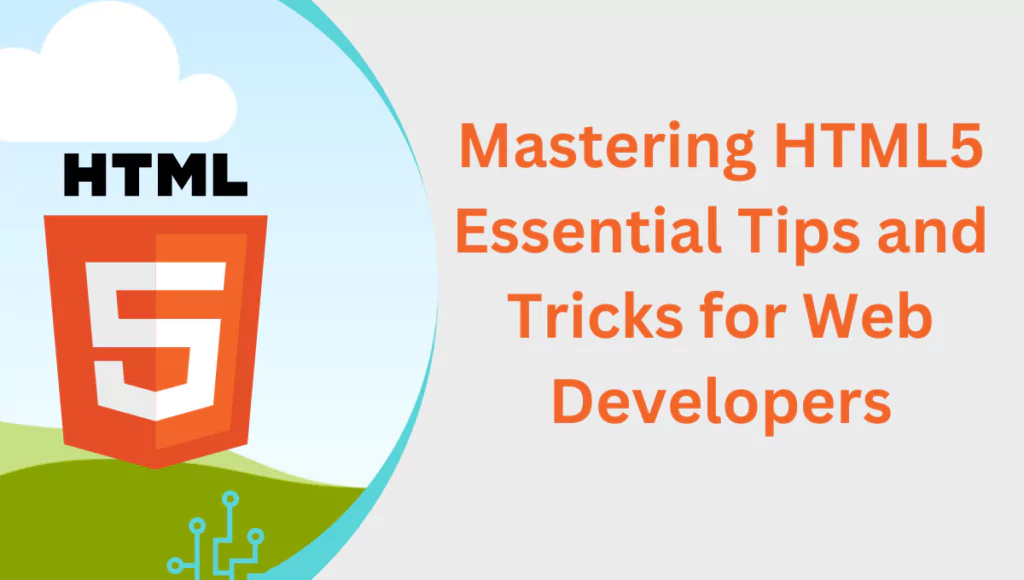
In today’s digital age, HTML5 has become the cornerstone of web development. This blog aims to provide you with essential insights, tips, and tricks to empower you in creating stunning web experiences using HTML5.
Understanding the Basics of HTML5
HTML5 is the fifth and most recent version of the HTML markup language. It is a markup language that is used to create web pages. HTML5 introduces a number of new features that make it easier to create more dynamic and interactive web pages. Some of the new features in HTML5 include:
- Multimedia and graphics: HTML5 allows you to embed audio and video content in your web pages. It also introduces the
canvas
element, which can be used to draw graphics and animations on a web page. - Forms: HTML5 introduces a number of new form elements, such as the
email
input type, which can be used to collect more specific types of user input. - Geolocation: HTML5 allows you to access the user’s geolocation information, which can be used to provide location-based services.
- Drag and drop: HTML5 allows you to add drag and drop functionality to your web pages.
- Offline support: HTML5 allows you to create web pages that can be used offline.
Example:
<audio controls>
<source src="audio.mp3" type="audio/mp3" />
Your browser does not support the audio element.
</audio>
This code will create a simple audio player that will play the audio file audio.mp3
. If the user’s browser does not support the audio element, then a message will be displayed.
<form action="myscript.php">
<input type="text" name="name" />
<input type="submit" value="Submit" />
</form>
This code will create a simple form that will collect the user’s name. When the user submits the form, the value of the name
input will be sent to the myscript.php
script.
HTML5 Semantic Markup
HTML5 Semantic Markup is a way of using HTML tags to describe the meaning of the content on a web page. This makes it easier for search engines to understand the content of a web page, and it also makes it easier for people to read and understand the content of a web page.
For example, instead of using a div
tag to mark up a paragraph of text, you could use a p
tag. The p
tag is a semantic tag that tells the browser that the content inside the tag is a paragraph of text. This makes it easier for search engines to understand the content of the page, and it also makes it easier for people to read and understand the content of the page.
Here are some examples of semantic HTML tags:
article
: This tag is used to mark up an independent piece of content, such as a blog post or news article.aside
: This tag is used to mark up content that is tangential to the main content of the page, such as a sidebar or a comment section.header
: This tag is used to mark up the header of a page.footer
: This tag is used to mark up the footer of a page.nav
: This tag is used to mark up the navigation of a page.
These are just a few examples of the many semantic HTML tags that are available. You can learn more about semantic HTML tags by visiting the official HTML5 website.
Example:
<html>
<head>
<title>My Website</title>
</head>
<body>
<header>
<h1>My Website</h1>
</header>
<main>
<article>
<h2>This is my blog post</h2>
This is the content of my blog post.
</article>
<aside>
<h3>This is my sidebar</h3>
This is the content of my sidebar.
