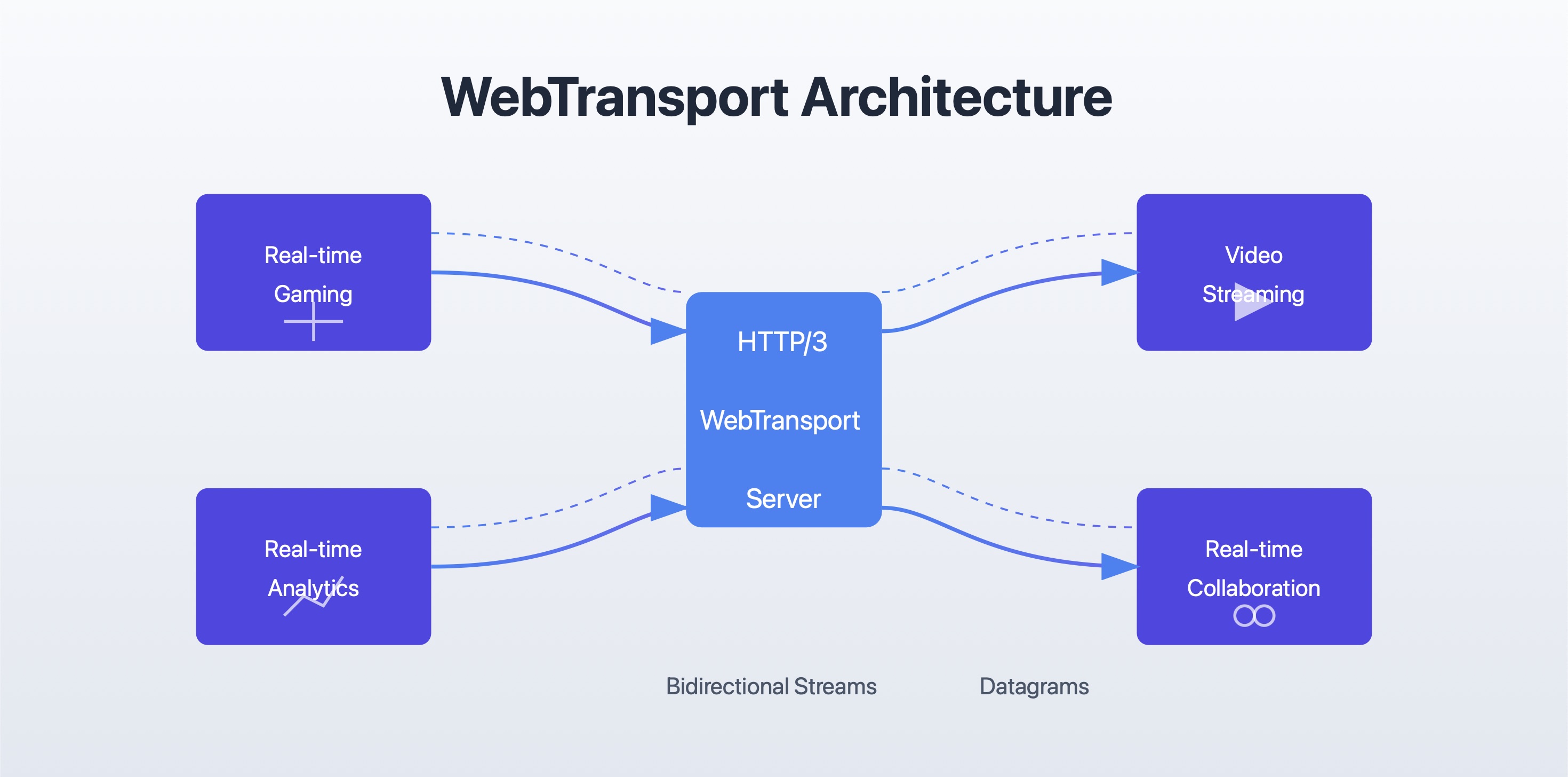
WebTransport Development Guide With Examples
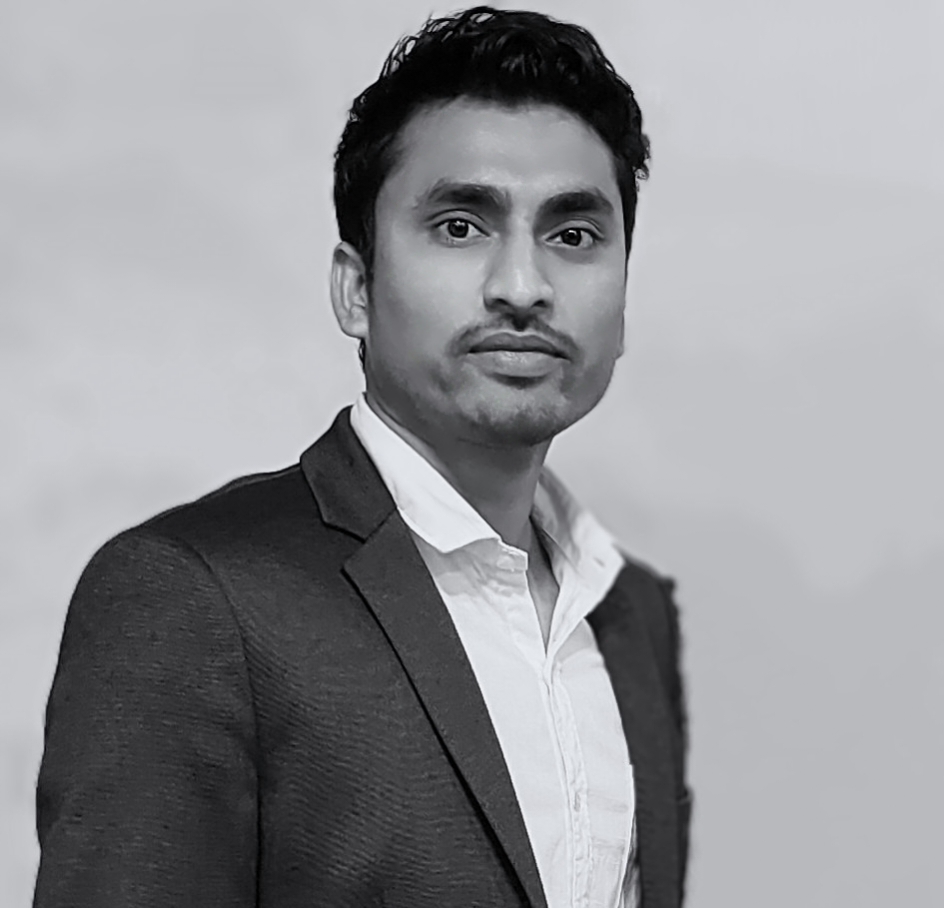
By Amaresh Adak
What is WebTransport?
WebTransport is a cutting-edge web API that revolutionizes real-time communication in web applications. Built on HTTP/3 and QUIC protocols, it provides a powerful alternative to traditional WebSocket and WebRTC technologies, offering enhanced performance and flexibility.
// Basic WebTransport initialization
const transport = new WebTransport('https://example.com/transport');
// Handle connection states
transport.ready.then(() => {
console.log('WebTransport connection established successfully');
}).catch((error) => {
console.error('WebTransport connection failed:', error);
});
Key Features and Benefits
1. Advanced Communication Capabilities
WebTransport provides multiple methods for data transmission, each optimized for different use cases. Bidirectional streams enable reliable, ordered data transfer perfect for critical information, while datagrams offer low-latency communication ideal for real-time updates. This flexibility allows developers to choose the most appropriate method based on their specific needs.
// Example of different communication methods
class TransportHandler {
constructor(url) {
this.transport = new WebTransport(url);
this.streams = new Map();
this.datagramsEnabled = false;
}
// Bidirectional streams
async createBidirectionalStream() {
const stream = await this.transport.createBidirectionalStream();
return {
reader: stream.readable.getReader(),
writer: stream.writable.getWriter()
};
}
// Datagram support
async enableDatagrams() {
this.datagramWriter = this.transport.datagrams.writable.getWriter();
this.datagramReader = this.transport.datagrams.readable.getReader();
this.datagramsEnabled = true;
}
}
2. Technical Advantages
The QUIC protocol foundation of WebTransport delivers significant improvements over traditional protocols. It enables multiplexing multiple streams over a single connection, reduces connection establishment time through 0-RTT handshakes, and provides built-in encryption. These features make it particularly effective for mobile applications and unreliable networks.
