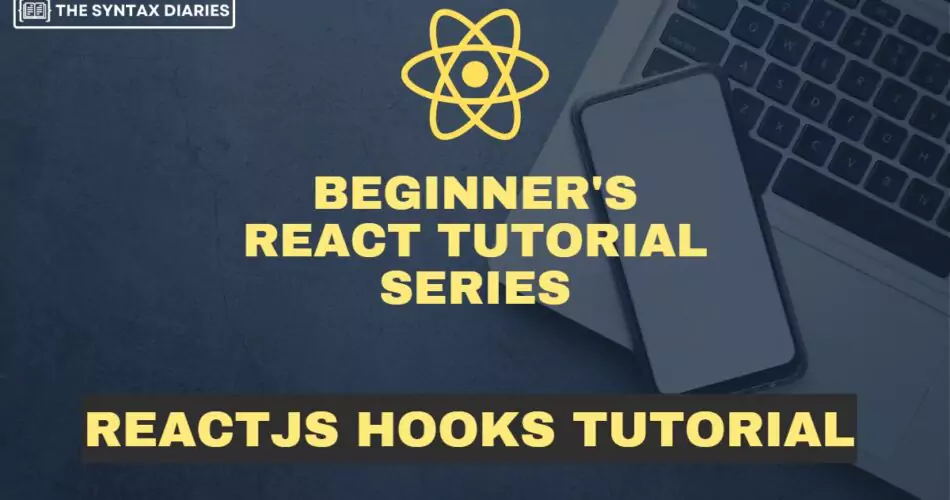
React Hooks Tutorials: Managing State and Side Effects
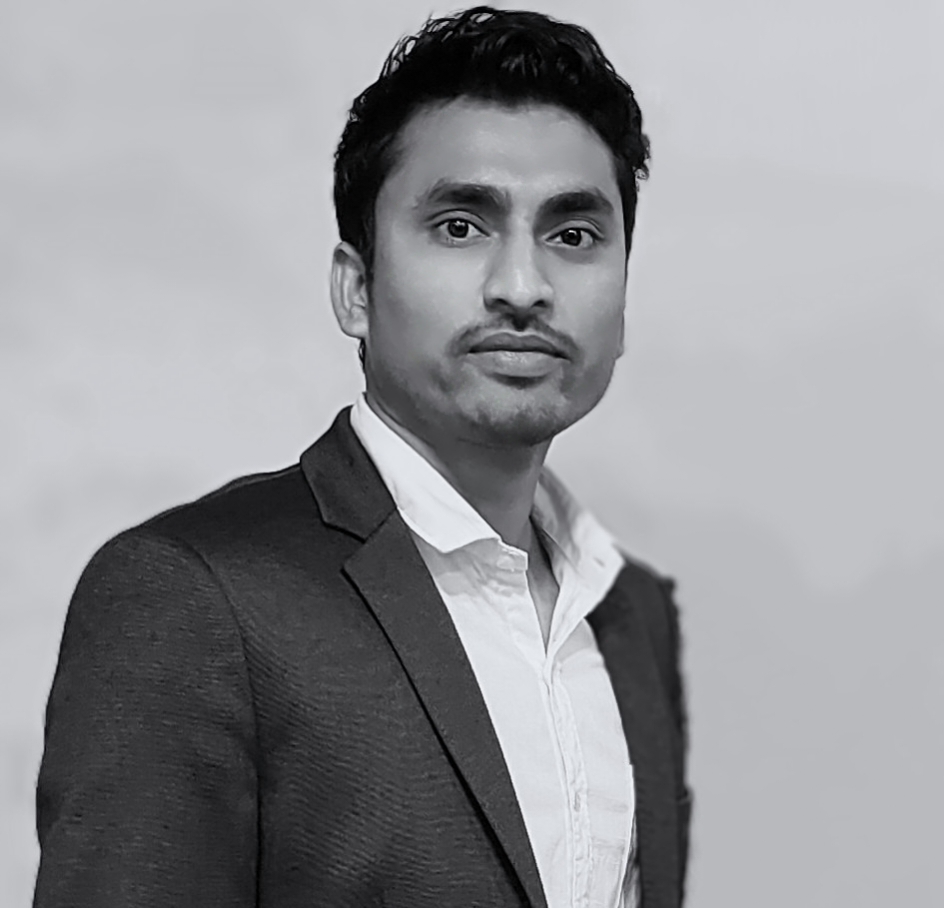
By Amaresh Adak
Welcome to our in-depth guide on React Hooks Tutorial: Managing State and Side Effects! React Hooks revolutionized the way we write React components by providing a simpler and more efficient approach to managing state and handling side effects. With react hooks, we can now write functional components that have access to state and lifecycle methods previously only available in class components. In this react hooks tutorial, we will dive into the world of React Hooks, explore their benefits, learn about the list of hooks in react, and understand how to use them effectively.