</aside>
</main>
<footer>Copyright © 2023</footer>
</body>
</html>
This code will create a web page with a header, main content area, sidebar, and footer. The header and footer will be marked up with header
and footer
tags, respectively. The main content area will be marked up with a main
tag. The sidebar will be marked up with an aside
tag.
HTML5 Forms and Input Types
HTML5 forms are used to collect user input. They can be used to collect information such as names, addresses, email addresses, and phone numbers. Forms can also be used to collect feedback from users.
There are many different types of input elements that can be used in forms. Some of the most common input elements include:
- Text: This is the most common type of input field. It allows users to enter text.
- Password: This type of input field hides the text that the user enters.
- Checkbox: This type of input field allows users to select multiple options.
- Radio: This type of input field allows users to select only one option.
- Select: This type of input field allows users to select an option from a list.
- File: This type of input field allows users to upload files.
- Date: This type of input field allows users to enter a date.
- Time: This type of input field allows users to enter a time.
- DateTime: This type of input field allows users to enter a date and time.
- Month: This type of input field allows users to enter a month.
- Week: This type of input field allows users to enter a week.
- Number: This type of input field allows users to enter a number.
- Tel: This type of input field allows users to enter a phone number.
- Color: This type of input field allows users to select a color.
- URL: This type of input field allows users to enter a URL.
You can use these input types to create forms that allow users to enter information. The information that users enter in forms can be used to send emails, create accounts, or submit orders.
Here is an example of how you could use an HTML5 form to create a contact form:
Example:
<form action="contact.php">
<input type="text" name="name" placeholder="Your Name" />
<input type="email" name="email" placeholder="Your Email" />
<input type="text" name="message" placeholder="Your Message" />
<input type="submit" value="Submit" />
</form>
This code will create a contact form that allows users to enter their name, email, and message. When the user submits the form, the values of the input fields will be sent to the contact.php
script.
Multimedia and Graphics in HTML5
HTML5 introduced a number of new features for multimedia and graphics, including the video
element, the audio
element, the canvas
element, the picture
element, the source
element, and the track
element.
- The
video
element can be used to embed video content in a web page. For example, the following code will embed a video from YouTube in a web page:
<video width="320" height="240" controls>
<source src="https://www.youtube.com/embed/video_id" type="video/mp4" />
Your browser does not support the video tag.
</video>
- The
audio
element can be used to embed audio content in a web page. For example, the following code will embed an audio file in a web page:
<audio controls>
<source src="audio.mp3" type="audio/mp3" />
Your browser does not support the audio tag.
</audio>
- The
canvas
element can be used to draw graphics and animations on a web page. For example, the following code will draw a simple rectangle on a web page:
<canvas width="200" height="200"></canvas>
- The
picture
element can be used to display multiple images, depending on the user’s device and preferences. For example, the following code will display a different image on mobile devices and desktop computers:
<picture>
<source src="mobile.jpg" media="(max-width: 768px)" />
<source src="desktop.jpg" media="(min-width: 768px)" />
<img
decoding="async"
src="https://thesyntaxdiaries.com/wp-content/themes/maktub/assets/images/transparent.gif"
data-lazy="true"
data-src="default.jpg"
alt="Image"
/>
</picture>
- The
source
element can be used to specify different sources for media files, such as different video formats or different audio codecs. For example, the following code will embed a video that can be played on both Chrome and Firefox:
<video width="320" height="240" controls>
<source src="video.mp4" type="video/mp4" />
<source src="video.webm" type="video/webm" />
Your browser does not support the video tag.
</video>
These are just a few examples of how you can use HTML5 multimedia and graphics features to create more dynamic and interactive web pages.
HTML5 APIs and Advanced Features
HTML5 introduced a number of new APIs and advanced features, including:
- The File API: This API allows you to access files on the user’s computer. For example, you could use the File API to allow users to upload files to your web server.
- The Geolocation API: This API allows you to get the user’s current location. For example, you could use the Geolocation API to show the user’s location on a map.
- The Web Storage API: This API allows you to store data on the user’s browser. For example, you could use the Web Storage API to store the user’s preferences.
- The Drag and Drop API: This API allows you to drag-and-drop elements on a web page. For example, you could use the Drag and Drop API to allow users to rearrange the elements on a page.
- The WebSocket API: This API allows you to create a persistent connection between the browser and the server. For example, you could use the WebSocket API to send real-time updates to the user.
These are just a few examples of the new APIs and advanced features that are available in HTML5.
Example:
Mobile Development with HTML5
HTML5 is a markup language that is used to create web pages. It is also used to create mobile apps. HTML5 mobile apps are web apps that are designed to be used on mobile devices. They are written in HTML, CSS, and JavaScript, and they can be accessed using a web browser.
