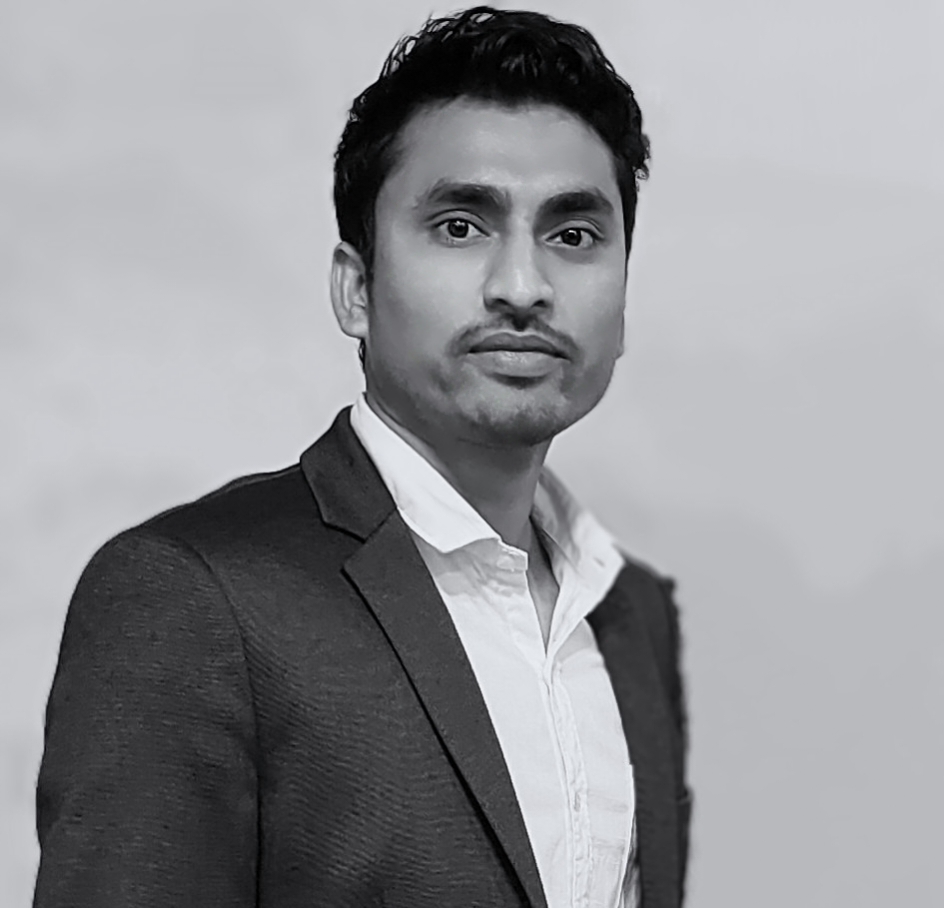
By Amaresh Adak
In the previous blog post, you were introduced to React Query and its primary hooks, useQuery and useMutation, which enable data fetching and updates from an API. However, React Query offers more advanced features to optimize data fetching. These include using React Query Devtools, React Query Cache, React Query Refetch, configuring the library, and setting dependencies for more efficient data management.
In this comprehensive blog post, you’ll gain valuable insights on managing query dependencies, configuring global settings and providers, harnessing advanced React Query devtools and debugging features, seamlessly integrating React Query with your React applications, and adhering to best practices for optimized data fetching. 🚀
By the time you finish reading this article, you’ll be a pro at utilizing React Query for various data fetching scenarios, thanks to the abundance of transition words and in-depth explanations provided. 💯
1. Managing Query Dependencies
Query dependencies play a crucial role in ensuring that the data displayed in our application is always up-to-date. React Query provides mechanisms to manage query dependencies and automatically update queries when the data they depend on changes. Let’s explore these features.
useQuery
with Dependencies
1.1 Using In some cases, the data displayed by a query might depend on other data. For example, consider a todo list where each todo item belongs to a specific category. When we edit a todo item’s title, it’s essential to update the corresponding category list to reflect the changes. React Query allows us to specify query dependencies using the queryKey
option. Let’s see an example:
import { useQuery } from "react-query"
const CategoryTodoList = ({ category }) => {
// Use 'category' as a dependency to update the query when it changes
const { data } = useQuery(["categoryTodos", category.id], fetchCategoryTodos)
return (
<div>
<p>{category.name}</p>
<ul>
{data.map((todo) => (
<li key={todo.id}>{todo.title}</li>
))}
</ul>
</div>
)
}
In this code snippet, we use the category.id
as part of the queryKey
to create a unique identifier for the ‘categoryTodos’ query. This ensures that when the category
prop changes, the ‘categoryTodos’ query will automatically update with the latest data.