If you are interested in learning about components, I recommend checking out my blog post dedicated to this topic. You can read the post by clicking here.
Why React Hooks?
React, a JavaScript library for building user interfaces has gained immense popularity due to its component-based architecture and declarative approach. Traditionally, React class components have been the primary means of building reusable UI components. However, as applications grew in complexity, class components started to exhibit drawbacks, such as cumbersome syntax, code duplication, and difficulties in code reuse.
To address these pain points, React introduced Hooks with the release of React 16.8. Hooks allow developers to write reusable and stateful logic without the need for class components. By utilizing hooks, developers can achieve more concise and readable code, improve component reusability, and enhance the overall development experience.
Understanding State in React
Before we delve into React Hooks, it’s crucial to understand the concept of state in React. State represents the dynamic data within a component, which can change over time due to user interaction, server responses, or other factors. Managing state effectively is essential to building interactive and responsive user interfaces.
In class components, state was managed using the this.state
object, and changes to state were handled with the this.setState()
method. However, with the introduction of hooks, we can now manage state in functional components as well, eliminating the need for class components in many cases.
Introducing React Hooks
React Hooks are functions that allow us to utilize React features, such as state and lifecycle methods, in functional components. They provide a way to “hook into” React’s internal mechanisms and extend the capabilities of functional components. By using hooks, we can extract and reuse stateful logic, making our code more modular and easier to maintain.
The React team established a set of rules for React Hooks in order to ensure consistent and correct usage of Hooks across different components. Developers must always call Hooks at the top level of a component or another custom hook, and they should not conditionally call them or call them within loops.
Below we discuss the list of hooks in react.
useState: Managing State with Hooks
The useState
hook is one of the fundamental hooks provided by React. It enables functional components to have their own internal state, just like class components. With useState
, we can define and update state variables within our functional components.
Getting Start with useState
To begin using useState
, we need to import it from the react
package. We can then call the useState
function, providing an initial value for our state variable. The useState
function provides an array comprising of two elements: the present state value and a corresponding function to modify or update the state.
import React, { useState } from "react"
function Counter() {
const [count, setCount] = useState(0)
// ...
}
In the example above, we define a component called Counter
that utilizes the useState
hook. We initialize the count
state variable to 0 and obtain the setCount
function to update the state. The convention for naming the update function is to prefix the state variable name with “set” followed by the capitalized variable name.
Updating State with useState
To modify the state, we can easily invoke the update function obtained from useState and provide the desired new value as an argument. React will then re-render the component, reflecting the updated state.
function Counter() {
const [count, setCount] = useState(0)
const increment = () => {
setCount(count + 1)
}
// ...
}
In the Counter
component above, we define an increment
function that increases the count
state by 1. By invoking setCount
with the updated value, we trigger a re-render of the component with the new state value.
Working with Complex State
While useState
is suitable for managing simple state variables, it can also handle more complex state objects. We can use objects or arrays as state variables and update them using the spread operator or other immutable update techniques.
function TodoList() {
const [todos, setTodos] = useState([])
const addTodo = (text) => {
const newTodo = { id: Date.now(), text }
setTodos([...todos, newTodo])
}
// ...