// Example of connection migration handling
class ConnectionManager {
constructor(transport) {
this.transport = transport;
this.setupMigrationHandler();
}
setupMigrationHandler() {
this.transport.closed.then(() => {
console.log('Connection closed normally');
}).catch((error) => {
if (error.name === 'NetworkChanged') {
this.handleConnectionMigration();
}
});
}
async handleConnectionMigration() {
// Implement connection migration logic
console.log('Handling connection migration...');
}
}
Implementation Guide
1. Connection Management
Proper connection management is crucial for maintaining stable WebTransport communication. This implementation includes automatic retry logic, connection state monitoring, and error handling. It ensures reliable connectivity even in challenging network conditions and provides clear feedback about connection status.
class WebTransportManager {
constructor(url, options = {}) {
this.url = url;
this.maxRetries = options.maxRetries || 3;
this.retryDelay = options.retryDelay || 1000;
this.transport = null;
this.connectionAttempts = 0;
}
async connect() {
while (this.connectionAttempts < this.maxRetries) {
try {
this.transport = new WebTransport(this.url);
await this.transport.ready;
console.log('Connection established');
this.setupConnectionMonitoring();
return this.transport;
} catch (error) {
this.connectionAttempts++;
console.error(`Connection attempt ${this.connectionAttempts} failed:`, error);
if (this.connectionAttempts < this.maxRetries) {
await new Promise(resolve => setTimeout(resolve, this.retryDelay));
}
}
}
throw new Error('Maximum connection attempts reached');
}
setupConnectionMonitoring() {
this.transport.closed.then(() => {
console.log('Connection closed normally');
}).catch((error) => {
console.error('Connection closed abnormally:', error);
});
}
}
2. Stream Handling
Stream handling in WebTransport requires careful management of both readable and writable streams. This implementation provides a robust system for creating, tracking, and managing multiple concurrent streams. It includes message queuing, error handling, and proper stream lifecycle management to prevent memory leaks.
class StreamHandler {
constructor(transport) {
this.transport = transport;
this.activeStreams = new Map();
}
async createStream(streamId) {
const stream = await this.transport.createBidirectionalStream();
const streamHandler = {
id: streamId,
reader: stream.readable.getReader(),
writer: stream.writable.getWriter(),
messages: []
};
this.activeStreams.set(streamId, streamHandler);
return streamHandler;
}
async sendMessage(streamId, message) {
const stream = this.activeStreams.get(streamId);
if (!stream) {
throw new Error(`Stream ${streamId} not found`);
}
const encoder = new TextEncoder();
await stream.writer.write(encoder.encode(JSON.stringify(message)));
}
async startReading(streamId) {
const stream = this.activeStreams.get(streamId);
if (!stream) {
throw new Error(`Stream ${streamId} not found`);
}
const decoder = new TextDecoder();
while (true) {
const {value, done} = await stream.reader.read();
if (done) break;
const message = JSON.parse(decoder.decode(value));
stream.messages.push(message);
this.handleMessage(streamId, message);
}
}
handleMessage(streamId, message) {
// Implement your message handling logic
console.log(`Received message on stream ${streamId}:`, message);
}
}
3. Datagram Implementation
Datagrams are perfect for real-time applications where speed is more important than guaranteed delivery. This implementation includes sequence numbering for tracking messages and latency measurement. It's particularly useful for gaming, live streaming, and other time-sensitive applications where occasional packet loss is acceptable.
class DatagramHandler {
constructor(transport) {
this.transport = transport;
this.writer = transport.datagrams.writable.getWriter();
this.reader = transport.datagrams.readable.getReader();
this.sequence = 0;
}
async sendDatagram(data) {
const message = {
sequence: this.sequence++,
timestamp: Date.now(),
data: data
};
const encoder = new TextEncoder();
await this.writer.write(encoder.encode(JSON.stringify(message)));
}
async startReceiving() {
const decoder = new TextDecoder();
while (true) {
const {value, done} = await this.reader.read();
if (done) break;
const message = JSON.parse(decoder.decode(value));
this.handleDatagram(message);
}
}
handleDatagram(message) {
const latency = Date.now() - message.timestamp;
console.log(`Received datagram ${message.sequence}, latency: ${latency}ms`);
}
}
Best Practices for Performance
1. Transport Method Selection
Choosing the right transport method is crucial for optimal performance. This implementation provides intelligent selection between streams and datagrams based on message size, reliability requirements, and time sensitivity. The system automatically routes messages through the most appropriate channel based on predefined criteria.
class TransportSelector {
static shouldUseDatagram(data) {
return (
data.isRealTime &&
data.size < 1200 && // MTU consideration
!data.requiresReliableDelivery
);
}
static async send(transport, data) {
if (this.shouldUseDatagram(data)) {
await transport.datagramHandler.sendDatagram(data);
} else {
const stream = await transport.streamHandler.createStream();
await transport.streamHandler.sendMessage(stream.id, data);
}
}
}
2. Performance Optimization
Monitoring and optimizing performance is essential for maintaining high-quality WebTransport connections. This system tracks key metrics like latency, message throughput, and error rates. It provides real-time insights into connection performance and helps identify potential bottlenecks or issues before they impact users.