1.2 Manual React Query Refetch
There might be scenarios where we want to manually trigger a react query refetch based on certain user actions or events. React Query provides the refetch
function to achieve this. Let’s see an example:
import { useQuery } from "react-query"
const TodosWithRefetch = () => {
const { data, refetch } = useQuery("todos", fetchTodos)
const handleButtonClick = () => {
// Manually trigger a refetch of the 'todos' query
refetch()
}
return (
<div>
<ul>
{data.map((todo) => (
<li key={todo.id}>{todo.title}</li>
))}
</ul>
<button onClick={handleButtonClick}>Refresh Todos</button>
</div>
)
}
In this code snippet, we use the refetch
function to manually trigger a refetch of the ‘todos’ query when the “Refresh Todos” button is clicked. This allows us to fetch the latest todo data from the server without needing to remount the component or wait for the automatic refetch interval.
2. Global Configuration and Providers ⚙️
React Query allows us to configure and customize its behavior globally across our application. We can use a QueryClientProvider to provide the global configuration to all components that use React Query hooks. Let’s see how to set up global structure:
import { QueryClient, QueryClientProvider } from "react-query"
// Create a new instance of QueryClient
const queryClient = new QueryClient({
defaultOptions: {
queries: {
refetchOnWindowFocus: false,
staleTime: 30000, // 30 seconds
},
mutations: {
onError: (error) => {
console.error("Mutation error:", error)
},
},
},
})
const App = () => {
return (
// Wrap the entire application with QueryClientProvider
<QueryClientProvider client={queryClient}>
{/* Your application components */}
</QueryClientProvider>
)
}
In this code snippet, we create a new instance of QueryClient
and provide default options for queries and mutations. The refetchOnWindowFocus
option disables automatic react query refetch when the window regains focus, ensuring a better user experience. The staleTime
option sets the time after which data is considered stale and eligible for refetching. Additionally, we define a custom onError
function for mutations to handle mutation errors globally.
3. Advanced React Query Devtools and Debugging 🛠️
React Query offers powerful devtools that help us inspect, debug, and optimize our queries and mutations. These devtools provide valuable insights into the status of queries, their dependencies, and performance metrics. Let’s see how to utilize these react query devtools:
import { ReactQueryDevtools } from "react-query/devtools"
import { QueryClient, QueryClientProvider } from "react-query"
const queryClient = new QueryClient()
const App = () => {
return (
<QueryClientProvider client={queryClient}>
{/* Your application components */}
<ReactQueryDevtools />
</QueryClientProvider>
)
}
In this code snippet, we import ReactQueryDevtools
from the react-query/devtools
package and add it to our application. This will display the React Query devtools in the browser, allowing us to inspect the status of queries and mutations, view cache details, and analyze performance.
4. Integrating React Query with React Applications 🔄➕
React Query is a versatile library and can be integrated with various other React libraries and frameworks. It seamlessly works with popular state management libraries like Redux and Mobx, allowing us to leverage the best of both worlds. Additionally, it can be used with popular UI libraries like Material-UI and Bootstrap to create visually stunning and responsive user interfaces. Let’s see how to integrate React Query with Redux and Material-UI:
// Integrating React Query with Redux
import { QueryClient, QueryClientProvider } from "react-query"
import { ReactQueryDevtools } from "react-query/devtools"
import { Provider } from "react-redux"
import store from "./store" // Your Redux store configuration
const queryClient = new QueryClient()
const App = () => {
return (
<QueryClientProvider client={queryClient}>
<Provider store={store}>{/* Your application components */}</Provider>
<ReactQueryDevtools />
</QueryClientProvider>
)
}
In this code snippet, we wrap the entire application with both QueryClientProvider
and Provider
from Redux to ensure React Query and Redux work together seamlessly.
// Integrating React Query with Material-UI
import { QueryClient, QueryClientProvider } from "react-query"
import { ReactQueryDevtools } from "react-query/devtools"
import { ThemeProvider, createTheme } from "@mui/material/styles"
import { CssBaseline } from "@mui/material"
import App from "./App" // Your application component
const queryClient = new QueryClient()
const theme = createTheme({
// Your Material-UI theme configuration
})
const Root = () => {
return (
<QueryClientProvider client={queryClient}>
<ThemeProvider theme={theme}>
<CssBaseline />
<App />
</ThemeProvider>
<ReactQueryDevtools />
</QueryClientProvider>
)
}
In this code snippet, we use ThemeProvider
from Material-UI to wrap our application and apply the specified theme. The CssBaseline
component ensures a consistent baseline styling for our app. The Root
component acts as the entry point where we combine React Query, Material-UI, and our main application component (App
).
5. Best Practices for Optimized Data Fetching ✨
To get the most out of React Query, it’s essential to follow some best practices for optimized data fetching. These practices will help maximize performance and create a smoother user experience:
5.1 Use React Query Cache Effectively
Leverage React Query Cache mechanism to its full potential. By implementing caching, you enable efficient retrieval of data, so that future requests for identical information can be swiftly fulfilled from the cache, sparing unnecessary network requests.