}
In the TodoList
component, we initialize the todos
state as an empty array. The addTodo
function adds a new todo to the todos
array by creating a new object with a unique ID and the provided text. We use the spread operator to create a new array that includes all existing todos and the new todo, and then update the state with setTodos
.
Using useState
in this manner allows us to manage more complex state structures within our functional components.
useEffect: Handling Side Effects with React Hooks
In React, side effects refer to actions that occur as a result of component rendering or state changes, such as fetching data from an API, subscribing to events, or manipulating the DOM. Traditionally, side effects were handled in class components using lifecycle methods like componentDidMount
and componentDidUpdate
.
With the useEffect
hook, we can manage side effects in functional components. The useEffect
hook combines the functionality of various lifecycle methods into a single hook, allowing us to handle side effects and specify cleanup logic in a declarative manner.
Understanding Side Effects
Side effects play a crucial role in many applications. For example, when we fetch data from an API, we need to send the request and update the state of the component once we receive the data. Similarly, when subscribing to events, we need to establish the subscription and clean it up when the component unmounts or when certain dependencies change.
The useEffect
hook enables us to perform these side effects in a controlled and predictable way. It ensures that the component executes side effects after rendering and takes care of cleaning them up when necessary.
Getting Start with useEffect
To use the useEffect
hook, we import it from the react
package. We then call useEffect
within our functional component, passing two arguments: a function that represents the side effect and an array of dependencies.
import React, { useEffect } from "react"
function UserProfile({ userId }) {
useEffect(() => {
// Perform side effect here
return () => {
// Clean up side effect here
}
}, [userId])
// ...
}
In the UserProfile
component above, we define a side effect using useEffect
. The side effect function is the first argument passed to useEffect
. Within this function, we can perform any actions necessary, such as making API requests, subscribing to events, or manipulating the DOM.
The second argument to the useEffect
hook is an array of values that the hook will watch for changes. Specifying dependencies allows us to control when to execute the side effect. If any of the dependencies change during renders, the side effect function will re-execute. On the other hand, if we don’t change the dependencies, we will skip the side effect.
Cleaning Up Side Effects
In some cases, side effects require cleanup to prevent memory leaks or invalid states. For example, when subscribing to events, we need to unsubscribe when the component unmounts or when specific dependencies change.
To handle cleanup, we can return a function from the side effect function provided to useEffect
. This cleanup function executes before the next side effect or when the component unmounts.
useEffect(() => {
// Perform side effect here
return () => {
// Clean up side effect here
}
}, [userId])
In the example above, the cleanup function is defined as a return
statement within the side effect function. This enables us to encapsulate the cleanup logic and ensures its execution when needed.
By utilizing the useEffect
hook and specifying cleanup logic, we can safely handle side effects within our functional components.
useContext: Simplifying Context Consumption
Context in React empowers the passing of data through the component tree without the need for manual prop passing at each level. Developers frequently utilize it to share global data, such as user authentication status or theme settings, among multiple components.
In class components, consuming context required using the static contextType
property or the Consumer
component. However, with the useContext
hook, consuming context becomes much simpler and more concise.
Consuming Context with useContext
To consume context using the useContext
hook, we first need to create a context using the React.createContext
function. This function returns a Provider
component and a Consumer
component, but we will focus on the Provider
for this example.
const UserContext = React.createContext()
function App() {
return (
<UserContext.Provider value={{ name: "John", age: 25 }}>
<UserProfile />
</UserContext.Provider>
)
}
In the code snippet above, we create a UserContext
using the React.createContext
function. We then wrap the UserProfile
component with the UserContext.Provider
component and provide a value prop. The value can be any data that we want to share with components consuming this context.
To consume the context within a functional component, we use the useContext
hook and pass the context as an argument.
function UserProfile() {
const user = useContext(UserContext)
return (
<div>
<p>Name: {user.name}</p>
<p>Age: {user.age}</p>
</div>
)
}
In the UserProfile
component, we call useContext
and pass in the UserContext
to consume the context value. We can then access the user object, which was provided by the UserContext.Provider
in the parent component.