class PerformanceMonitor {
constructor() {
this.metrics = {
datagramLatencies: [],
streamLatencies: [],
messagesSent: 0,
messagesReceived: 0,
errors: 0
};
}
recordLatency(type, latency) {
this.metrics[`${type}Latencies`].push({
timestamp: Date.now(),
value: latency
});
// Keep only recent measurements
if (this.metrics[`${type}Latencies`].length > 100) {
this.metrics[`${type}Latencies`].shift();
}
}
getAverageLatency(type) {
const latencies = this.metrics[`${type}Latencies`];
return latencies.reduce((sum, item) => sum + item.value, 0) / latencies.length;
}
generateReport() {
return {
avgDatagramLatency: this.getAverageLatency('datagram'),
avgStreamLatency: this.getAverageLatency('stream'),
messageStats: {
sent: this.metrics.messagesSent,
received: this.metrics.messagesReceived,
errors: this.metrics.errors
}
};
}
}
Security Considerations
1. Data Protection
Security is paramount in any web application. This implementation includes message validation, rate limiting, and signature verification to protect against various attacks. It helps prevent common security issues like message tampering, replay attacks, and denial-of-service attempts while maintaining performance.
class SecureTransportHandler {
constructor(transport) {
this.transport = transport;
this.rateLimiter = new RateLimiter(100, 1000); // 100 messages per second
}
async validateMessage(message) {
// Implement message validation
if (!message.signature || !this.verifySignature(message)) {
throw new Error('Invalid message signature');
}
if (!await this.rateLimiter.checkLimit()) {
throw new Error('Rate limit exceeded');
}
}
verifySignature(message) {
// Implement signature verification
return true; // Placeholder
}
}
class RateLimiter {
constructor(limit, window) {
this.limit = limit;
this.window = window;
this.requests = [];
}
async checkLimit() {
const now = Date.now();
this.requests = this.requests.filter(time => now - time < this.window);
if (this.requests.length >= this.limit) {
return false;
}
this.requests.push(now);
return true;
}
}
Real-World Example: Real-Time Game State Synchronization
Here's a complete example of a game state synchronization system:
class GameStateSync {
constructor(url) {
this.transport = new WebTransportManager(url);
this.streamHandler = null;
this.datagramHandler = null;
this.gameState = {
players: new Map(),
worldState: {},
lastUpdate: 0
};
}
async initialize() {
const transport = await this.transport.connect();
this.streamHandler = new StreamHandler(transport);
this.datagramHandler = new DatagramHandler(transport);
// Set up reliable game state stream
const stateStream = await this.streamHandler.createStream('gameState');
this.startStateSync(stateStream.id);
// Start receiving real-time updates
this.datagramHandler.startReceiving();
}
async sendPlayerUpdate(playerState) {
await this.datagramHandler.sendDatagram({
type: 'playerUpdate',
data: playerState,
timestamp: Date.now()
});
}
async startStateSync(streamId) {
// Send initial state request
await this.streamHandler.sendMessage(streamId, {
type: 'stateRequest',
timestamp: Date.now()
});
// Start receiving state updates
this.streamHandler.startReading(streamId);
}
}
// Usage example
const gameSync = new GameStateSync('https://game.example.com/transport');
await gameSync.initialize();
// Send player updates
setInterval(() => {
gameSync.sendPlayerUpdate({
position: { x: 100, y: 200 },
velocity: { x: 5, y: 0 },
action: 'move'
});
}, 50); // 20 times per second
Future Developments
As WebTransport continues to evolve, we can expect:
- Enhanced Integration Features
- Better integration with WebAssembly
- Improved compression algorithms
- Advanced security features
- Extended protocol support
- Performance Improvements
- Reduced latency
- Better bandwidth utilization
- Improved connection stability
- Enhanced error recovery
Conclusion
WebTransport represents the future of real-time web communication, offering a powerful combination of features that make it ideal for modern web applications. By following the implementation patterns and best practices outlined in this guide, developers can create robust, high-performance applications that take full advantage of WebTransport's capabilities.
Remember to:
- Choose the appropriate transport method for your use case
- Implement proper error handling and security measures
- Monitor performance and optimize accordingly
- Stay updated with the latest WebTransport specifications and features