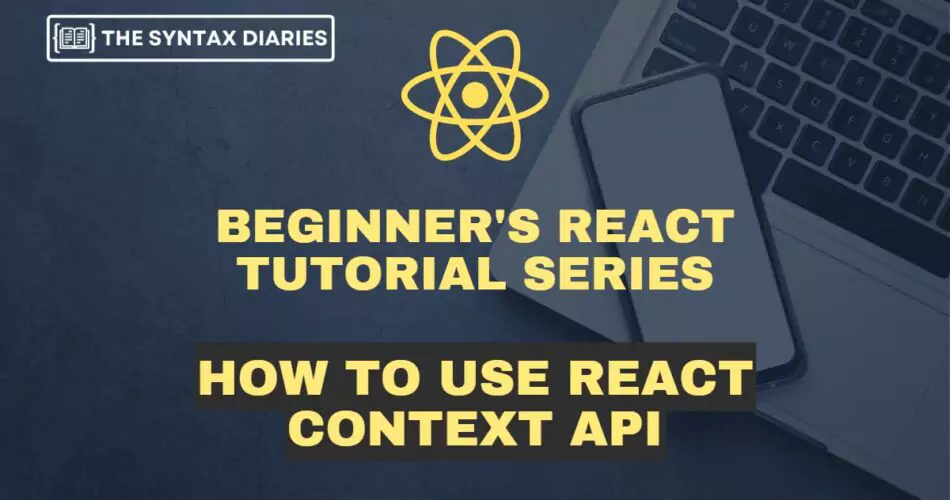
How to Use React Context API – React Context vs Redux
1. Introduction
React Context API is a powerful feature in the React JavaScript library that provides a way to share data between components without the need for explicit props passing. React Context API is a global state management solution that simplifies the process of passing data through the component tree π². In this article, I will explore React Context API, its usage in React applications, and the benefits it offers. We will also delve into the practical aspects of using React Context API, provide examples to illustrate its usage, and discuss best practices as well as common mistakes to avoid. π
2. Benefits of Using React Context API
Context API brings several benefits to the table when it comes to managing state and sharing data in React applications. Letβs explore some of the key advantages: πͺ
2.1 Simplifies Data Sharing Between Components
React Context API simplifies the process of sharing data between components, making it one of its primary advantages. π Instead of passing props through multiple levels of nested components, React Context API enables the creation of a context object that any component within its tree can access. This eliminates the need for prop passing and streamlines the data-sharing mechanism. π³ This eliminates the need to pass props through multiple levels of nested components. This eliminates the need for βprop drilling,β where intermediate components receive and pass down props that are not directly relevant to them. π
2.2 Avoids Prop Drilling
Prop drilling, also known as the βtunnelingβ of props, can lead to code clutter and reduced readability. Context API helps to eliminate prop drilling by providing a centralized mechanism for accessing shared data. With context, you can define the data at a higher level in the component tree and access it directly in any component that needs it, regardless of its depth in the hierarchy. β°οΈ
2.3 Facilitates Global State Management
React Context API is handy for managing the global state of your application. Creating a context at the top level of your component tree allows you to store and update global data that multiple components can access. This allows for a more efficient and organized approach to managing shared state, reducing the need for complex event handling or passing data through intermediate components. π
2.4 Increases Code Readability and Maintainability
By using Context API, you can improve the readability and maintainability of your codebase. Instead of scattering props across multiple components, the context provides a clear and centralized way to access shared data. This makes it easier to understand the flow of data and reduces the cognitive load when working with complex component structures. Additionally, updating the shared data in the context automatically triggers re-renders in the components that consume that context, simplifying the state management process. π
3. How to Use React Context API
3.1 Creating a Context
To use Context API, you need to create a context using the createContext
function provided by React. This function returns a context that allows sharing of data between components. The context comprises two parts: a Provider and a Consumer. The Provider shares the data, while the Consumer accesses that data in the consuming components. π¨
import React, { createContext } from 'react';
// Create a context
const MyContext = createContext();
// Provide the context value to child components
function MyProvider({ children }) {
const sharedData = 'Hello from Context';
return (
<MyContext.Provider value={sharedData}>
{children}
</MyContext.Provider>
);
}
In the example above, we create a context called MyContext
using createContext()
. We then define a MyProvider
component that wraps its child components with the MyContext.Provider
. The value
prop the Provider
specifies the data that will be shared, in this case, the string 'Hello from Context'
. π
3.2 Consuming a Context
Once the context is created and provided, consuming components can access the shared data by using the useContext
hook or the Consumer
component. π
Using the useContext
hook:
import React, { useContext } from 'react';
function MyComponent() {
const sharedData = useContext(MyContext);
return <div>{sharedData}</div>;
}
Using the Consumer
component:
import React from 'react';
function MyComponent() {
return (
<MyContext.Consumer>
{(sharedData) => <div>{sharedData}</div>}
</MyContext.Consumer>
);
}
In both examples, the sharedData
variable receives the value provided by the MyProvider
component. This data can then be rendered or used in any way required by the consuming component. π
3.4 Handling Context Changes
React Context API also provides a mechanism for handling context changes. When the value of a context changes, the components that consume that context are automatically re-rendered to reflect the updated data. π
To handle context changes, you can use the useState
hook or other state management solutions within the provider component. By updating the state, you trigger a re-render of the consuming components. π
import React, { createContext, useState } from 'react';
const MyContext = createContext();
function MyProvider({ children }) {
const [sharedData, setSharedData] = useState('Initial value');
// Function to update shared data
const updateSharedData = (newValue) => {
setSharedData(newValue);
};
return (
<MyContext.Provider value={{ sharedData, updateSharedData }}>
{children}
</MyContext.Provider>
);
}
In the example above, we enhance the previous MyProvider
component to include state management using the useState
hook. We also introduce an updateSharedData
function that allows updating the shared data. π
4. Examples of Using React Context API
4.1 Sharing Global Data