Using the useContext
hook simplifies context consumption in functional components, making it easier to access and utilize shared data.
useReducer: A Powerful Alternative to useState
While useState
is suitable for managing simple state variables, more complex state management scenarios may benefit from using the useReducer
hook. useReducer
is an alternative to useState
that provides a way to manage state through a reducer function, similar to how state is managed in Redux.
Creating a Reducer
To utilize the useReducer
hook effectively, it is necessary to begin by defining a reducer function. A reducer represents a pure function that accepts the present state and an action as input parameters, and subsequently produces the updated state as its output.
function reducer(state, action) {
switch (action.type) {
case "INCREMENT":
return { count: state.count + 1 }
case "DECREMENT":
return { count: state.count - 1 }
default:
throw new Error("Unknown action type")
}
}
In the example above, we define a reducer function that handles two actions: INCREMENT
and DECREMENT
. Depending on the action type, the reducer returns a new state object with the updated count value.
Using useReducer
To use useReducer
, we call it within our functional component, providing the reducer function and an initial state value. The useReducer
function provides an array consisting of two elements: the current state value and a dispatch function. This dispatch function allows you to initiate updates to the state.
function Counter() {
const [state, dispatch] = useReducer(reducer, { count: 0 })
const increment = () => {
dispatch({ type: "INCREMENT" })
}
const decrement = () => {
dispatch({ type: "DECREMENT" })
}
return (
<div>
<p>Count: {state.count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
)
}
In the Counter
component, we initialize the state using useReducer
with the reducer
function and an initial state object. We obtain the current state and the dispatch function, which allows us to trigger state updates by dispatching actions.
The increment
and decrement
functions dispatch the corresponding actions to update the state. The component re-renders with the new state, reflecting the updated count value.
useReducer
proves particularly useful when managing complex state updates or when the reducer function needs to handle multiple actions simultaneously.
Optimizing Performance with useMemo and useCallback
In certain scenarios, components may perform expensive computations or calculations that don’t require re-execution on every render. This can lead to decreased performance and unnecessary re-renders.
The useMemo
hook allows us to optimize such computations by caching the result and only re-computing it when the dependencies change. It memoizes the value and returns the cached result if the dependencies remain the same.
Using useMemo
To use useMemo
, we call it within our functional component, providing a function and an array of dependencies. We want to optimize the function that represents the computation, and the dependencies determine when to re-execute the computation.
function Counter() {
const [state, dispatch] = useReducer(reducer, { count: 0 })
const increment = () => {
dispatch({ type: "INCREMENT" })
}
const decrement = () => {
dispatch({ type: "DECREMENT" })
}
return (
<div>
<p>Count: {state.count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
)
}
In the example above, the ExpensiveComponent
receives a data
prop. We use useMemo
to memoize the result of an expensive computation that depends on the data
. The computation function will only be re-executed if the data
prop changes.
By using useMemo
, we can optimize our components and avoid unnecessary calculations on every render.
useCallback: Memoizing Functions
In React, passing new function references to child components can lead to unnecessary re-renders. This can be problematic when dealing with components that rely on referential equality for performance optimizations or when passing functions to pure child components.
The useCallback
hook solves this issue by memoizing functions, ensuring that the same function reference is returned if the dependencies remain unchanged. This allows us to avoid unnecessary re-renders and ensure consistent behavior across renders.
Using useCallback
To use useCallback
, we call it within our functional component, providing a function and an array of dependencies. The function represents the function we want to memoize, and the dependencies determine when the function should be re-created.
function ParentComponent() {
const handleClick = useCallback(() => {
// Handle click event
}, [])
return <ChildComponent onClick={handleClick} />
}
In the example above, the ParentComponent
defines a click handler function, handleClick
, using useCallback
. The function is then passed as a prop to the ChildComponent
.
Since we provide an empty array as the dependencies, the handleClick
function will be memoized and maintain referential equality across renders.
