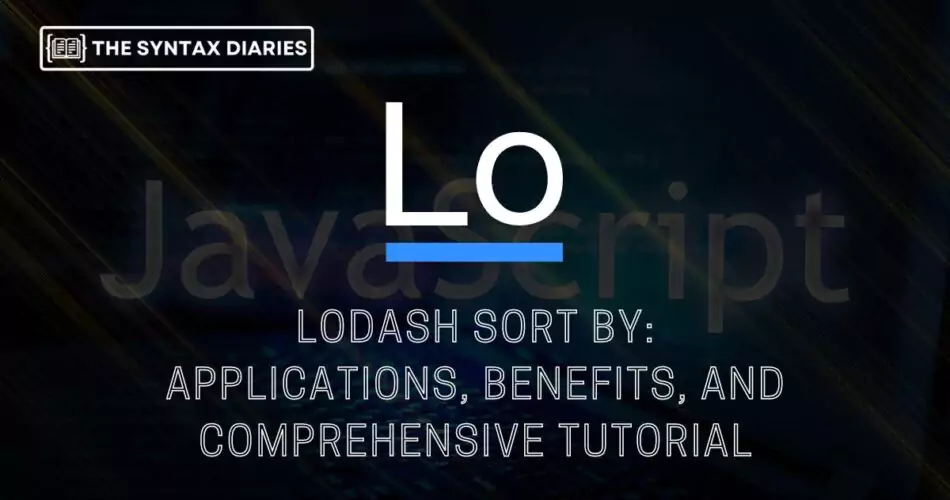
Hi there, welcome to this awesome post on the magic of lodash sort by. If you are a JavaScript lover, you know how important it is to sort data in your apps. Whether you are dealing with arrays or objects, sorting can help you arrange, filter, search, and show your data in a meaningful way.
1. Introduction to lodash sort by
Sorting data is one of the most common and essential tasks in programming. Whether you’re working with arrays, objects, strings, numbers, or any other data type, you’ll often need to arrange them in a certain order to make sense of them, display them, or perform calculations on them.
However, sorting data in JavaScript can be tricky and tedious at times. The native Array.prototype.sort()
method has some limitations and quirks that can lead to unexpected results or errors. For example, it sorts numbers as strings by default, it modifies the original array instead of returning a new one, and it doesn’t handle null or undefined values well.
That’s where lodash
comes in handy. lodash
is a popular JavaScript library that provides a rich set of utilities and functions for working with data in a fast, consistent, and elegant way. One of its most useful and versatile functions is _.sortBy()
, which allows you to sort any collection (array or object) by one or more criteria, using simple or complex logic.
In this article, we’ll show you how to use _.sortBy()
to sort data in various ways, from basic to advanced, and how to overcome some of the common pitfalls and challenges that you might encounter when sorting data in JavaScript.
lodash
2. Installing and Importing Before we can use _.sortBy()
, we need to install and import lodash
in our project. There are several ways to do this, depending on your environment and preferences.
If you’re using Node.js, you can install lodash
as a dependency using npm:
npm install --save lodash
Then you can import it in your JavaScript file using either CommonJS or ES6 syntax:
// CommonJS
const _ = require("lodash")
// ES6
import _ from "lodash"
If you’re using a browser, you can either download lodash
from its official website or use a CDN link to load it from a remote server:
<!-- Downloaded -->
<script src="lodash.js"></script>
<!-- CDN -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.17.21/lodash.min.js"></script>
Either way, you’ll have access to the global _
variable that contains all the lodash
functions.
Alternatively, if you only want to use the _.sortBy()
function and not the entire library, you can install and import it as a standalone module using npm:
npm install --save lodash.sortby
// CommonJS
const sortBy = require("lodash.sortby")
// ES6
import sortBy from "lodash.sortby"
This way, you’ll reduce the size of your bundle and improve your performance.
3. Basic Sorting with lodash sort by
Now that we have lodash
ready to go, let’s see how we can use _.sortBy()
to sort some simple data.