React Context API is well-suited for sharing global data throughout an application. For instance, you can create a context to store user authentication information, such as the current userβs data or their authentication status. This context can then be accessed by various components to display user-specific content or enforce authorization rules. π
import React, { createContext, useState } from 'react';
const UserContext = createContext();
function UserProvider({ children }) {
const [user, setUser] = useState(null);
const login = (userData) => {
setUser(userData);
};
const logout = () => {
setUser(null);
};
return (
<UserContext.Provider value={{ user, login, logout }}>
{children}
</UserContext.Provider>
);
}
In this example, we create a UserContext
to store user-related data. The UserProvider
component manages the user state using the useState
hook and provides the login
and logout
functions to update the user data. Components that need access to the user data can consume UserContext
and utilize the provided functions. πββοΈ
4.2 Managing State
React Context API can also be used to manage state within a specific feature or module of an application. For instance, if you have a complex form with multiple inputs spread across different components, you can use a context to manage the form state and make it accessible to all the form components. π
import React, { createContext, useState } from 'react';
const FormContext = createContext();
function Form
Provider({ children }) {
const [formData, setFormData] = useState({
name: '',
email: '',
message: '',
});
const updateFormData = (field, value) => {
setFormData((prevData) => ({
...prevData,
[field]: value,
}));
};
return (
<FormContext.Provider value={{ formData, updateFormData }}>
{children}
</FormContext.Provider>
);
}
In this example, we create a FormContext
to manage the state of a form. The FormProvider
component uses the useState
hook to store the form data object and provides an updateFormData
function to update specific fields. Components within the form can consume FormContext
and access the form data and update functions. π
4.3 Creating Custom Hooks
You can leverage React Context API to create custom hooks that encapsulate the context logic and provide a simplified interface for consuming components. Custom hooks can abstract away the details of context access and make it easier to consume shared data in various components. π§
import React, { createContext, useContext, useState } from 'react';
const ThemeContext = createContext();
function useTheme() {
return useContext(ThemeContext);
}
function ThemeProvider({ children }) {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme((prevTheme) => (prevTheme === 'light' ? 'dark' : 'light'));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
}
In this example, we create a custom hook called useTheme
that encapsulates the logic of consuming the theme context. The ThemeProvider
component manages the theme state and provides the toggleTheme
function. Components that need to access the theme can use the useTheme
hook to easily consume the theme value and the toggle function. ππ
5. The Difference Between Context API and Redux
Context API and Redux are both state management solutions. In the React ecosystem, they have different scopes and use cases. π
React Context API is a built-in feature of React that allows sharing of data between components. Primarily, React Context API focuses on providing an efficient mechanism for passing data through the component tree. React Context API is suitable for managing local component state. To sharing global data, or creating small-scale state management within specific features. π³
Feature | React Context | Redux |
---|---|---|
Purpose | Sharing data between components | Centralized state management |
Pros | Easy to use, lightweight, integrated into React | Predictable state updates, time-travel debugging, large-scale state management |
Cons | Not suitable for complex state management, not as well-documented as Redux | Large and complex applications with complex state management needs |
When to use | Small to medium-sized applications with simple state management needs | Large and complex applications with complex state management needs |
On the other hand, Redux is a standalone library designed specifically for managing complex application state. It provides a centralized store, reducers, and actions to handle state changes in a predictable and scalable manner. Redux suits large-scale applications with extensive state management needs, where multiple components require access to the same state and involve complex data flow. π
While Context API can handle state management tasks, Redux offers additional features. Redux offers time-travel debugging, middleware support, and ecosystem tools that enhance the developer experience for managing larger-scale application state. π
When choosing between React Context API and Redux, consider the size and complexity of your applicationβs state. React Context API can be a good fit for applications with a simple state structure or applications that require localized state management. If your applicationβs state is large and requires advanced features, such as middleware or time-travel debugging, Redux might be a more appropriate choice. π€
6. Pros and Cons of Using React Context API
Context API offers several advantages, but it also has some limitations and considerations to keep in mind. Letβs explore the pros and cons of using React Context API. β β
6.1. Pros of Using Context API
- Simplifies Data Sharing: Context API eliminates the need for prop drilling and provides a straightforward way to share data between components. π
- Global State Management: It enables efficient management of the global state by allowing multiple components to access and update shared data. π
- Code Readability: Context improves code readability by providing a clear and centralized way to access shared data, reducing complexity. π
- Easy to Use: Context API is relatively easy to grasp and implement, especially for smaller applications or specific use cases. π