By using useCallback
, we can optimize function references and prevent unnecessary re-renders in our components.
useRef: Preserving Values between Renders
In React, components are typically re-rendered whenever the state or props change. However, there are cases where we want to preserve a value between renders without triggering a re-render.
The useRef
hook allows us to create a mutable ref object that persists across renders. It provides a way to store and access a value that won’t cause re-renders when it changes.
Using useRef
To use useRef
, we call it within our functional component, optionally passing an initial value. The useRef
hook returns a ref object with a current
property that can be used to access and modify the stored value.
useEffect(() => {
intervalRef.current = setInterval(() => {
// Perform timer logic
}, 1000);
return () => {
clearInterval(intervalRef.current);
};
}, []);
// ...
}
In the Timer
component, we create a ref object using useRef
. We store the interval ID in the ref’s current
property within the useEffect
hook. Since the ref value remains unchanged between renders, it does not initiate a re-render when we update the interval ID.
By utilizing useRef
, we can preserve values between renders without causing unnecessary re-renders.
Custom React Hooks: Reusable Logic
React Hooks enable us to create custom hooks, which are functions that encapsulate reusable logic and can share it across multiple components. Custom react hooks enable us to abstract complex logic into reusable units, promoting code reusability and maintainability.
Creating a Custom React Hooks Tutorial
To create a custom hook, we define a function that uses one or more built-in hooks. Custom hooks must start with the word “use” to comply with the Rules of Hooks.
function useCustomHook() {
// Use built-in hooks here
return /* Custom hook logic */
}
In the example above, we define a custom hook called useCustomHook
. Within this function, we can utilize any built-in hooks, such as useState
, useEffect
, or other custom hooks.
The custom hook returns the logic that we want to share across components. It can include state variables, side effects, or any other functionality required by the consuming components.
Using a Custom Hook
Once we have created a custom hook, we can use it within our functional components just like any other hook.
function ComponentA() {
const customData = useCustomHook()
// ...
}
function ComponentB() {
const customData = useCustomHook()
// ...
}
In the example above, both ComponentA
and ComponentB
use the useCustomHook
custom hook. This allows them to share the same logic and state provided by the custom hook.
By creating custom hooks, we can extract and reuse common functionality, making our code more modular and easier to maintain.
React Hooks Tutorial: Managing State and Side Effects – FAQs
1. Q: Can I use React hooks in class components?
A: No, React hooks are designed to be used in functional components. Class components have their own lifecycle methods and state management mechanisms.
2. Q: Are React hooks backward compatible?
A: React hooks were introduced in React 16.8, so they are compatible with versions 16.8 and above. If you are using an earlier version of React, you’ll need to upgrade to use hooks.
3. Q: When should I use useEffect instead of useLayoutEffect?
A: useEffect should be used for most cases. useLayoutEffect is similar to useEffect, but it fires synchronously after all DOM mutations. Use useLayoutEffect only when you need to perform measurements or manipulate the DOM before the browser paints.
4. Q: Are React hooks suitable for large-scale applications?
A: Yes, React hooks can be used in large-scale applications. They provide a more concise and declarative way of managing state and side effects, making the code easier to understand and maintain.
5 Q: Are React hooks a replacement for Redux?
A: Yes, React hooks can be used in large-scale applications. They provide a more concise and declarative way of managing state and side effects, making the code easier to understand and maintain.
Conclusion
React hooks have revolutionized the way we manage state and side effects in functional components. With hooks like useState, useEffect, useContext, useReducer, useMemo, useCallback, and useRef, we have powerful tools at our disposal to create robust and maintainable React applications.
By leveraging hooks, we can simplify our code, improve performance, and enhance reusability. The flexibility and ease of use provided by hooks enable us to build complex applications with cleaner and more concise code.
So, whether you have experience as a seasoned React developer or you’re just starting out, embracing React hooks will help you develop more efficiently and enjoyably.
Now that you have a good understanding of React hooks and their usage, it’s time to dive in and start incorporating them into your own projects. Happy coding!