Sorting an array of numbers or strings using lodash sort by is straightforward. You simply pass the array to the function, and it returns a new sorted array:
const numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
const sortedNumbers = _.sortBy(numbers)
4. Sorting Objects by a Single Property
What sets lodash sort by
apart is its ability to sort arrays of objects based on a specific property. Assume you have an array of objects representing books:
const books = [
{ title: "The Great Gatsby", author: "F. Scott Fitzgerald" },
{ title: "To Kill a Mockingbird", author: "Harper Lee" },
// ... other books
]
You can sort these books alphabetically by title using lodash sort by:
const sortedBooks = _.sortBy(books, "title")
5. Sorting with Multiple Properties
In scenarios where you want to sort by multiple properties, lodash sort by
remains your ally. Consider an array of students with both names and ages:
const students = [
{ name: "Alice", age: 25 },
{ name: "Bob", age: 22 },
// ... other students
]
Sorting first by age and then by name can be achieved with a single line of code:
const sortedStudents = _.sortBy(students, ["age", "name"])
6. Custom Sorting Logic using lodash sort by
Sometimes, you need to apply custom sorting logic. For example, sorting numbers by their parity can be accomplished using lodash sort by along with a custom function:
const numbers = [3, 7, 2, 8, 5, 4]
const sortedByParity = _.sortBy(numbers, (num) => num % 2)
7. Sorting Arrays of Strings
When dealing with arrays of strings, you can sort them based on their lengths:
const words = ["apple", "banana", "cherry", "date", "elderberry"]
const sortedWords = _.sortBy(words, "length")
8. Sorting in Descending Order
By default, lodash sort by
sorts in ascending order. To sort in descending order, you can use the _.orderBy
function:
const descendingNumbers = _.orderBy(numbers, [], ["desc"])
9. Sorting with Case Sensitivity
Sorting strings in a case-insensitive manner can be accomplished by converting all strings to lowercase before sorting:
const caseInsensitiveWords = ["Apple", "banana", "cherry", "Date", "elderberry"]
const sortedCaseInsensitive = _.sortBy(caseInsensitiveWords, (word) => word.toLowerCase())
10. Handling Null and Undefined Values
When dealing with null or undefined values, you can ensure they appear at the beginning or end of the sorted array:
const mixedValues = [null, 3, undefined, 1, null, 5, undefined]
const sortedWithNulls = _.sortBy(mixedValues, _.isNil)
11. Sorting Nested Objects using lodash sort by
Sorting nested objects requires a bit more effort. You need to access the nested property using the dot notation:
const people = [
{ name: { first: "Alice", last: "Adams" } },
{ name: { first: "Bob", last: "Brown" } },
// ... other people
]
const sortedByLastName = _.sortBy(people, "name.last")
12. Performance Considerations
While lodash sortBy
is a powerful tool, it’s essential to consider performance when dealing with large datasets. In such cases, using native JavaScript sorting methods might offer better performance.
13. Use Cases of lodash sort by
lodash sort by
finds applications in various scenarios, including e-commerce websites, data visualization projects, and content management systems. Anytime you need to present sorted data to users, lodash sort by
can save you time and effort.
14. Advantages of Using lodash sort by
- Simplicity: Sorting with
lodash sortBy
is concise and easy to implement. - Customizability: You can sort by multiple properties and apply custom sorting logic.
- Flexibility: It works seamlessly with arrays of objects and values.
- Performance: While considering performance, it offers a convenient solution for many sorting needs.
15. Conclusion
The lodash sort by
function provided by lodash is a powerful tool for sorting arrays with flexibility and performance in mind. Whether you’re sorting primitive values, objects, or dealing with advanced sorting scenarios, sortBy
offers a wide array of features to help you achieve the desired order. By understanding its capabilities and applying best practices, you can master the art of sorting arrays efficiently in your projects.
Happy coding! ❤️
Frequently Asked Questions
lodash
a replacement for native JavaScript methods?
1. Is No, lodash
is not a replacement but an extension that provides additional functionalities, including advanced sorting like lodash sort by
.
lodash
only for sorting purposes?
2. Can I use No, lodash
offers a wide range of utility functions beyond sorting, making it a valuable library for various development tasks.
lodash
compatible with modern JavaScript frameworks?
3. Is Yes, lodash
can be used with popular frameworks like React, Vue.js, and Angular to simplify and enhance data manipulation tasks.
4. Are there any performance concerns with using lodash sort by ?
While lodash sort by
is efficient for most use cases, it’s recommended to assess performance with large datasets and consider using native methods if needed.
lodash
compatible with modern JavaScript frameworks?
5. Is While lodash sortBy
is efficient for most use cases, it’s recommended to assess performance with large datasets and consider using native methods if needed.