6.2. Cons of Using Context API
- Performance Considerations: Context updates can cause unnecessary re-renders if not optimized correctly. Careful consideration should be given to the context tree structure and updating mechanisms. β οΈ
- Lack of Middleware Support: React Context API lacks built-in middleware support compared to Redux, making it less suitable for complex asynchronous operations or advanced data flow manipulation. β
- Limited Devtools and Ecosystem: Redux has a robust set of developer tools and an extensive ecosystem of third-party libraries, whereas React Context API has fewer dedicated tools and community support. β
Considering these pros and cons, React Context API is a powerful tool for managing state and sharing data within React applications, but it might not be the optimal choice for every scenario. Assess the specific needs of your application before deciding on the state management solution to use. π€
7. Best Practices for Using React Context API
To make the most out of React Context API, follow these best practices: π§
- Keep Contexts Focused: Create focused contexts that serve specific purposes. Avoid creating a single global context for all data, as it can lead to unnecessary re-renders and make the codebase harder to maintain. π³
- Memoization and Optimization: Utilize memorization techniques like
useMemo
oruseCallback
to prevent unnecessary re-renders in consuming components when using context values or functions. π§ - Testing and Debugging: Test components that consume contexts thoroughly to ensure correct behavior. Use React DevTools or third-party tools to inspect and debug context values and updates. π
- Consider Alternative Libraries: If your application requires more advanced features like middleware or time-travel debugging, consider using Redux or other dedicated state management libraries that are better suited for complex scenarios. π
By adhering to these best practices, you can ensure that your React Context API implementation is efficient, maintainable, and performs optimally. β
8. Common Mistakes to Avoid when Using React Context API
While using React Context API, be cautious of these common mistakes: β οΈ
- Overusing Context for All State Management: Avoid using context for every piece of state in your application. Overusing context can lead to unnecessary re-renders and make the codebase harder to understand and maintain. Consider using other local state management techniques for non-shared data. β
- Neglecting Performance Considerations: Context updates can trigger re-renders in all-consuming components. Be mindful of the context tree structure and use memoization techniques to prevent unnecessary re-renders. β οΈ
- Misusing Context in Class Components: React Context API is primarily designed for functional components using hooks. While it can be used in class components, be cautious of potential issues related to context updates and lifecycle methods. β
- Not Providing Default Values: When consuming a context, make sure to handle cases where the context value is undefined or not provided. Use default values or fallback logic to prevent errors. β
- Not Keeping Context Updates Isolated: Context updates should be limited to the necessary components. Avoid triggering context updates in unrelated components to maintain code modularity and prevent unintended side effects. β οΈ
By avoiding these common mistakes, you can ensure a smooth and effective implementation of React Context API in your application. π
9. Conclusion
React Context API provides a powerful and efficient solution for managing state and sharing data in React applications. It simplifies data sharing between components, avoids prop drilling, facilitates global state management, and enhances code readability and maintainability. By creating contexts, consuming them, and handling context changes, you can easily manage and share data throughout your application. While React Context API is not a replacement for Redux in complex scenarios, it offers a lightweight and versatile solution for many use cases. By following best practices, avoiding common mistakes, and considering the specific needs of your application, you can leverage the power of Context API to build robust and efficient React applications. π
10. FAQs
1. Is React Context API a replacement for Redux?
No, React Context API is not a direct replacement for Redux. While Context API can handle state management tasks, Redux offers additional features and ecosystem tools that make it more suitable for managing complex application state.
2. Can I use Context API in class components?
Yes, React Context API can be used in class components. However, be cautious of potential issues related to context updates and lifecycle methods. Functional components using hooks are the preferred approach for working with React Context API.
3. How does Context API differ from prop drilling?
Prop drilling involves passing props through multiple levels of nested components to reach a component that needs the data. Context API eliminates the need for prop drilling by providing a centralized mechanism to access shared data without passing it explicitly through intermediate components.
4. Can I have multiple contexts in a React application?
Yes, you can have multiple contexts in a React application. Each context can manage a different piece of data or state. It is recommended to keep contexts focused and specific to their respective purposes.
5. Can I update a context value from any component?
Context values should generally be updated from the component that provides the context (the provider component). Components that consume the context can access and use the data, but they should not directly update the context value. Instead, the provider component should provide functions or mechanisms to update the context value, which will trigger re-renders in the consuming components.