5.2 Set Stale Time and React Query Cache Time
Set appropriate values for the staleTime
and cacheTime
options when defining queries. These values determine how long the data remains fresh in the cache and how long React Query keeps the data in the cache, respectively.
5.3 Use Query Prefetching
Prefetch data for upcoming pages or components to reduce perceived loading time and improve user experience. Prefetching ensures that data is readily available when needed.
5.4 Implement Pagination for Large Datasets
When dealing with large datasets, consider implementing pagination to fetch data in smaller chunks. This helps optimize performance and reduces the amount of data fetched at once.
5.5 Optimize Query Dependencies
Carefully manage query dependencies to ensure that queries are updated when the data they depend on changes. Avoid unnecessary react query refetching of data by setting the correct query dependencies.
5.6 Handle React Query Refetch
If required, implement manual react query refetch to provide users with an option to refresh data when needed. This can be useful in scenarios where data updates frequently.
5.7 Use Query Invalidation Wisely
Use query invalidation strategically to ensure that cached data is always up-to-date, especially after performing mutations that affect the data displayed by queries.
5.8 Monitor Performance with Devtools
Keep a close eye on the performance of your queries using React Query’s devtools. This will help identify potential bottlenecks and areas for optimization.
6. Pros and Cons of React Query 👍👎
React Query offers numerous benefits for data fetching and state management in React applications. However, it’s essential to be aware of its strengths and limitations:
6.1 Pros
- Simplifies data fetching: React Query abstracts away the complexities of data fetching, making it easy to fetch, cache, and update data.
- Optimized performance: With caching and intelligent refetching, React Query minimizes redundant API calls and optimizes performance.
- Automatic data synchronization: React Query automatically synchronizes data between the server and the client, ensuring that the UI is always up-to-date.
- Flexible and customizable: React Query provides options to customize caching behavior, retry strategies, and more to fit specific project requirements.
- Seamless integration: It seamlessly integrates with popular libraries like Redux and Material-UI, enhancing their capabilities with advanced data-fetching features.
6.2 Cons
- Learning curve: React Query introduces new concepts and hooks that might have a learning curve for developers new to the library.
- Query duplication: In certain scenarios, multiple components might duplicate the same query, leading to redundant cache entries.
- Browser support: Some features like background updates and automatic retries might have limited support in older browsers.
Despite these limitations, React Query’s benefits significantly outweigh its drawbacks, making it an excellent choice for efficient data management in React applications.
Conclusion 🎉
Great job on reaching the end of this blog post! You’ve made it, and we celebrate your accomplishment! 🎊
You’ve learned how to use some of the advanced features of React Query to optimize your data fetching in React applications. You’ve learned how to manage query dependencies, configure global settings and providers, use advanced devtools and debugging features, integrate React Query with React applications, and follow best practices for optimized data fetching. 🙌
React Query is a powerful and flexible library that can handle any data fetching scenario you can think of. Incorporating caching mechanisms can significantly enhance your application’s speed, user interaction, and programming convenience. 💯
If you want to learn more about React Query, you can check out its official documentation, GitHub repository, and YouTube channel. You can also join its Discord community and follow its Twitter account for updates and news. 🔗
I hope you enjoyed this blog post and found it useful. If you have any questions or feedback, feel free to leave a comment below. 😊
FAQs ❓
1. What is React Query Devtools, and how does it enhance the React Query experience?
React Query Devtools is a browser extension that provides a visual interface to monitor and debug data fetching and caching in React Query. It allows developers to inspect queries, mutations, and react query cache data, and track the network activity of their application, making it easier to optimize data fetching and overall performance.
**2. **What are the recommended best practices for configuring React Query Devtools effectively?****
To configure React Query Devtools effectively, ensure you enable the extension in your browser and use it in conjunction with React Query’s setup. Always update to the latest version of Devtools and React Query for compatibility. Additionally, follow the documentation guidelines to utilize the Devtools’ features for effective debugging and performance improvements.
**3. **What advantages does React Query Devtools offer in optimizing data fetching and API calls?****
React Query Devtools offers several advantages, including real-time visibility into API calls, react query cache management, and query status. It allows developers to inspect and analyze queries, identify redundant or inefficient API calls, and fine-tune query configurations to minimize unnecessary requests, resulting in optimized data fetching and reduced network load.
**4. **Are there any potential downsides or cons to using React Query Devtools in certain scenarios?****
While React Query Devtools is a powerful tool, using it extensively in production might introduce a slight overhead due to the additional data tracking. In such cases, it is advisable to use it primarily during development and testing to avoid any unintended side effects on performance.
5. Does React Query work with server-side rendering (SSR)?
Yes, React Query works well with server-side rendering (SSR). It provides built-in support for server-side data fetching, making it easy to fetch and hydrate data on the server and client.