There are a number of benefits to using HTML5 to develop mobile apps. First, HTML5 is a cross-platform technology. This means that HTML5 apps can be used on a variety of mobile devices, including smartphones, tablets, and phablets. Second, HTML5 apps are easy to develop. HTML, CSS, and JavaScript are all well-known technologies, and there are a number of tools and resources available to help developers create HTML5 apps. Third, HTML5 apps are fast and efficient. HTML5 apps are typically smaller and faster than native apps, which means that they can be downloaded and installed more quickly.
<!doctype html>
<html>
<head>
<title>My App</title>
</head>
<body>
<h1>My App</h1>
This is my first HTML5 mobile app.
</body>
</html>
This code will create a simple web page that can be used as a mobile app. The page has a title and a paragraph of text. The page can be accessed using a web browser on a mobile device.
There are a number of frameworks and tools available to help developers create HTML5 mobile apps. Some of the most popular frameworks include:
- PhoneGap: PhoneGap is a popular framework that allows developers to create HTML5 apps that can be used on a variety of mobile devices.
- Cordova: Cordova is a similar framework to PhoneGap. It is also open source and free to use.
- Ionic: Ionic is a framework that uses HTML5, CSS, and Sass to create mobile apps that look and feel like native apps.
These are just a few of the many frameworks and tools available to help developers create HTML5 mobile apps. With the right tools and resources, it is possible to create high-quality HTML5 mobile apps that can be used on a variety of mobile devices.
Performance Optimization Techniques
Optimizing HTML5 applications is crucial for fast and smooth user experiences. There are a number of techniques that can be used to optimize HTML5 applications, including:
- Minifying and compressing HTML5 code: Minifying removes unnecessary whitespace and comments from HTML5 code, which can significantly reduce its file size. This can lead to faster loading times, especially on mobile devices with slower internet connections.
- Caching: Caching stores copies of frequently accessed files in the browser’s memory. This can help to improve loading times by reducing the number of times that the same files need to be downloaded from the server.
- Optimizing images and multimedia: Images and multimedia can often be a major source of performance problems. To improve the performance of images and multimedia, you can:
- Use smaller image sizes
- Use a format that is supported by the browser
- Optimize the images and multimedia for the web
- Using a content delivery network (CDN): A CDN is a network of servers that are located all over the world. When a user requests a file from a CDN, the file is served from the server that is closest to the user, which can significantly improve loading times.
- Using lazy loading: Lazy loading is a technique that delays the loading of images and other resources until they are actually needed. This can help to improve loading times by reducing the amount of data that needs to be loaded initially.
By following these techniques, you can improve the performance of your HTML5 applications and provide a better user experience.
Cross-Browser Compatibility and Polyfills
HTML5 is a markup language that is used to create web pages. It is a living standard, which means that it is constantly being updated and improved. As a result, not all browsers support all of the features of HTML5. This can lead to cross-browser compatibility problems.
A polyfill is a JavaScript library that is used to add support for missing features in a browser. Polyfills can be used to solve cross-browser compatibility problems.
Here is an example of how a polyfill can be used to solve a cross-browser compatibility problem:
The <video>
element is a new element in HTML5 that is used to embed video content in a web page. Not all browsers support the <video>
element. To solve this problem, you can use a polyfill.
The following code shows how to use a polyfill to add support for the <video>
element in Internet Explorer:
The html5shiv
library is a polyfill that adds support for the <video>
element in Internet Explorer. By adding this code to your web page, you can ensure that the <video>
element will work in all browsers, including Internet Explorer.
There are a number of polyfill libraries available that can be used to add support for missing features in browsers. Some of the most popular polyfill libraries include:
- html5shiv: This library adds support for a variety of HTML5 features, including the
<video>
element, the<audio>
element, and the<canvas>
element. - Modernizr: This library adds support for a variety of HTML5 features and provides information about which features are supported by the current browser.
- Polyfill.io: This website provides a list of polyfill libraries that can be used to add support for missing features in browsers.
By using polyfills, you can ensure that your web pages will work in all browsers, regardless of their capabilities.
Accessibility in HTML5
Web accessibility ensures everyone can use your website, regardless of disabilities. HTML5 offers accessibility features like ARIA attributes and semantic elements. By following best practices, you make your HTML5 websites more inclusive. For example, use ARIA roles and labels to improve screen reader compatibility.
Next Steps in HTML5 Mastery
Mastering HTML5 is an ongoing journey. Explore online tutorials, documentation, and community forums to enhance your skills. Delve into advanced HTML5 topics like Web Components, Web Workers, and WebRTC. Stay updated with the latest HTML5 developments as the web evolves rapidly.
Conclusion
Congratulations on your journey to mastering HTML5! This blog covered the basics of HTML5, including semantic markup, form enhancements, multimedia capabilities, APIs, mobile development, performance optimization, cross-browser compatibility, and accessibility. By applying these tips and tricks, you’ll create beautiful and engaging websites. Embrace HTML5’s power, continue learning, and unlock your creativity to build the next generation of web experiences. Happy coding!